Building NFT-related dapps (decentralized applications) requires the best tools. Thanks to the ultimate NFT API, you can easily integrate specific features for your dapps, such as getting all NFTs owned by an address. To get all NFTs owned by address, you can use the following script that includes the “getWalletNFTs” endpoint:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/evm-utils"); const runApp = () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const address = "0xd8da6bf26964af9d7eed9e03e53415d37aa96045"; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.nft.getWalletNFTs({ address, chain, }); console.log(response); } runApp();
If you’ve worked with Moralis before, you probably know how to use the above lines of code. Hence, simply use the link below to get to the documentation page to get started immediately. However, if this is your first rodeo with Moralis and you would like a step-by-step process on how to get all NFTs owned by address, make sure to complete today’s three-step tutorial!
Overview
Moving forward, we’ll first do a proper overview of the topic to ensure you all know what NFTs and Web3 addresses are. Then, we’ll take a closer look at the “getWalletNFTs” endpoint, where we’ll also explore its parameters. Finally, we’ll take you through the three steps you need to complete to get all NFTs owned by address.
Furthermore, we’ll focus on the Ethereum chain in this article. However, we’ll also explain how to tweak a single snippet of code to tackle other leading programmable chains. After all, Moralis is all about cross-chain interoperability. As a result, Moralis lets you develop on major blockchain networks, including BNB Smart Chain, Avalanche, Polygon, and more! With that said, if you want to learn how to get all NFTs owned by address the easy way, create your free Moralis account and follow our lead!
What are NFTs and Web3 Addresses?
Non-fungible tokens are special kinds of crypto assets. Unlike fungible tokens, NFTs are not interchangeable. After all, each NFT has its unique token ID (on-chain signature) that makes it different from any other NFT. This property makes non-fungible tokens great for digital art and digital collectibles. Also, since NFTs can represent all sorts of files, they are starting to revolutionize real-world use cases. Moreover, NFTs may serve as proof of ownership, certifications, and more. Of course, NFTs are also changing the gaming industry. Players can truly own their game progress by tokenizing (converting to NFTs) in-game assets. Consequently, they can trade their NFTs and transfer their in-game assets into the world economy.
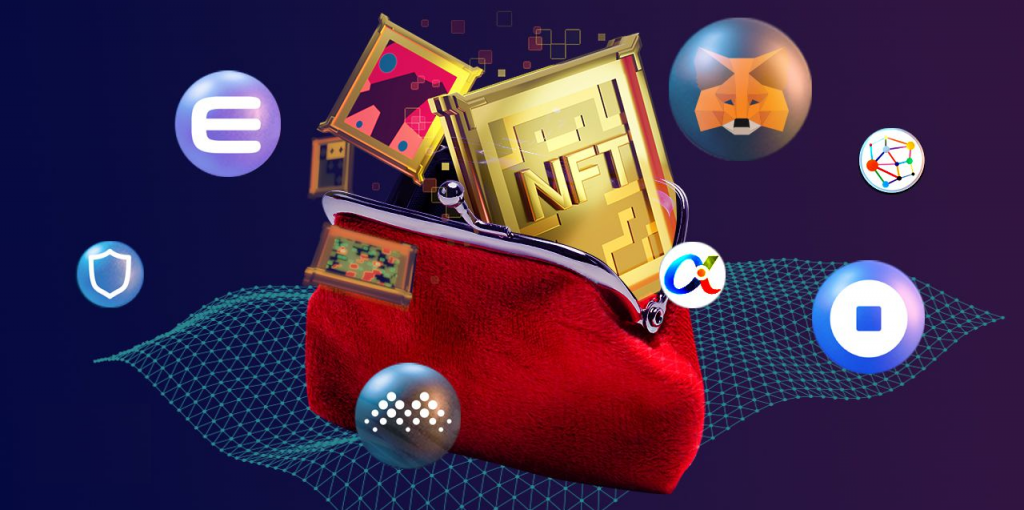
Furthermore, it’s worth pointing out that NFTs need to be minted. This is the process of executing an on-chain transaction that stores an NFT’s metadata on the blockchain. Moreover, minting NFTs is done by deploying smart contracts (on-chain pieces of software), which contain predefined conditions and actions regarding the NFT(s) they focus on. Also, to ensure that things follow established guidelines, there are different smart contracts for different NFT standards. The most common ones are ERC-721 and ERC-1155. Remember that every NFT is assigned to the smart contract used to mint it. Accordingly, you can also get all NFTs from contract.
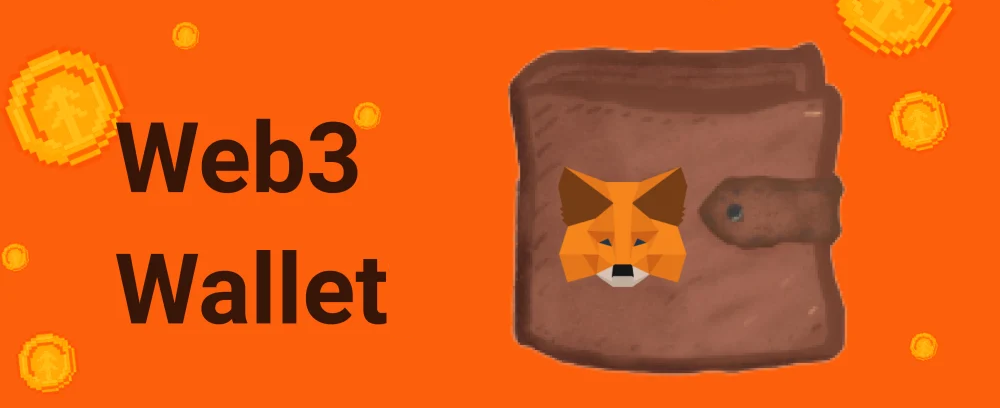
However, once users buy or gain ownership of an NFT, the latter is transferred to their Web3 wallet. Moreover, a Web3 wallet can come in many forms, but essentially, it is a combination of private and public keys. The public keys are known as blockchain addresses. Hence, we can get all NFTs owned by address.
NFT API to Get All NFTs Owned by Address
This section takes a closer look at Moralis’ “getWalletNFTs” endpoint. The latter enables devs to get all NFTs owned by address without breaking a sweat. Further, this NFT API endpoint returns the response that includes the “[SYNCED/SYNCING]” status based on the contracts that the code is indexing. This endpoint’s results include all indexed NFTs. Any request that includes the “token_adress” parameter will start the indexing process for that NFT collection the first time the script requests it. In addition, the “getWalletNFTs” endpoint includes several optional query parameters, which help you further refine your search.
Ultimately, here are all the parameters you can use when working with the above-stated NFT API endpoint:
- “address” – This is the NFT owner’s blockchain address and the only required parameter.
- “chain“ – This parameter lets you determine the chain you want to query. You can choose among all supported chains.
- “format“ – You can choose between the decimal or HEX format of the token ID.
- “limit“ – This parameter enables you to set the desired page size of the result.
- “token_addresses” – You can use an array of strings representing different addresses you want to get balances for.
- “cursor“ – The cursor parameter comes in the previous response and serves for getting the next page.
How to Explore the “getWalletNFTs” Endpoint Yourself
If you want to explore the “getWalletNFTs” endpoint and even view its response for specific parameters, make sure to visit the Moralis documentation:
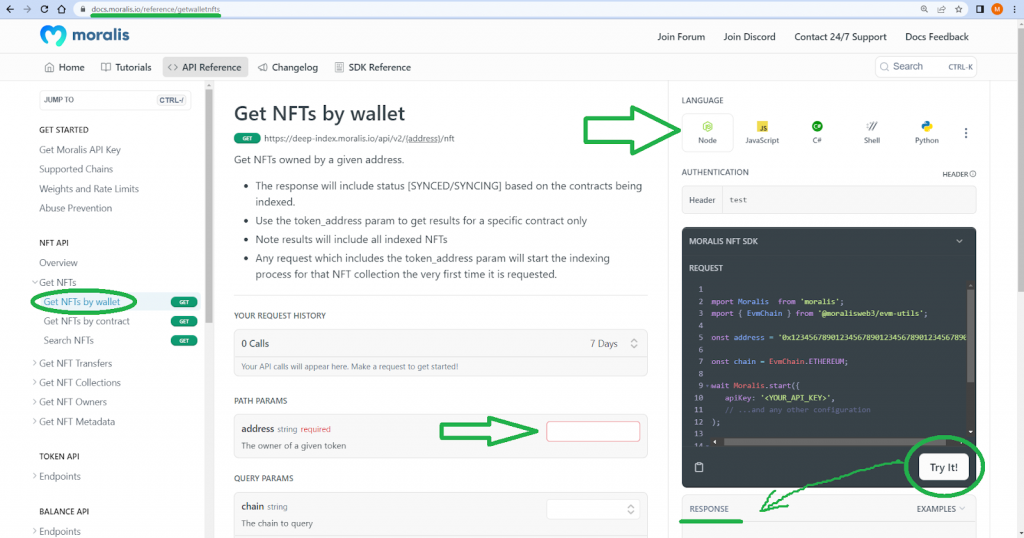
As the above screenshot indicates, you must enter a wallet address first. Of course, you can also add any of the optional parameters. Next, you must select the programming language in the top-right part of the page. Finally, you can hit the “Try It!” button and view the response below. However, to see the real power of this Moralis’ NFT API endpoint, you’ll need to paste in your Moralis Web3 API key. You can learn how to obtain that key with two clicks in the “Step 1: Set Up Moralis” section below.
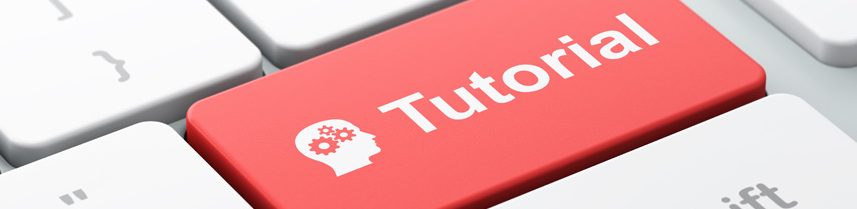
3-Step Tutorial – How to Get All NFTs Owned By Address
Before we take you through the details of this three-step tutorial, it’s worth pointing out that being able to get all NFTs owned by address is extremely useful. After all, you know that NFTs can play significant roles in many dapps. Devs use these types of tokens to represent ownership of a unique item – either virtual or physical – on the blockchain. Some of the most common NFT utility use cases include NFT portfolio trackers, NFT-gated websites, DAOs, and social graphs. As such, all of these require the backend code to get all NFTs owned by address. Hence, by mastering the upcoming three steps, you’ll be able to build all sorts of NFT dapps.
Furthermore, there are some prerequisites you need to take care of before you can start implementing the code:
- You should have Node v.14 or higher ready.
- Have your favorite code editor or IDE installed and set up. We prefer to use Visual Studio Code (VSC).
- Also, make sure you have your favorite package manager (“npm“, “yarn“, or “pnpm“) installed.
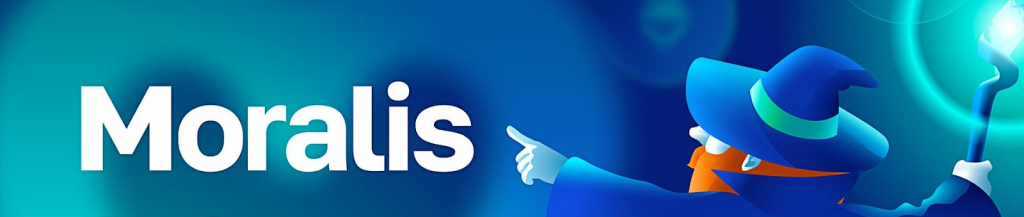
Step 1: Set Up Moralis
If you haven’t done so, create your free Moralis account now. You can use the link stated at the outset of this article or visit the Moralis homepage and click on the “Start for Free” button:
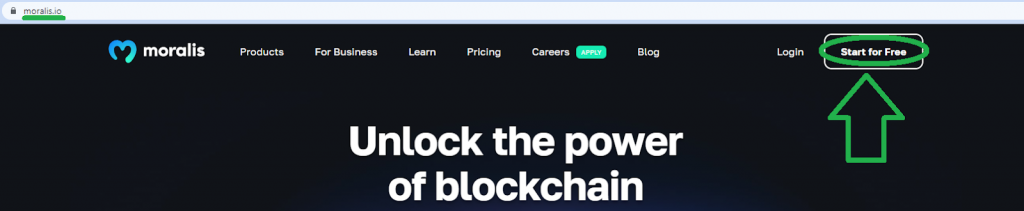
Whichever path you choose, you’ll land on the register page, where you need to enter your details:
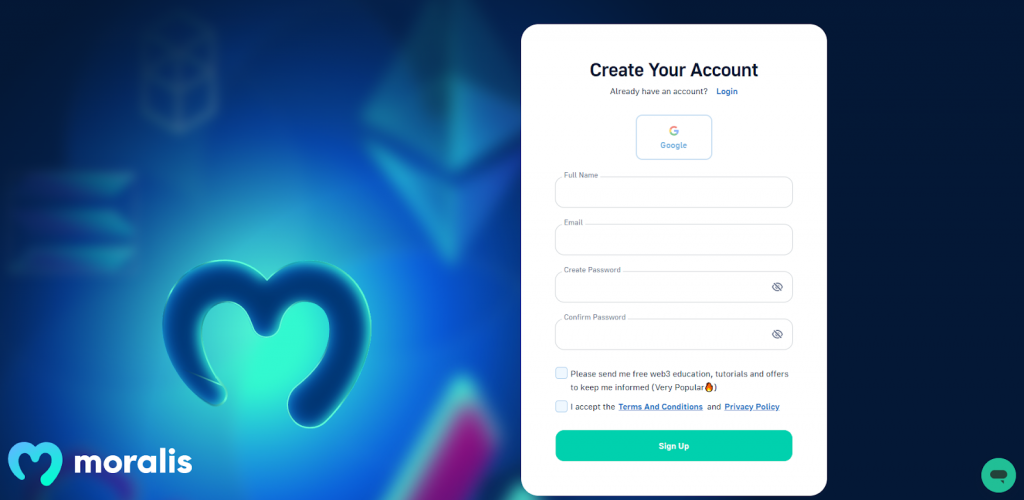
Once you enter your full name, email address, and password and accept the terms and conditions, you’ll be able to hit the “Sign Up” button. Then, you just need to confirm your account by clicking on the link that will arrive in your email inbox.
With your Moralis account ready, you can obtain your Moralis Web3 API key. To do this, select the “Web3 APIs” option in your admin area. Then, use the copy icon next to your API key:

We’ll show you where to paste your API key shortly. However, first, you must install the Moralis SDK in your project. To do this, use your terminal and enter one of the following commands (depending on the package manager that you are using):
npm install moralis
yarn add moralis
pnpm add moralis
Step 2: Get All NFTs Owned By Address
With the Moralis SDK installed, you can use the lines of code provided at the top of this article. If you remember, the “getWalletNFTs” endpoint is the script’s core. It uses the required “address” and optional “chain” parameters. As such, you must decide which chain you will focus on and which address you will explore. Of course, you can follow our lead and use the example address in the “index” script and focus on the Ethereum chain.
By looking at the lines of code below, you can see that we use “EvmChain.ETHEREUM” to define the chain we want to focus on. So, if you shift your attention to any other chain, you’d need to change that line of code.
Here are the supported EVM-compatible chains and the snippets of code you need to replace “ETHEREUM” with:
- Ethereum Görli: “GOERLI”
- Ethereum Sepolia: “SEPOLIA”
- Polygon Mainnet: “POLYGON”
- Polygon Mumbai Testnet: “MUMBAI”
- BNB Smart Chain Mainnet: “BSC”
- BNB Smart Chain Testnet: “BSC_TESTNET”
- Avalanche C-Chain: “AVALANCHE”
- Avalanche Fuji Testnet: “FUJI”
- Fantom: “FANTOM”
- Cronos Mainnet: “CRONOS”
- Cronos Testnet: “CRONOS_TESTNET”
Note: In addition to the above chains, you can use the Moralis Solana API. However, since Solana is in a non-EVM-compatible chain, you need to tweak other lines of code and use a different API endpoint.

Use JavaScript or TypeScript
Below is the same code as in the intro. However, we optimized it below for TypeScript (TS) while it was in the JavaScript (JS) format at the outset. So, this is what the “index.ts” script that enables you to get all NFTs owned by address on Ethereum looks like:
import Moralis from "moralis"; import { EvmChain } from "@moralisweb3/evm-utils"; const runApp = () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const address = "0xd8da6bf26964af9d7eed9e03e53415d37aa96045"; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.nft.getWalletNFTs({ address, chain, }); console.log(response); } runApp();
Whether you use JS or TS, do not forget to replace the “YOUR_API_KEY” placeholder with your Web3 API key. Also, feel free to use other addresses and chains, as explained above.
Step 3: Execute the Program and Explore the Results
With your “index.js” or “index.ts” file ready, it’s time to execute the program. Hence, use one of the following commands:
node index.js
node index.ts
After running the appropriate command, you’ll see the results in your terminal. Here’s what the results look like for the “0xd8da6bf26964af9d7eed9e03e53415d37aa96045” address on Ethereum:
{ "total": 1456, "page": 1, "page_size": 100, "cursor": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjdXN0b21QYXJhbXMiOnsid2FsbGV0QWRkcmVzcyI6IjB4ZDhkYTZiZjI2OTY0YWY5ZDdlZWQ5ZTAzZTUzNDE1ZDM3YWE5NjA0NSJ9LCJrZXlzIjpbIjE2NjMyMzgxNzUuMDc3Il0sIndoZXJlIjp7Im93bmVyX29mIjoiMHhkOGRhNmJmMjY5NjRhZjlkN2VlZDllMDNlNTM0MTVkMzdhYTk2MDQ1In0sImxpbWl0IjoxMDAsIm9mZnNldCI6MCwib3JkZXIiOltdLCJ0b3RhbCI6MTQ1NiwicGFnZSI6MSwidGFpbE9mZnNldCI6MSwiaWF0IjoxNjY2NjgyNTUyfQ.E5DkWYvRTaFnVhgedRuT3IW-rb2V-ikFKwP2cg2Qf78", "result": [ { "token_address": "0x57f1887a8bf19b14fc0df6fd9b2acc9af147ea85", "token_id": "4765809967066625256798886812262830659450023020194524584471225959000376492819", "amount": "1", "owner_of": "0xd8da6bf26964af9d7eed9e03e53415d37aa96045", "token_hash": "ba6d44b5f16be94283cecffeb784b7ca", "block_number_minted": "15572796", "block_number": "15573017", "contract_type": "ERC721", "name": "Ethereum Name Service", "symbol": "ENS", "token_uri": null, "metadata": null, "last_token_uri_sync": null, "last_metadata_sync": "2022-09-20T06:06:08.153Z", "minter_address": null }, { "token_address": "0x57f1887a8bf19b14fc0df6fd9b2acc9af147ea85", "token_id": "84453794918345416145331514647027903846664455083247396107154093349515123913389", "amount": "1", "owner_of": "0xd8da6bf26964af9d7eed9e03e53415d37aa96045", "token_hash": "7c0212cd3daf1b6b64f193c6dc102fb4", "block_number_minted": "15572811", "block_number": "15573017", "contract_type": "ERC721", "name": "Ethereum Name Service", "symbol": "ENS", "token_uri": null, "metadata": null, "last_token_uri_sync": null, "last_metadata_sync": "2022-09-20T06:09:09.838Z", "minter_address": null }, { "token_address": "0x57f1887a8bf19b14fc0df6fd9b2acc9af147ea85", "token_id": "16476931145019337030786748713476010946621971075817308111460324192065814192354", "amount": "1", "owner_of": "0xd8da6bf26964af9d7eed9e03e53415d37aa96045", "token_hash": "627ffc76405a90ee940cb91f7e90b294", "block_number_minted": "15572818", "block_number": "15573017", "contract_type": "ERC721", "name": "Ethereum Name Service", "symbol": "ENS", "token_uri": null, "metadata": null, "last_token_uri_sync": null, "last_metadata_sync": "2022-09-20T06:11:34.545Z", "minter_address": null }, ], "status": "SYNCED" }
We encourage you to run the above program for various addresses on different chains and explore the results returned by your terminal. Then, put your new ability to further use by creating an actual dapp that will either display users’ NFTs or grant them access based on their ownership of specific NFTs. If that sounds interesting, make sure to take on our “NFT-gated website” tutorial in our documentation.
However, in case you are interested in blockchain gaming development, our Web3 Unity content is just what you need. The latter awaits you on the Moralis YouTube channel and the Moralis blog.
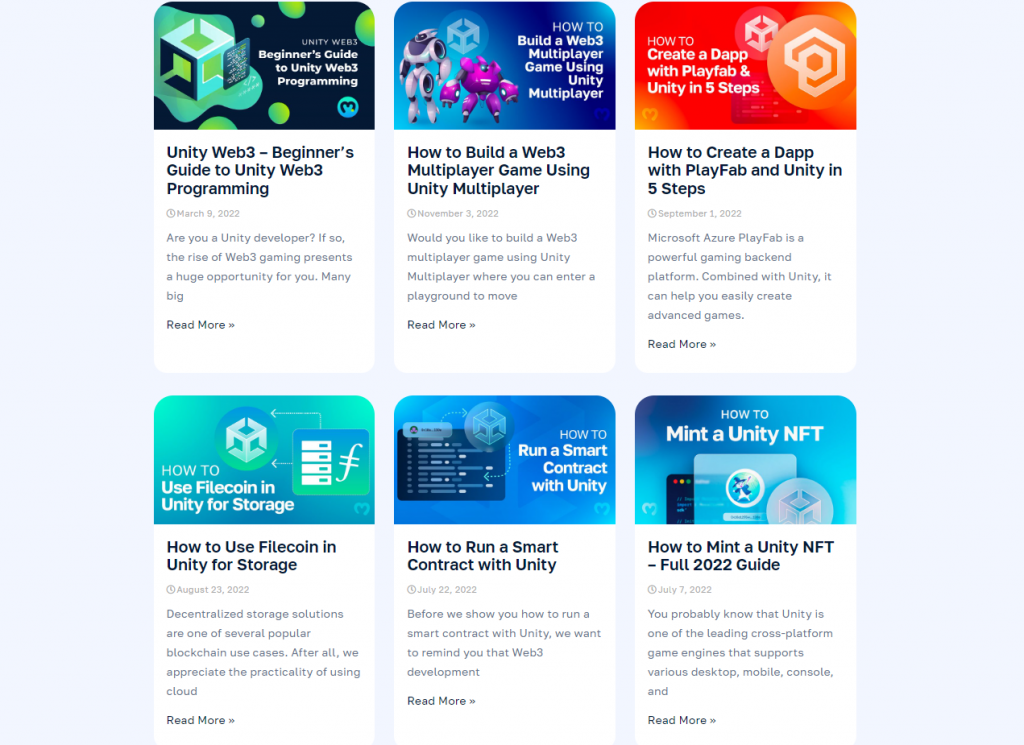
How to Get All NFTs Owned by an Address – 3-Step Process – Summary
We covered a lot of ground in today’s article. First, we did a quick overview of NFTs and Web3 wallets, where you also learned what blockchain addresses are. Next, we looked closely at the Moralis NFT API endpoint – “getWalletNFTs“. This is where you found out that you can test this endpoint (and all others) using the Moralis docs. You also learned how to easily select the programming language and copy the appropriate lines of code. Finally, we took you through our three-step tutorial illustrating how to get all NFTs owned by address effortlessly. Accordingly, you had a chance to complete the following three steps:
- Set Up Moralis
- Create a Script to Get All NFTs Owned by Address
- Execute the Program and Explore the Results
By using the knowledge and skills obtained herein, you can easily start creating some killer dapps. With the Moralis docs at your side, you should be able to do that using your favorite programming framework. Furthermore, you can stick to NFT utility or shift your focus to other types of dapps. In either case, you can get additional guidance from other Moralis resources. Both our YouTube channel and blog are great outlets to support your ongoing crypto education for free. Some of our latest topics cover the best crypto wallet API, the best Web3 provider, Web3 for business, a Web3 JS tutorial, building a Web3 Unity multiplayer game, and much more.
Of course, you can additionally speed up your process of becoming a blockchain expert by enrolling in Moralis Academy. There you get to explore the full scope of blockchain development. If you are new to the crypto domain, we recommend starting with blockchain and Bitcoin fundamentals.