Are you looking for the quickest and easiest way to get the price of any Ethereum token? If so, the Token API from Moralis is a no-brainer. With this application programming interface, you can query any Ethereum token price with a single call to the getTokenPrice
endpoint:
const response = await Moralis.EvmApi.token.getTokenPrice({ address, chain, });
Calling this endpoint returns a JSON response with parsed data looking something like this:
{ "nativePrice": { "value": "13753134139373781549", "decimals": 18, "name": "Ether", "symbol": "ETH" }, "usdPrice": 16115.165641767926, "exchangeAddress": "0x1f98431c8ad98523631ae4a59f267346ea31f984", "exchangeName": "Uniswap v3" }
By familiarizing yourself with the getTokenPrice
endpoint and Moralis in general, you can effortlessly build decentralized applications (dapps) and other Web3 platforms in no time. Signing up is free, and you gain immediate access to the enterprise-grade Web3 APIs from Moralis, enabling you to completely leverage the power of blockchain technology!
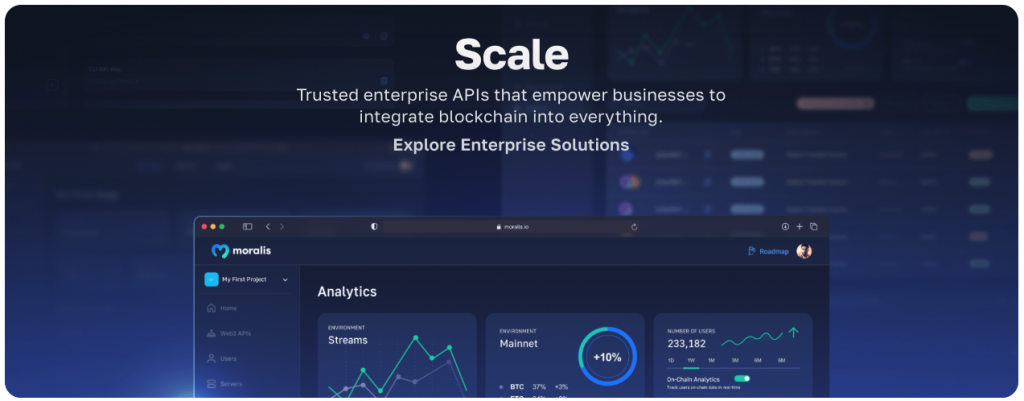
Overview
In today’s article, we will show you how to get any ERC-20 Ethereum token price in three straightforward steps:
- Setting up your project and Moralis
- Calling the Moralis
getTokenPrice
endpoint - Running the script
By completing the aforementioned steps, you will learn how to query the price of any Ethereum token in just a few minutes! If this sounds exciting and you are eager to get going, click here to jump straight into the tutorial.
After the third step, we also show you how to implement this functionality into a NextJS and NodeJS application. This section features a YouTube video where one of our talented software engineers shows you how to build an app allowing users to continuously query the price of any Ethereum token. Following this, we also take a section to explore Moralis further, as this is the easiest way to continue building in Web3.
Lastly, for the uninitiated, the final part of this article is dedicated to exploring the ins and outs of ERC-20 tokens. As such, if you are new to the space and are unfamiliar with these tokens, we recommend starting with the ”What is an Ethereum ERC-20 Token?” section.
Now, this tutorial is relatively simple, as it only takes a few lines of code to get any Ethereum token price when working with Moralis. That said, if you are looking to build more advanced projects, we recommend checking out our guides on how to build a cryptocurrency portfolio dashboard and diving into Web3 marketplace development!
Also, remember to sign up with Moralis before continuing, as you need an account to make Web3 API calls to Moralis’ various endpoints!
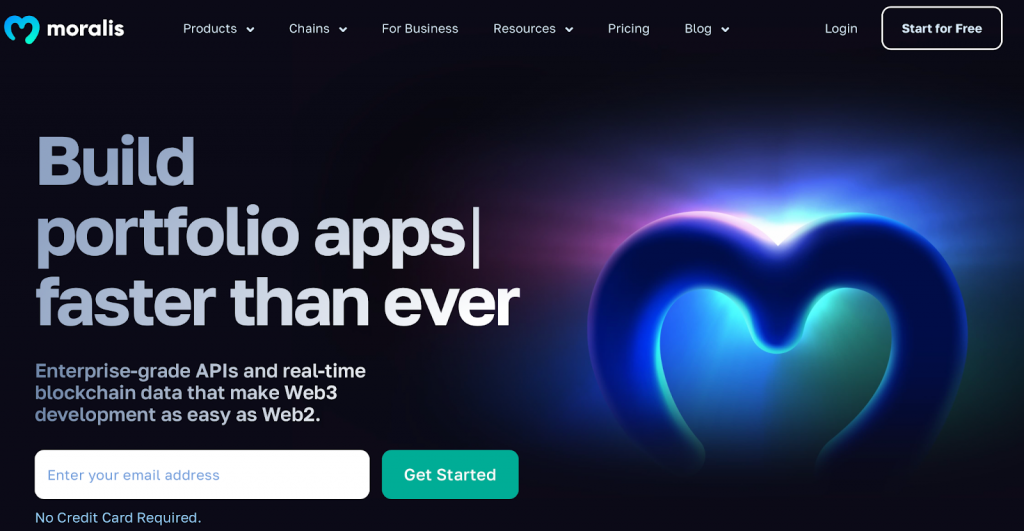
Get Any Ethereum Token Price in 3 Steps
In the following sections, we will show you how to get any Ethereum token price in three steps using the best Ethereum price API. For this tutorial, we will be using JavaScript. However, you can also, for instance, use Python or Go. If you prefer either of these languages, go to the get ERC-20 token price documentation page, where you can toggle between various options to see how the getTokenPrice
endpoint works:
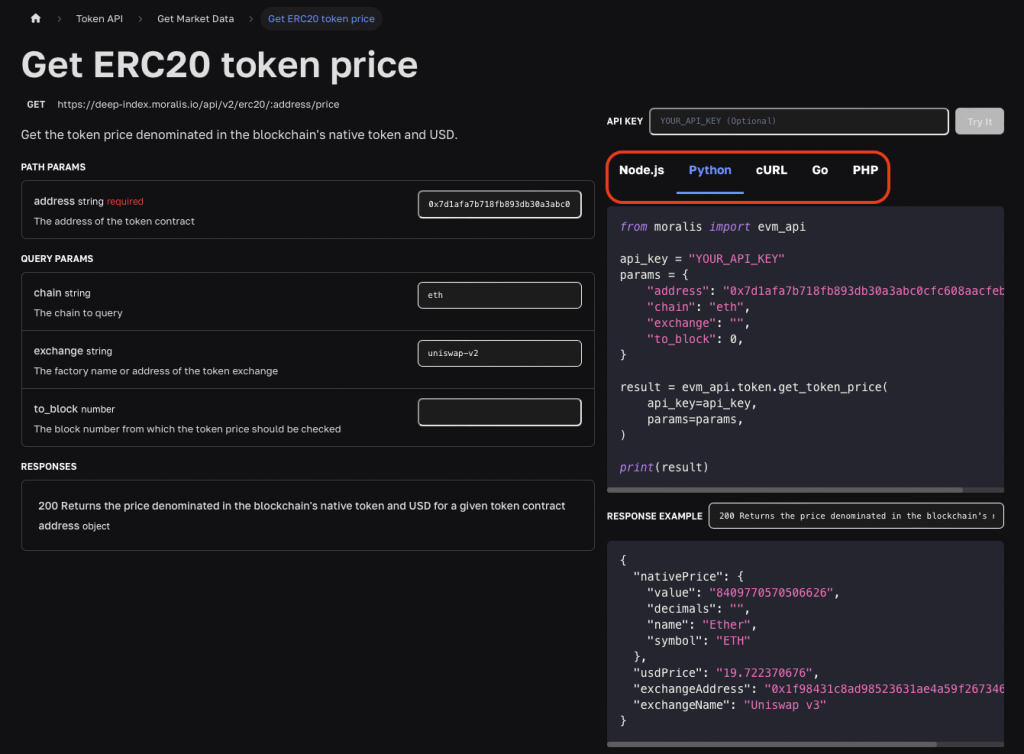
Otherwise, if you want to use JavaScript and NodeJS, join us below as we show you how to get the price of any Ethereum token in no time. But before jumping straight into the first step, we must cover a few prerequisites!
Prerequisites
Before we get going with the tutorial on how to get any Ethereum token price, you need to have the following ready:
- Node v.14+
- The npm or yarn package manager
Step 1: Setting Up Your Project and Moralis
With the required prerequisites taken care of, it is time to kickstart this tutorial on how to get any Ethereum token price. To begin with, create a new project folder and open it in your preferred integrated development environment (IDE).
In this tutorial, we will use Visual Studio Code (VSC); however, you are free to use any IDE of your choice. Just note that if you opt for another alternative, the process might differ slightly on occasion.
With a project folder at your disposal, create a new ”index.js” file. This file will contain your script, but more on that in the second step. Next, you must also install the Moralis SDK. To do so, open a new terminal by clicking on ”Terminal” at the top of VSC, followed by ”New Terminal”:
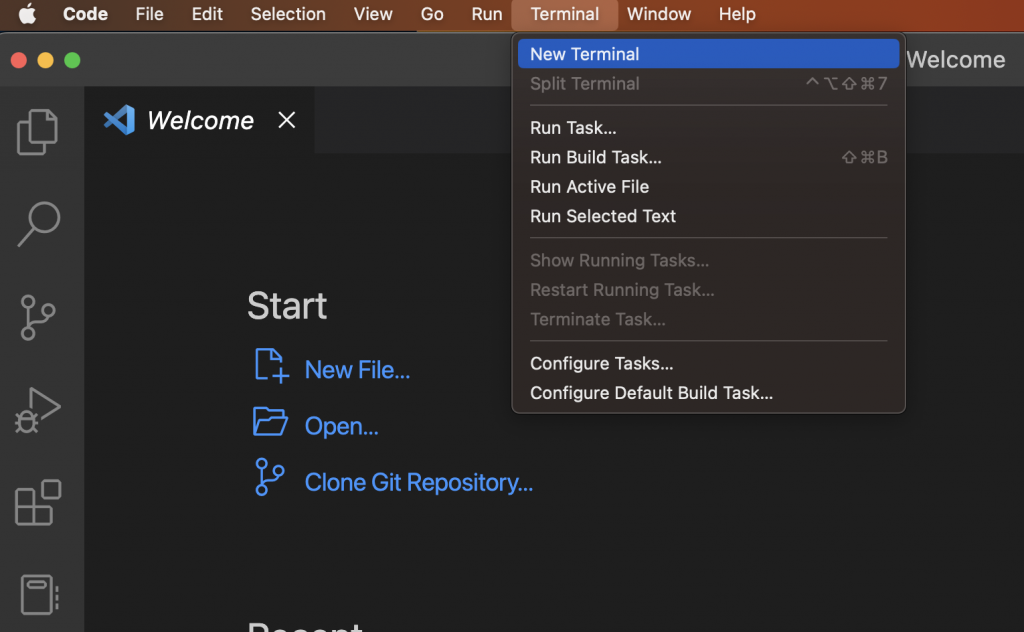
You can then cd
into the project folder and install the SDK by running the following command:
npm install moralis @moralisweb3/common-evm-utils
That’s it for the project setup. From here, you need to set up a Moralis account. So, if you have not already, register with Moralis right now. You can create an account for free, which only takes a few seconds!
With an account, log in to the Moralis admin panel and navigate to the ”Web3 APIs” tab. This is where you find your Moralis API key, which you can go ahead and copy as you need it in the next step:
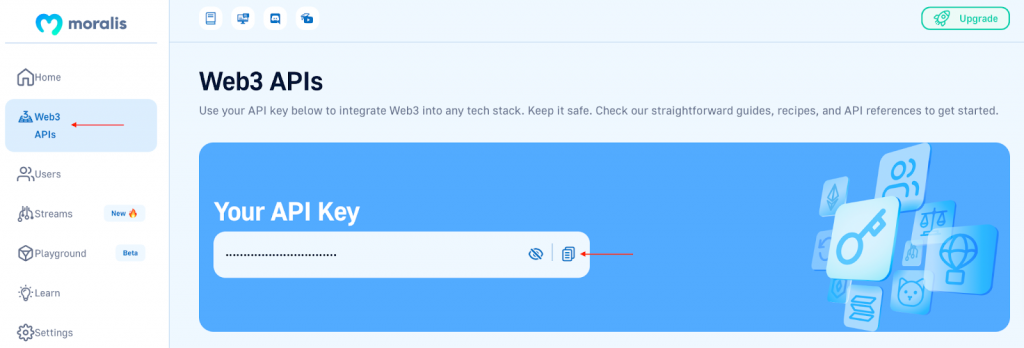
Step 2: Calling the Moralis getTokenPrice
Endpoint
For the second step, you must create the script for calling the getTokenPrice
endpoint. To do so, open your ”index.js” file and insert the following code snippet:
const Moralis = require('moralis').default; const { EvmChain } = require('@moralisweb3/common-evm-utils'); const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const address = 'replace_me'; const chain = replace_me; const response = await Moralis.EvmApi.token.getTokenPrice({ address, chain, }); console.log(response.toJSON()); } runApp();
To make the code operational, you must add three parameters: your Moralis API key, a token address, and a blockchain network. So, to begin with, replace YOUR_API_KEY
with the key you copied in the first step:
const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration });
Next, since you are looking to query the price of a particular token, you need to add its address and chain. As such, go ahead and replace replace_me
for both of these variables:
const address = 'replace_me'; const chain = replace_me;
From there, as you can see if you inspect the code, we utilize these parameters when calling the getTokenPrice
endpoint:
const response = await Moralis.EvmApi.token.getTokenPrice({ address, chain, });
To give you an idea of what it might look like in practice, here is an example where we have added the three parameters:
const Moralis = require('moralis').default; const { EvmChain } = require('@moralisweb3/common-evm-utils'); const runApp = async () => { await Moralis.start({ apiKey: "JnJn0MWxF…", // ...and any other configuration }); const address = '0x2260fac5e5542a773aa44fbcfedf7c193bc2c599'; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.token.getTokenPrice({ address, chain, }); console.log(response.toJSON()); } runApp();
Once you are done configuring the code, all that remains is running this script to get any Ethereum ERC-20 token price!
Step 3: Running the Script
Running the script is easy. All you need to do is open a new terminal, cd
into the project folder, and run the following command:
node index.js
In return, you should find a JSON response similar to the one shown down below in your terminal:
{ "nativePrice": { "value": "13753134139373781549", "decimals": 18, "name": "Ether", "symbol": "ETH" }, "usdPrice": 16115.165641767926, "exchangeAddress": "0x1f98431c8ad98523631ae4a59f267346ea31f984", "exchangeName": "Uniswap v3" }
As you can see in the snippet above, you get parsed data appertaining to the token address you queried. This includes the name, token symbol, USD price, etc., all values you can use directly in your Web3 projects!
That’s it; congratulations! You now know how to get any Ethereum token price with a few lines of code using the Moralis Token API!
Going Beyond – Create an App to Get Any Ethereum Token Price
Now that you know how to get the price of any Ethereum token by making a single call to the Moralis getTokenPrice
endpoint, you might wonder how to implement this functionality into your projects. If this is your ambition and you want to know more about this, we recommend watching the YouTube video below.
In this clip, one of our software engineers shows you how to create a NextJS and NodeJS application incorporating the functionality to get any Ethereum token price. The application has two input fields for the token address and chain variables. As such, this is a more dynamic implementation of the getTokenPrice
endpoint where users can continuously query the price of any Ethereum token by specifying these parameters. If this sounds exciting, you can find the video here:
Continue Building with Moralis Web3 APIs
Throughout this tutorial and the video above, you have been able to familiarize yourself with the Moralis Token API. However, if you are serious about becoming a blockchain developer, you might also want to check out additional blockchain development resources offered by Moralis!
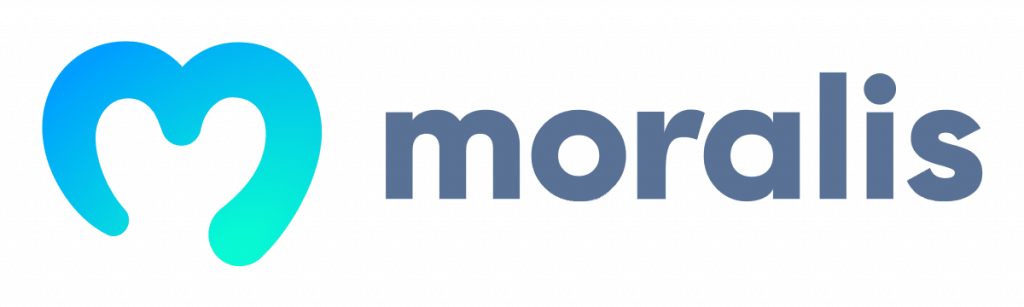
The price of tokens is only one piece of data that you, as a Web3 developer, might be interested in. Fortunately, no matter what blockchain data you are after, Moralis has you covered! Along with the Web3 Data API, Moralis also offers prominent interfaces like the Web3 Streams API and Authentication API. Furthermore, with the Moralis Web3 Streams API, you can seamlessly stream on-chain data into the backend of all your Web3 projects via Web3 webhooks. Also, using this API, you can effortlessly get real-time user asset transfers, notify users autonomously about Web3 events, and track any smart contract events based on your filters!
With the Authentication API, you get a unified interface for all Web3 authentication methods, a comprehensive SDK for easy implementation, and compatibility with auth aggregators. As such, setting up a Web3 authentication flow has never been easier!
You should also be aware of Moralis’ cross-capabilities, supporting all major blockchain networks. This includes Ethereum, BNB Chain, Polygon, Arbitrum, Aptos, Avalanche, and many others. As such, when working with Moralis, you do not limit yourself to one ecosystem. This means you can easily port your project across networks with only minor configurations.
So, if you are looking to build more sophisticated Web3 projects, do like other industry leaders such as MetaMask, Delta, NFTrade, etc., and use Moralis to build and scale your platforms!
What is an Ethereum ERC-20 Token?
If you are new to blockchain development, chances are that terms such as ”ERC-20” or ”ERC-721” go over your head. As this might be the case, we will take this section to explore the intricacies of Ethereum ERC-20 tokens. So, without further ado, let us answer the question, ”what is an ERC-20 token?”.
Tokens within the Ethereum ecosystem can represent virtually anything. Some great examples are skill points in a game, fiat currency, lottery tickets, an amount of gold, etc. With this utility, it is essential that tokens on the Ethereum network are handled by a robust standard, which is where ERC-20 enters the equation. That said, what exactly is the ERC-20 token standard?
ERC-20 is the most popular token standard, and it is used to regulate so-called ”fungible tokens”. The term ”fungible” describes interchangeable assets, meaning that fungible tokens of a particular type are exactly the same. This further means they share the same type and value, which is why one can be swapped for another without monetary compensation. For instance, a prominent example is Ethereum’s native currency ETH.
ERC-20 is an abbreviation for ”Ethereum Request for Comments 20”, and this standard was introduced in 2015 by Fabian Vogelsteller. This token standard implements an API for tokens with smart contracts. For instance, here are three prominent functionalities ERC-20 provides:
- Get the current token balance of a wallet
- Transfer tokens between accounts
- Get the total supply available on a blockchain network
To summarize, an ERC-20 token is essentially a token implementing the ERC-20 token standard. What’s more, to be able to be called an ERC-20 token, the token smart contract needs to implement the following methods and events:
Methods:
function name() public view returns (string) function symbol() public view returns (string) function decimals() public view returns (uint8) function totalSupply() public view returns (uint256) function balanceOf(address _owner) public view returns (uint256 balance) function transfer(address _to, uint256 _value) public returns (bool success) function transferFrom(address _from, address _to, uint256 _value) public returns (bool success) function approve(address _spender, uint256 _value) public returns (bool success) function allowance(address _owner, address _spender) public view returns (uint256 remaining)
Events:
event Transfer(address indexed _from, address indexed _to, uint256 _value) event Approval(address indexed _owner, address indexed _spender, uint256 _value)
Summary – How to Get the Price of Any Ethereum Token
This article taught you how to get the price of any ERC-20 Ethereum token in three steps using Moralis:
- Setting up your project and Moralis
- Calling the Moralis
getTokenPrice
endpoint - Running the script
All you essentially needed to do was create a script for calling the getTokenPrice
endpoint. So, if you have stayed with us this far, you now know how to get any Ethereum token price. Also, if you watched the video from the ”Going Beyond…” section, you know how to incorporate this functionality into your projects. From here, it is now time to get creative and use your newly acquired skills to build sophisticated Web3 projects!
Along with a comprehensive walkthrough on how to get any Ethereum token, the article also explored ERC-20 tokens. In doing so, you learned about the ERC-20 standard and what it entails.
If you liked this article and want to explore Moralis’ amazing Web3 development content further, you can find other tutorials here on the Web3 blog. For example, check out our guide on how to create a blockchain explorer, explore the ins and outs of an Optimism Goerli faucet, or learn how to get blockchain data!
Also, if you have not already, make sure to sign up with Moralis right now. This will give you immediate access to all Moralis Web3 development tools. Creating your account is free, and you can immediately start leveraging the full potential of blockchain to build more efficiently!