Verifying smart contracts is crucial since using defective contracts can cause devastating results, such as economic losses. But how can you verify a smart contract? One of the best ways to accomplish this is by using Hardhat. By following along in this article, you’ll learn how to verify a smart contract with Hardhat by completing the following five steps:
- 1. Set Up Hardhat
- 2. Create an NFT Smart Contract
- Here, we’re going to create a contract looking like this:
contract NFT is ERC1155 { constructor() ERC1155(“URL”){ _mint(msg.sender, 0, 1, “”); } }
- 3. Create a Deployment Script
- 4. Modify “hardhat.config.js”
- 5. Run Scripts and Verify the Contract
- In this final step, we’re going to run the following Hardhat verification command:
npx hardhat verify “ADDRESS” --network goerli
If you’d like to dive into the complete tutorial right away of the above steps, click here to jump into the “How to Verify a Smart Contract in 5 Steps Using Hardhat” section. Below the tutorial, we’ll explore what Hardhat is in further detail. After the tutorial, make sure to sign up with Moralis if you’re interested in taking your Web3 development skills to the next level! Also, by signing up, you can receive the latest news, product updates, and more!
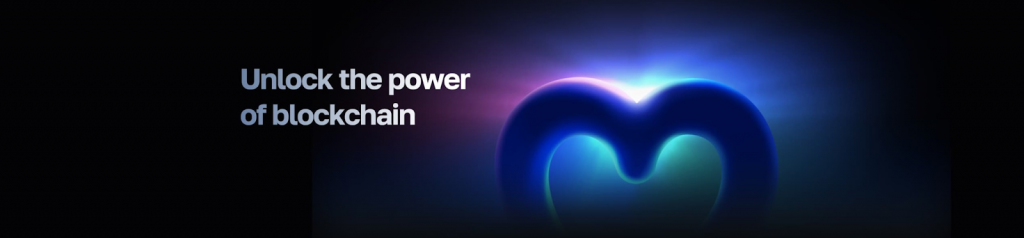
Overview
Smart contracts are a vital part of Web3 development, and they hold an essential role in the blockchain ecosystem of dapps. Since they have such a crucial function, it’s critical that they work as intended and remain entirely secure. This is because incomplete and malfunctioning smart contracts can lead to vast economic losses for businesses. For this reason, it’s highly beneficial to verify smart contracts to check their validity, correctness, and security. As such, we’re going to look closer at the process of how to verify a smart contract using Hardhat.
To make the process straightforward, we’re relying on instructions from Moralis. Moralis is the industry’s leading Web3 API provider, and with this provider, users gain access to a fully functioning Web3 backend infrastructure. What’s more, Moralis provides a selection of great Web3 data tools, such as the EVM API and Moralis Streams API. Also, all of the APIs from Moralis feature cross-chain compatibility!
How to Verify a Smart Contract in 5 Steps Using Hardhat
The process of how to verify a smart contract doesn’t have to be complicated. With the proper tools, we can accomplish this in just a matter of minutes. For this example, we’ll use Hardhat, Visual Studio Code, and some other tools!
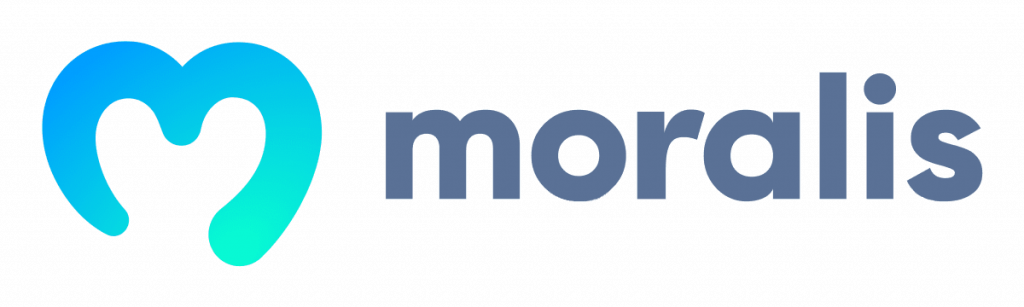
Although we’ve already outlined the steps of verifying with Hardhat, let’s look at the steps again on how we can accomplish the process:
- Set Up Hardhat
- Create an NFT Smart Contract
- Create a Deployment Script
- Modify “hardhat.config.js”
- Run Scripts and Verify the Contract
In the proceeding sections, we’ll provide you with a complete walkthrough of the process where we’ll create a contract, deploy it to the Goerli testnet, and finally verify the contract using Hardhat. So, if you’re interested, follow along as we start with installing Hardhat and setting up our own project!
Step 1: Set Up Hardhat
First and foremost, we must install Hardhat before we can begin creating our project. Installing Hardhat is relatively easy, and all we need to do is input the following command into the terminal of our source code editor:
npm install hardhat
This will immediately install Hardhat, and it will only take a couple of seconds. Once the development environment is installed, we kick off the process by initiating a new Hardhat project. To create a new project, we need to input npx hardhat
into the terminal. This command will provide us as developers with a few different options. The first thing we need to determine is what kind of project we would like to create. In our case, we’re going to select a basic sample project. Once we’re done selecting the project, we set the root for the project, and finally, we can also add a .gitignore
.

Once we’re done with our selections, we’re provided with a basic structure for our project. For example, the sample project we just created contains some files by default, such as a smart contract called ”Greeter.sol”.
With our sample project at hand, we can continue with installing the OpenZeppelin package. We can do this by inputting the following command into the terminal:
npm install @openzeppelin/contracts
This will supply us with all the different OpenZeppelin contracts so that we can import them into our code. That’s the final step for setting up our environment. We can now move on to the next step and explore how to create a smart contract that we’ll later verify with Hardhat!
Step 2: Create an NFT Smart Contract
In the previous step, we mentioned the default smart contract ”Greeter.sol”. We can begin by deleting it and instead create a new contract. We can call this new contract ”NFT.sol”, which will be a fundamental NFT smart contract. Since this is a tutorial on how to verify a smart contract with Hardhat, we’re not going to put a lot of effort into creating our contract. So, if you’re interested, the Moralis blog offers you a complete guide on how to create an ERC-721 NFT. Nonetheless, this is the complete file:
pragma solidity ^0.8.0; import “@openzeppelin/contracts/token/ERC1155/ERC1155.sol”; contract NFT is ERC1155 { constructor() ERC1155(“URL”){ _mint(msg.sender, 0, 1, “”); } }
As you can see from the image above, we’re implementing a pragma line into our code. This line determines which version of Solidity is to be used when we compile our contract. Once we have established the correct Solidity version, we must also import the proper OpenZeppelin contract, which in this case, is the contract for an ERC-1155 token.
The contract itself follows the import line, and we are naming the contract NFT
and then determining that it will inherit from the ERC-1155 token contract that we previously imported. After this, we add the contract’s constructor
, which is followed by a second ERC-1155 constructor. The latter must pass an argument, and in this example, we’re simply going to input the string URL
. However, when creating a real NFT, we would rather pass a URI
as the argument instead.
Finally, within the curly braces of our constructor, we simply initiate the mint function that, in turn, takes a few arguments. For example, the first argument, msg.sender
, ensures that the NFT(s) are provided to the wallet used to deploy the contract.
Once we’re done creating our contract, we can go ahead and compile the smart contract using Hardhat. To do so, we input the following command into the terminal:
npx hardhat compile
Step 3: Create a Deployment Script
Within the default structure of our Hardhat sample project, we’ll find a JavaScript file called ”sample.script.js”, which we can use to create our own deployment script. So, we can begin by renaming the script to ”deploy.js” and use the file’s basic structure to create the script.
All we really need to do here is rename and change some of the parameters within the file. We’re going to change everything that says Greeter
and replace it with NFT
or nft
. So, we are, for example, going to set getContractFactory
to NFT
and then remove the constructor arguments from the next line in the code. As such, this is what the changes will look like:
const NFT = await hre.ethers.getContractFactory(“NFT”); const nft = await NFT.deploy(); await nft.deployed(); console.log(“NFT deployed to:”, nft.address);
However, this is not the complete ”deploy.js” file; this is only to showcase what we’ve changed. The other part of the file remains completely unaltered.
Step 4: Modify “hardhat.config.js”
The fourth step in the process will involve making some minor modifications to the ”hardhat.config.js” file. However, before doing so, we’re going to install the verify plugin. To install this plugin, all we need to do is input the following command into the terminal:
npm install @nomiclabs/hardhat-etherscan
Once the plugin is installed, we also need to load the module by implementing the require()
function. There should already be an existing require()
function call at the beginning of the file, and all we need to do is write require(”@nomiclabs/hardhat-etherscan”);
below this function.
Next up, we must also modify module.exports
, which can be found further down in the code. We’ll create a networks
object where we add the Goerli network with a few secret details.
We’re going to need a URL and a private key which we’re going to fetch from the ”secrets.json” file, which we’ll create in just a moment. The last thing we need to do is to add an Etherscan API key to the config file. To fetch the API key, we must create an Etherscan account. Once we have an account, we can click our profile and navigate to the ”My API Keys” page. Here we can create a new API key that we can copy and paste into our code.
This is what the module looks like once we’re done with the modifications:
module.exports = { solidity: “0.8.4”, networks: { goerli: { url: secrets.url, accounts: [secrets.key] } }, etherscan: { apiKey: “INSERT_API_KEY_HERE” } };
Create a ”secrets.json” File
To enable our networks
object to access the secret URL and key, we must create a ”secrets.json” (JSON) file. The reason for doing this is because of the sensitive information stored, which is not something we’d like to share with others.
As such, we can go ahead and create a file called ”secrets.json”. In this file, we’ll add url
, which you can get from whatever node provider you decide to use. For example, you can use Alchemy or QuickNode. Next, you can get the key
info from our MetaMask account. This is what the file should look like:
{ “url”: “INSERT_URL_HERE” “key”: “INSERT_KEY_HERE” }
Since we’re going to deploy the contract to the Goerli testnet, we want to copy the testnet URL from your provider and implement it into our code. Moving on, we can find the private key of our MetaMask account by clicking on the three dots in our MetaMask interface and then selecting ”Account details”. Then, we copy the key and implement it into our code from above.
Once we’re done with the file, we must also load the module with an additional require()
function. As such, these will be the three require()
function calls of the ”hardhat.config.js” file:
require(“@nomiclabs/hardhat-waffle”); require(“@nomiclabs/hardhat-etherscan”); let secrets = require(“./secrets”);
Step 5: Run Scripts and Verify the Contract
Now that we’ve completed all the previous steps of creating a smart contract and a deployment script and also modified the config file, we have everything we need to verify our smart contract. So, the first thing we can do is go ahead and deploy our contract to the blockchain using the following command:
npx hardhat run script/deploy.js --network goerli
However, the account we added holding the private key to the ”secrets.json” file must contain tokens for the Goerli testnet to be able to run. If it doesn’t, all you need to do is find a crypto faucet, such as our Goerli testnet faucet, and input your wallet address to receive some.
Once we execute the previous command, we’ll be provided with an address to where the contract was deployed. We can then use this address (without the quotation marks in the example below) to run the verification command, which looks like this:
npx hardhat verify “ADDRESS” --network goerli
That’s it; congratulations! You have verified your own smart contract with Hardhat! If you still have questions regarding the process, we recommend reaching out to our experts at our Moralis forum. You can also join our Discord channel!
What is Hardhat?
Hardhat is an environment developers use to compile, test, deploy, and debug Ethereum based dApps. This means that it helps developers and coders to manage many of the native tasks of developing smart contracts.
Hardhat comes with an already pre-built local Ethereum network with a focus on development. The network is specified for Solidity debugging and features error messages, stack traces, among other things. As such, this environment is especially helpful in enabling developers to understand where their dApps fail and how they can solve the issues.
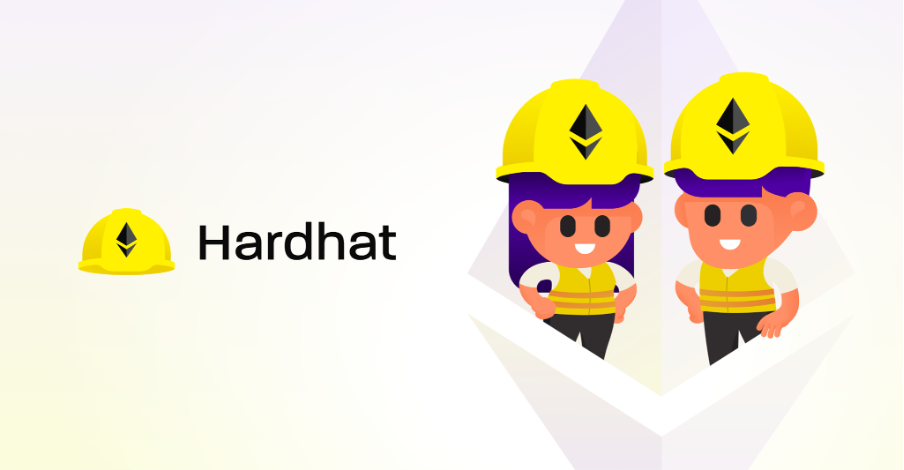
Furthermore, plugins characterize Hardhat, and this is where most of the functionalities originate. As such, it’s a modular design allowing users to choose which plugins to include in their development process.
However, this only covers the basics of Hardhat, and if you’re interested in more information, then check out our article from Moralis explaining what Hardhat is in more detail.
How to Verify a Smart Contract with Hardhat – Summary
In this article, we took you through a simple five-step process, demonstrating how to easily verify a smart contract with Hardhat.
Following these five steps allows us to verify any smart contract in a matter of minutes. In this article, we decided to upload the contract to the Goerli testnet, but the fundamentals of the process remain the same for any other blockchain network. However, if you decide to opt for another chain, there are a few alterations that need to be made regarding the process.
Moreover, this is only one of the many scenarios in which the Moralis platform comes in handy. For example, you can also use Moralis when you build a cryptocurrency portfolio dashboard, create your own ERC-20 token, or want to learn more about Web3 marketplace development. Furthermore, if you’re interested in learning more about Moralis, we recommend checking out the Moralis blog. The blog features great articles to better understand the potential of working with the industry’s leading Web3 API provider. So, if you are looking to verify contracts with Hardhat or develop dapps and tokens, you should sign up with Moralis today. As a Moralis user, you’ll gain access to all the platform’s tools, allowing you to save valuable time and resources throughout the complete development process!