In the world of traditional “centralized” social media, Reddit is an immensely popular users platform. Nonetheless, Reddit is still a centralized platform – and with that comes the risk of censorship or user data exploitation. As such, there are several problematic aspects of today’s centralized social media platforms. However, the Web3 era is now upon us. This paradigm shift will undoubtedly upend various disciplines – potentially even social media – by empowering users with decentralized platforms. With Reddit as an example, could you imagine developing a different version of its platform, such as a Web3 Reddit? In fact, by using Moralis, you can create a Reddit clone for Web3 quickly and easily. As such, we invite you to follow along in this step-by-step guide as we build a Web3 Reddit clone with Moralis.
Thanks to Moralis, the ultimate Web3 development platform, frontend developers can take on all sorts of blockchain projects with JavaScript proficiency alone. With Moralis on your side, you get to create your own NFTs, ERC-20 tokens, launch an NFT marketplace, create a DeFi dashboard, and many other types of dApps (decentralized applications). Moreover, all it takes to cover the entire blockchain-related backend is to copy and paste short snippets of code provided by Moralis’ documentation. As such, you get to overcome all the limitations of RPC nodes and, therefore, focus your attention and resources on creating an impressive and user-friendly frontend.
In this article, we’ll take on the challenge of creating a Reddit Web3 clone, during which you’ll see the power of Moralis’ SDK first-hand. Its Ethereum API, in combination with its IPFS and MetaMask integration and the ultimate Ethereum dApp boilerplate, will enable us to have a fully functional Web3 Reddit dApp ready in a couple of hours.
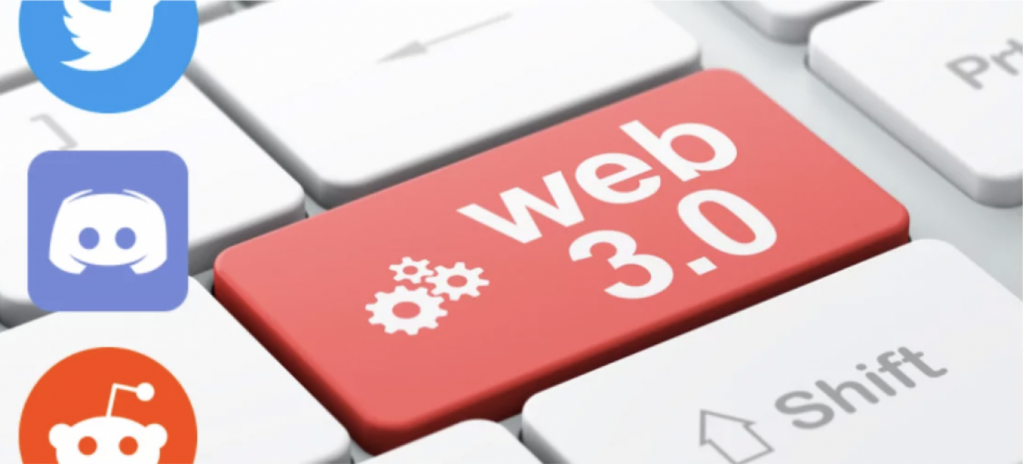
Creating a Web3 Reddit Clone with Moralis
When taking on decentralized projects, such as building a dApp with Moralis, you first need to set things up properly. Fortunately, this setup is straightforward and revolves around the tools we will be using. The latter include the following:
- Moralis – Moralis is the ultimate Web3 development platform that covers all your blockchain-related backend needs.
- MetaMask – MetaMask is the most popular hot wallet that also offers developers the functionality to authenticate their dApp’s users. Essentially, it serves as a gateway into the crypto realm. Moreover, thanks to Moralis’ MetaMask integration, you get to use this wallet seamlessly.
- Code Editor – Despite all the shortcuts provided by Moralis, you still need to do some coding (most often apply simple tweaks to templates). For that purpose, you need a code editor. We normally use Visual Studio Code (VSC); however, feel free to use any other option.
- Ethereum dApp Boilerplate – To make the process of creating a wide range of dApps as simple as possible, we created an Ethereum dApp boilerplate. That way, you can have generic dApps up and running in minutes. Of course, it is then up to your creativity and frontend skills to apply the necessary tweaks and additions to get unique Web3 applications.
When it comes to setting up the tools mentioned, we recommend checking out our article covering the topic of building an Ethereum social network. There you will learn how to initiate the Ethereum dApp boilerplate and create a Moralis server. Furthermore, that article also covers all the details of what our Web3 Reddit clone will look like once finished and what kind of functionalities it will include. The latter include authenticating users (Web3 log in), publishing posts in various categories, and an option to up-vote or down-vote a specific post (see the upcoming section).
Our Web3 Reddit Clone – Quick Preview
Before we take a closer look at each step of this guide, let’s do a quick preview of the Web3 Reddit clone we aim to create. Here’s an image of our finished platform:
The image above shows the name (Decentradit) of our Web3 Reddit clone in the top-left corner, as well as a logged-in user’s address (top-right corner). Furthermore, you can also see that our decentralized Reddit clone incorporates users’ reputations and posts in different categories. Moreover, once a specific category is selected, users get to post by clicking the “Post” button. In addition, each post has the creator’s address on top, followed by a title and then the content itself. Also, as mentioned previously, each post can be up-voted or down-voted, which is the basis for users’ reputation scores. The latter is a great area for applying specific rules that can add more credibility to the platform. For instance, you may add a rule that adds weight to the votes of users with a higher reputation by multiplying them.
Note: To view a detailed demo of the Web3 Reddit clone that we are about to create, check out the video at the end of this article (starting at 0:30). Moreover, you get to see how users can publish posts at 1:36 and how they vote up (or vote down) at 2:14.
A Step-by-Step Guide to Creating a Web3 Reddit Clone with Moralis
Before we actually roll up our sleeves and start tweaking and adding code to create a Web3 Reddit clone as presented above, let’s do a brief overview of the steps that we need to complete:
- Create your free Moralis account, which will enable you to create a Moralis server.
- Make use of the ultimate Ethereum dApp boilerplate (quick start) by using a code editor (such as VSC).
- Create a smart contract that will ensure all of the transactions related to posting and voting inside our Web3 Reddit platform will be properly executed.
- Set up event listeners for our Moralis server.
- Apply the necessary tweaks and additions to the Ethereum dApp boilerplate to get the frontend that we’re looking for (as presented in the preview above).
- Add the proper code to cover the functionality of our Reddit clone. This includes the use of IPFS integration to store posts as content in a decentralized manner. Moreover, connect the created smart contract properly so that the functionalities of adding posts, voting, and updating the post feed and users’ reputation will be executed as planned.
As mentioned above, we have a separate article covering the details of setting things up properly to create a Web3 Reddit clone (steps one and two). As such, we will devote our attention to steps three to six herein. Moreover, for easier understanding of certain steps, we will be referencing specific timestamps in the video, in which a Moralis expert takes on all of the steps listed above.
Add Your Necessary Smart Contract
The smart contract that we’ve created for this purpose is at your disposal at GitHub (“decentradit.sol”). As explained above, our Web3 Reddit platform will implement posting and voting functionalities, which will be guided by the use of the “decentradit.sol” smart contract. Moreover, let’s take a closer look at some particular parts of this smart contract. If you prefer to watch video explanations, check out the video below at 3:13.
The following four events are the core of our smart contract: creating a post, adding content, creating a new category, and voting (upvoting or downvoting).
contract Decentradit { event PostCreated (bytes32 indexed postId, address indexed postOwner, bytes32 indexed parentId, bytes32 contentId, bytes32 categoryId); event ContentAdded (bytes32 indexed contentId, string contentUri); event CategoryCreated (bytes32 indexed categoryId, string category); event Voted (bytes32 indexed postId, address indexed postOwner, address indexed voter, uint80 reputationPostOwner, uint80 reputationVoter, int40 postVotes, bool up, uint8 reputationAmount);
Proper “mapping” must be set in place to ensure that the key events above are triggered properly and follow predefined rules. As such, we need to map a category registry, content registry, post registry, vote registry, and reputation registry for our Reddit clone:
mapping (address => mapping (bytes32 => uint80)) reputationRegistry; mapping (bytes32 => string) categoryRegistry; mapping (bytes32 => string) contentRegistry; mapping (bytes32 => post) postRegistry; mapping (address => mapping (bytes32 => bool)) voteRegistry;
For each of the four events above, we have at least one function that automates the proper functionality. In total, our smart contract has the following nine functions:
- “createPost” – Provides guidelines for creating posts.
- “voteUp” – Provides guidelines for upvoting.
- “voteDown” – Provides guidelines for downvoting.
- “validateReputationChange” – Ensure proper users’ reputation is tracked.
- “addCategory” – Provides guidelines for adding categories.
- “getContent” – Get content from the content registry.
- “getCategory” – Get categories from the category registry.
- “getReputation” – Get reputation from the reputation registry.
- “getPost” – Get posts from the post registry.
Note: To activate your smart contract, you have to deploy it.
Set Event Listeners
If we want our smart contract to execute the required function in the proper situation, it needs to be able to “listen” to what is going on with our Web3 Reddit platform. For that purpose, we need to set proper event listeners in place. This is another area where we get to rely on Moralis to make things a lot simpler for us. To set event listeners in place, complete these steps (watch video at 14:08 – 21:35):
- Log in to your Moralis account and navigate to your admin area.
- Go to the “Servers” section of your Moralis admin area and click “View Details”. At this point, you should have your server ready (if not, use the instructions provided in the Ethereum social network article).
- After the pop-up window containing your Moralis server details appears, click the “Sync” tab in the top-right corner.
- Then click the “Add New Sync” and “Sync and Watch Contract Events”:
- Select a chain, add the description, topic, ABI, address, and “TableName” for each of your events. Here are all the sub-steps for our smart contract’s “CategoryCreated” event:
- Select a chain by clicking on the chain you selected for your server when you were creating it:
- Add a description; we use “Categories” for this event listener:
- Add a topic; for our “category created” event, we use the following:
- Add the ABI, which you copy from the “eventsAbi.json” file:
- Insert your smart contract’s address (which you’ve deployed in the previous step):
- Add a table name; we use “Categories” for this event listener and complete this listener by clicking the “Confirm” button:
Now, repeat step five (and all of the above sub-steps) for other events in our smart contract:
This means that you need to have a total of four listeners (categories, contents, posts, and votes) before moving on.
Apply Tweaks to the Ethereum dApp Boilerplate
By completing step two (as presented in the “A Step-by-Step Guide to Creating a Web3 Reddit Clone with Moralis” section), you should have your boilerplate ready and our template dApp active. For the purpose of creating a Web3 Reddit clone, we need to apply the necessary tweaks. The simplest way for you to apply these changes is to follow a Moralis expert in the video below starting at 25:35. Next, you can follow the Moralis expert’s lead to use Bootstrap and avoid CSS coding.
Moving forward, you’ll be guided to delete certain components (inside the “src” folder), including “Chains”, “Contract”, “ERC20Transfers”, “InchDex”, “NativeTransactions”, and “Wallet”. You will proceed with deleting “ERC20Balances.jsx”, “NativeBalance.jsx”, “NFTBalance.jsx”, “Ramper.jsx”, “QuickStart.jsx”, and “TokenPrice.jsx”.
Next, you will clean the “App.jsx” file (28:38), starting by deleting several “import” lines.
Next, you will be deleting <Menu /> and <Chains />. Then, you will be cleaning <Switch> by eliminating all routes aside from the “nonauthenticated” route. Moving forward, you’ll be deleting numerous footers that you won’t need for our Web3 Reddit dApp. Moreover, you’ll also remove the logo. To wrap the clean-up, you will be instructed to add a particular add-on (“ES7 React/Redux/GraphQL/React-Native snippets”) to VSC. Next, you’ll get to create a new component, “Main.jsx” (32:20), and add the proper “import” line. After applying all these tweaks, you will have our Reddit clone dApp in its initial phase:
This is what a user will see after logging in:
The current state of our Web3 Reddit clone is a clean slate, which we must upgrade by adding proper code.
Creating a Web3 Reddit Clone with Moralis – Adding Code
This is the programming part where you’ll start adding the necessary code to create the Web3 Reddit clone that we’re after. To cover this part of our quest, we are entrusting you with the Moralis expert in the video below at 36:03. He will show you how to program several files (components), starting with “Main.jsx”. Moving forward, you’ll also add full frontend and backend functionality to your dApp. The most vital part of our Web3 Reddit clone will be connecting the application with the smart contract created previously (1:04:25). Furthermore, to obtain the highest level of decentralization, you’ll learn how to make use of Moralis’ IPFS integration, which will enable you to store your Reddit clone’s posts as content in a decentralized manner.
Finally, here’s the video that we’ve been referencing throughout the article:
Create a Reddit Clone for Web3 – Step-by-Step Guide – Summary
We hope that you’ve successfully followed all of the steps covered in the sections throughout this article and that you now have your own version of a Web3 Reddit clone available. All in all, there were six steps you needed to complete to successfully finish this example project:
- Create your free Moralis account.
- Use the ultimate Ethereum dApp boilerplate.
- Create a proper smart contract and deploy it.
- Set up event listeners for your Moralis server.
- Apply the necessary tweaks and additions to the Ethereum dApp boilerplate.
- Add proper code to cover the full functionality of your Reddit clone.
Moreover, please note that this is a slightly more advanced project. Therefore, it took us about two hours to cover all the aspects. However, in our opinion, it is still pretty impressive that we were able to create a Web3 Reddit clone in such a short amount of time. If you are interested in taking on other simpler and quicker example projects, make sure to visit Moralis’ YouTube channel and Moralis’ blog. Some of our latest quests will show you how to lazy mint NFTs, how to create an Ethereum dApp instantly, and how to generate thousands of NFTs. Furthermore, in addition to creating Web3 applications, we also encourage you to focus on mobile dApps, which you can create easily by using our Ethereum mobile boilerplate.