Are you looking for the easiest way to get blockchain data? If so, you are exactly where you need to be! In this tutorial, we will show you how to query blockchain data with Moralis using the following:
- Web3 Data APIs from Moralis
- The Moralis Streams API
You can get a quick overview of these application programming interfaces in the following two subsections as we explore their most prominent features!
Web3 Data API Features
The Moralis Web3 Data API is a collection of highly scalable programming interfaces solving common blockchain challenges. By indexing all central aspects of blockchain data, Moralis provides a significantly more accessible developer experience, no matter your data needs. Via a suite of data-focused API endpoints, you can easily get and understand blockchain data!
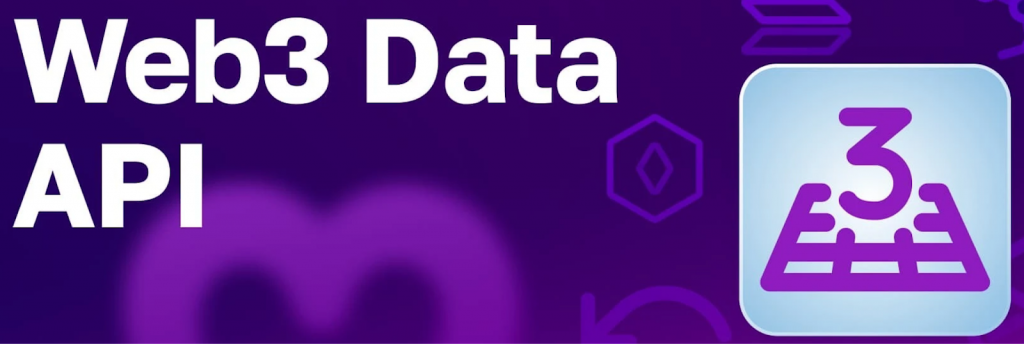
Here are five standard features of the Web3 Data APIs from Moralis:
- Token Data – Through the Web3 Data APIs, you can seamlessly access real-time ownership, transfer, and price data.
- NFT Data – Query real-time NFT metadata, ownership data, token prices, and much more.
- Block Data – Get the blockchain data of any block, including transactions, events, logs, and more.
- DeFi Data – Query out-of-the-box liquidity reserve and pair data over multiple blockchain networks effortlessly.
- Transaction Data – Get user transaction blockchain data with ease. To highlight the accessibility of the Web3 Data APIs, here is an example of how to use the
getWalletTransactions
endpoint:
const response = await Moralis.EvmApi.transaction.getWalletTransactions({ address, chain, });
Moralis Streams API Features
The Moralis Web3 Streams API allows you to listen to real-time, on-chain events effortlessly. All you need to do is set up your own stream to funnel blockchain data into your application’s backend via webhooks. You can find five prominent Moralis Streams API features here:
- Listen to contract or wallet events or both with several streams.
- Track one or millions of addresses with just a single stream.
- Listen to events from all contract addresses.
- Get blockchain events data streamed into applications’ backends in real time.
- Fully customize streams using filters to receive webhooks for particular events.
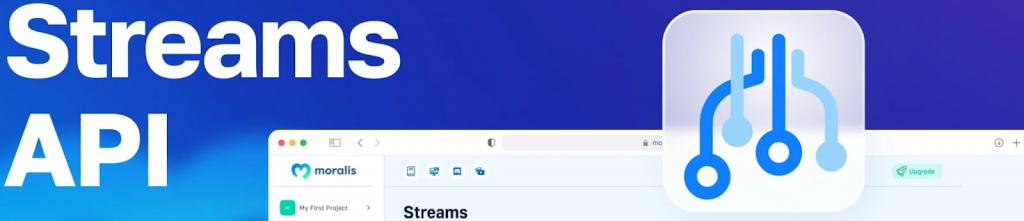
Here is an example of what a stream for monitoring incoming and outgoing transactions of a wallet can look like:
import Moralis from 'moralis'; import { EvmChain } from “@moralisweb3/common-evm-utils”; Moralis.start({ apiKey: 'YOUR_API_KEY', }); const stream = { chains: [EvmChain.ETHEREUM, EvmChain.POLYGON], // list of blockchains to monitor description: "monitor Bobs wallet", // your description tag: "bob", // give it a tag webhookUrl: "https://YOUR_WEBHOOK_URL", // webhook url to receive events, includeNativeTxs: true } const newStream = await Moralis.Streams.add(stream); const { id } = newStream.toJSON(); // { id: 'YOUR_STREAM_ID', ...newStream } // Now we attach bobs address to the stream const address = "0x68b3f12d6e8d85a8d3dbbc15bba9dc5103b888a4"; await Moralis.Streams.addAddress({ address, id });
If you want to access the Moralis Streams and Web3 Data APIs, remember to sign up with Moralis now! Creating an account is free, and you can immediately leverage the full power of blockchain technology to build smarter and more efficiently!
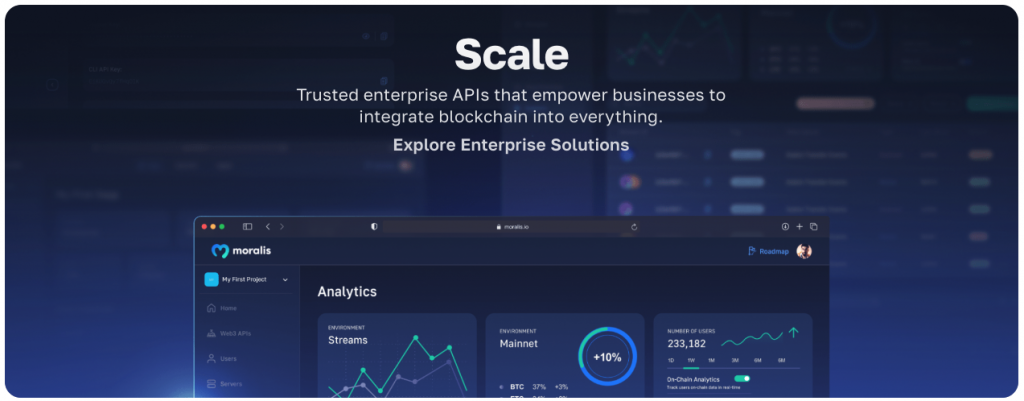
Overview
In today’s article, we will illustrate how to get blockchain data using the Moralis Web3 Data APIs and Moralis Streams API. If this sounds exciting and you are eager to get going, you can jump straight into the tutorial section by clicking here!
Along with two comprehensive tutorials on how to get blockchain data, the article also takes a deep dive into the intricacies of on-chain data. So, if you are unfamiliar with what it entails, we recommend starting in the ”Exploring Blockchain Data” section further down!
Remember to sign up with Moralis if you want to follow along in this article. A free account gives immediate access to the Streams API, Web3 Data APIs, and much more. Create your account now to leverage the full potential of the blockchain industry!
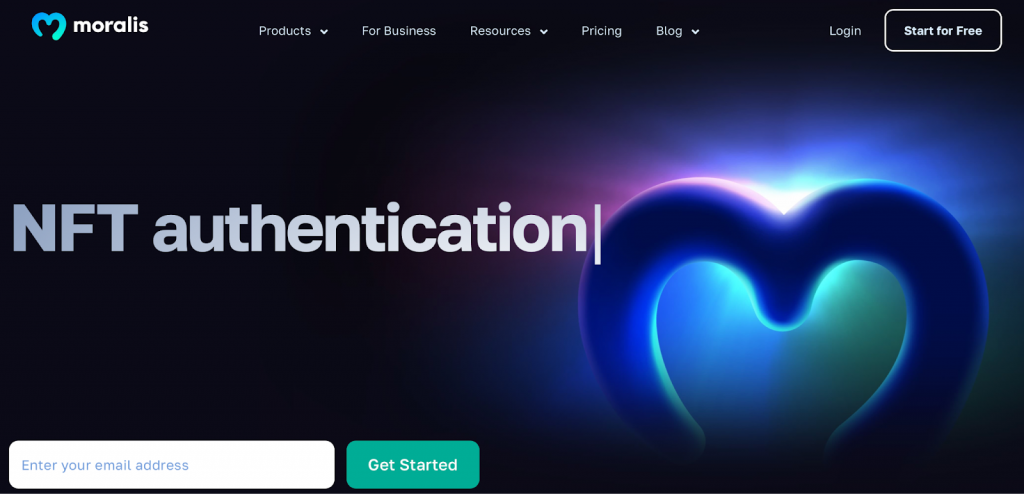
How to Get Blockchain Data
In the coming sections, you will learn how to query blockchain data using Moralis in two different ways. Let’s take a look at these in more detail before we kick things off.
- Moralis Web3 Data APIs – To begin with, we will teach you how to build an application for fetching the transaction data of a block based on its number. In doing so, you will familiarize yourself with the Moralis Web3 Data APIs.
- Moralis Streams API – For the second demo, we illustrate how to set up a stream for monitoring native transactions of a specific wallet using the Streams API.
So, without further ado, let’s jump straight into the former and explore how the Moralis Web3 Data APIs work!
Get Blockchain Data with the Web3 Data API
To kickstart this tutorial, we will show you how to get blockchain data using one of Web3 Data APIs. More specifically, you will learn to create a straightforward application allowing you to query blockchain data regarding transactions of a block based on its hash. You can find a print screen below of what the app looks like:
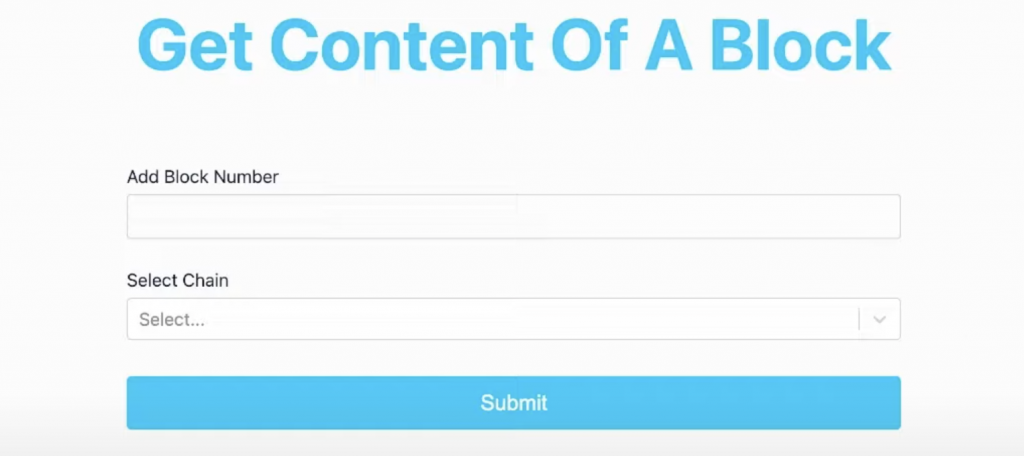
As you can see, you only need to add a block number, select a chain, and hit the ”Submit” button. In return, you receive information such as a timestamp, the transaction’s ”from” address, the gas price at the time, the transaction hash, etc.:

Creating the Application
Start by setting up a new NodeJS project. From there, visit the GitHub repository down below and clone the project to your local machine:
Get Blockchain Block Data Repository – https://github.com/MoralisWeb3/youtube-tutorials/tree/main/get-content-of-a-block
When you are done copying the project to your local directory, you need to make a few configurations. First, create a new file called ”.env” in the project’s backend folder. In this file, add a new environment variable called MORALIS_API_KEY
. It should look something like this:
MORALIS_API_KEY = ”replace_me”
You must replace replace_me
with your own Moralis API key. So, if you have not already, now is the time to sign up with Moralis. With an account at hand, you must log in, navigate to the ”Web3 APIs” tab, copy your key, and input the API key into your code:
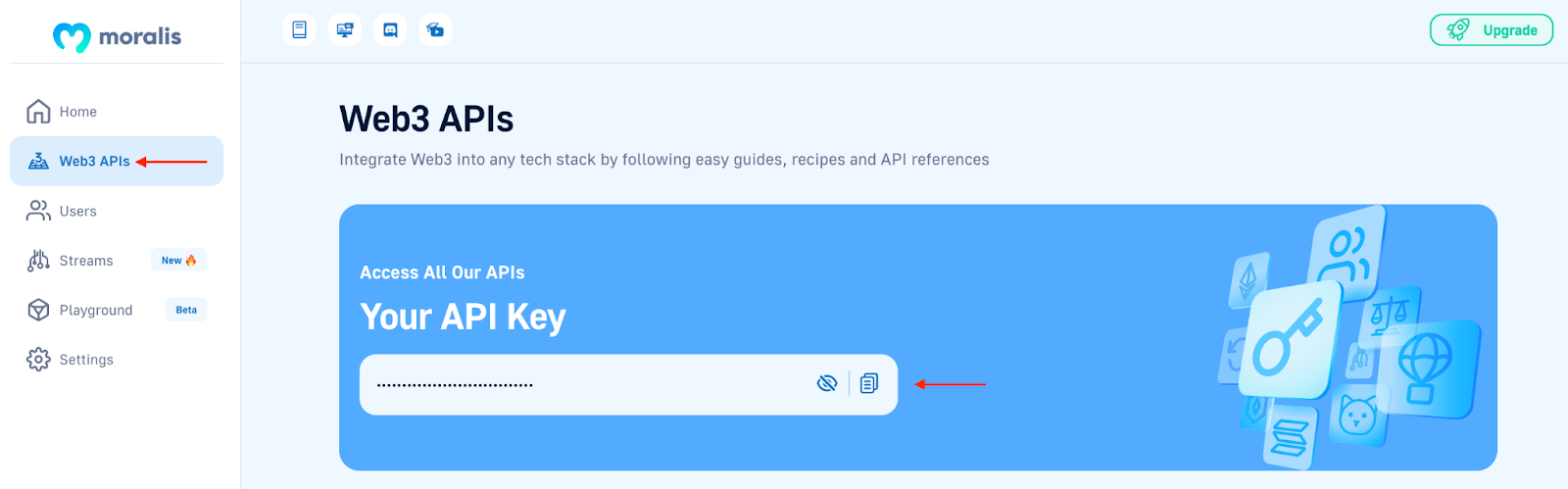
That is it; you should now be able to start the application by opening a new terminal and executing the following command:
npm run dev
Doing so starts your app on localhost 3000, and you can now continuously get blockchain data using the getBlock
endpoint. For a more detailed breakdown of the code and how the getBlock
endpoint works, check out the following video from the Moralis YouTube channel:
Listen to Blockchain Data with the Moralis Streams API
In this section, we will briefly illustrate how the Moralis Streams API works by showing you how to set up your very own stream for monitoring the native transactions of a wallet. The walkthrough is divided into three steps, and we will start by showing you how to create a server!
Step 1: Create a Server
To begin with, the first thing you need to do is set up a server. This server will be used to receive webhooks containing the requested blockchain data. As such, go ahead and create a NodeJS Express application and add the following contents:
const express = require("express"); const app = express(); const port = 3000; app.use(express.json()); app.post("/webhook", async (req, res) => { const {body} = req; try { console.log(body); } catch (e) { console.log(e); return res.status(400).json(); } return res.status(200).json(); }); app.listen(port, () => { console.log('Listening to streams') });
As you can see, the server features a ”/webhook”
endpoint, to which Moralis can post the streams. What’s more, the code also parses and console logs the body that Moralis sends. Once you have implemented the code, you can launch your server with the following terminal input:
npm run start
Step 2: Set Up a Webhook URL
For the second step, you need to use ngrok to create a tunnel to your port 3000
, as this is where your server is running. You can do so by opening a new terminal and running the following command:
ngrok http 3000
Executing the command above provides the following response containing your webhook URL:

Step 3: Create Your Web3 Stream
Finally, create another NodeJS file called “index.js” and use the following code:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); require("dotenv").config(); Moralis.start({ apiKey: process.env.MORALIS_KEY, }); async function streams(){ const options = { chains: [EvmChain.MUMBAI], description: "Listen to Transfers", includeConractLogs: false, includeNativeTxs: true, webhookURL: "YOUR_WEBHOOK_URL" } const newStream = await Moralis.Streams.add(options) const {id} = newStream.toJSON(); const address = "YOUR_WALLET_ADDRESS"; await Moralis.Streams.addAddress({address, id}) } streams()
In the snippet above, we first add a few imports, including Moralis, EVM utils, and dotenv. Next, we call the Moralis.start()
function, passing an API key as a parameter to initialize Moralis. Since displaying the API key directly in the code is a security risk, you need to set up a ”.env” file with an environment variable:
MORALIS_KEY = “replace_me”
Here, you need to replace replace_me
with your own Moralis API key. To get the key, sign up with Moralis, then log in, and navigate to the Web3 APIs tab:

From there, we define a couple of options. This includes the network you want to listen to, a stream description, a tag, your webhook URL, etc. Next, we create a new Moralis stream using the Moralis.Streams.add()
function while passing the options object as an argument. From there, we get the stream ID and add an address variable. Finally, we use the Moralis SDK to add the address.
Remember that you must replace YOUR_WEBHOOK_URL
and YOUR_WALLET_ADDRESS
with your own values.
That’s it! That’s how easy it is to query blockchain data when working with the Moralis Streams API! However, if you want a more comprehensive code breakdown, check out the video below. In this video, one of our talented software engineers walks you through the steps above in even more detail:
Exploring Blockchain Data
If you are unfamiliar with the concept of ”blockchain data”, this is probably a good starting point. In the coming sections, we will explore the intricacies of blockchain data and where this information is stored. So, let’s begin and answer the ”what is blockchain data?” question.
What is Blockchain Data?
Blockchain data, which is also called ”on-chain data”, refers to the publicly available information associated with transactions that have occurred on a blockchain network. In short, it is all the data from the blocks making up an entire blockchain. What’s more, with the transparency of these networks, the information is publicly available. Consequently, this means that anyone can query blockchain data if they need it!
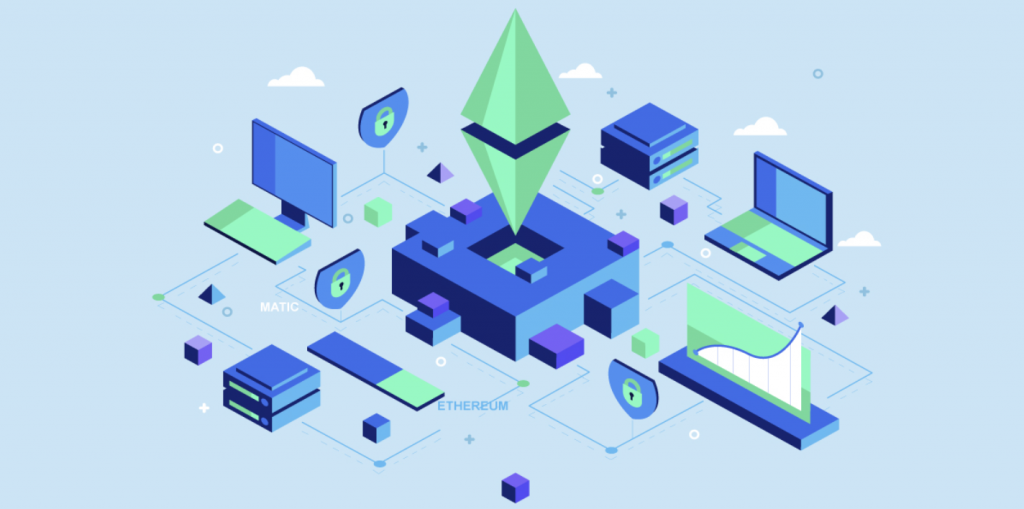
The blockchain’s transaction data is continuously recorded when transactions are validated. This record is generally immutable, meaning it is difficult to alter the information once it is validated. Moreover, this ensures the accuracy and security of this “digital ledger”, which is also why this data is valuable when developing Web3 platforms. Some different data types can, for instance, be wallet addresses, miner fees, transfer amounts, smart contract code, and much more.
To summarize, on-chain data is publicly available information regarding blocks, transactions, and smart contracts on a blockchain network. That said, where and how is this information actually stored?
Where is Blockchain Data Stored?
Blockchain data is, of course, stored on a blockchain network. To make this more understandable, let us briefly break down the concept of a blockchain. In essence, a blockchain is a type of distributed and decentralized ledger that stores, synchronizes, and publicly shares transactions and other blockchain data.
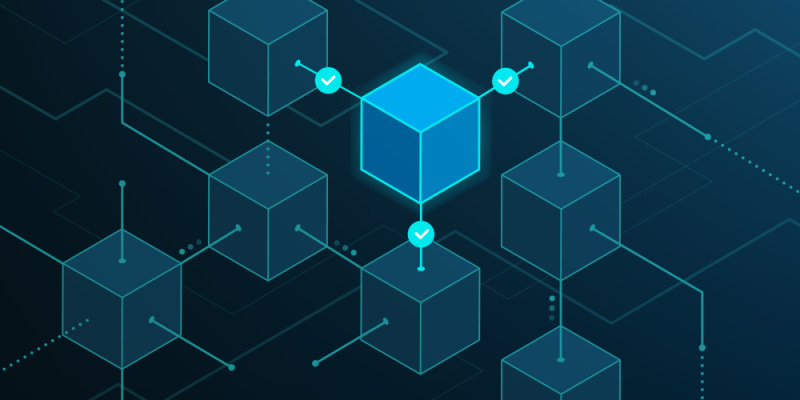
This data is stored in blocks linked together using cryptography and compromise a blockchain, hence the name. Each block generally consists of two main parts: a block header and a block body. So, let’s break down each of these, starting with the former:
- Block Header – The block header stores metadata. This includes everything from the block number to a timestamp.
- Block Body – This part contains the block’s transaction data.
With a better understanding of how blockchain data is stored, let us take the following section to explore the easiest way to access this information!
Easiest Way to Get Blockchain Data
From a conventional perspective, it has been relatively challenging to query blockchain data. Fortunately, this is no longer the case as the Web3 space has evolved, and infrastructure providers such as Moralis have emerged to facilitate a more seamless developer experience. In fact, with the Moralis Web3 Streams API and Web3 Data API, it has never been easier to query blockchain data!
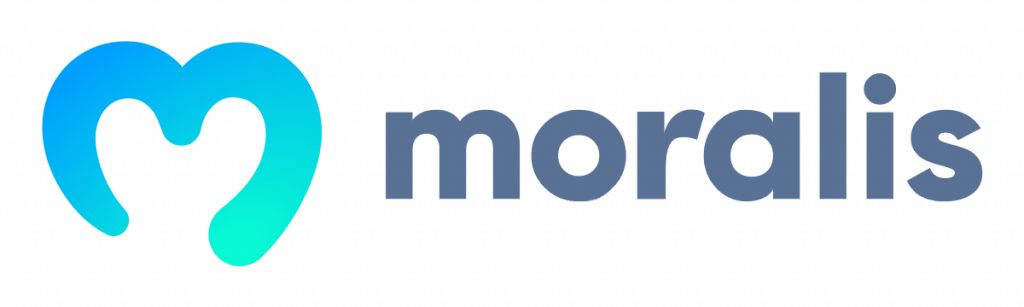
With the Streams API, you can seamlessly set up streams to funnel blockchain data directly into the backend of your Web3 projects. Also, you no longer need to worry about maintaining and connecting to buggy RPC nodes, building unnecessary abstractions, wasting time setting up complex data pipelines, etc. Instead, you can seamlessly create your own Web3 streams using the Moralis Streams API to receive webhooks whenever an asset is traded, someone partakes in a tokens sale, a battle starts in a video game, or any other smart contract events fire based on filters specified by you.
Along with setting up streams to receive webhooks based on smart contract events, you can also use the Web3 Data API to seamlessly query blockchain data. Moralis indexes all core aspects of on-chain data and provides effortless access through data-focused API endpoints. As such, it does not matter what Web3 project you are building; the Web3 Data APIs support all the data you need. This includes transactions, NFTs, balances, blocks, and much more!
If you find this interesting, check out the Ethereum price API and the Binance NFT API.
So, if you want to get into Web3 development, sign up with Moralis right now to gain immediate access to all of Moralis’ unique features, all for free!
Summary – How to Get Blockchain Data
If you have followed along this far, you have familiarized yourself with the Moralis Streams API and Web3 Data APIs. As such, you can now set up your own Web3 stream and use the various endpoints from Moralis to query blockchain data!
If you found this article helpful, consider checking out additional content on the Web3 blog. For example, check out our NFT smart contract example, read more about the best Ethereum development tools and scaling solutions for Ethereum, or learn how to build a decentralized cryptocurrency exchange. Also, make sure to check out our gwei to ETH converter!
In the aforementioned tutorials, you will get to work with amazing Web3 Data APIs from Moralis, enabling you to leverage the power of blockchain. So, if you want to build Web3 projects yourself, remember to sign up with Moralis!