This tutorial will teach you to use the Python-compatible Web3 Data API from Moralis to pull cryptocurrency prices, transactions, balances, and more! If this sounds interesting and you are eager to get crypto data using Python, you can find three core endpoints examples below:
get_token_price()
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "address": "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599", "chain": "eth" } result = evm_api.token.get_token_price( api_key=api_key, params=params, ) print(result)
get_wallet_token_balances()
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "address": "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d", "chain": "eth", } result = evm_api.token.get_wallet_token_balances( api_key=api_key, params=params, ) print(result)
get_wallet_token_transfers()
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "address": "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599", "chain": "eth" } result = evm_api.token.get_wallet_token_transfers( api_key=api_key, params=params, ) print(result)
Getting crypto data with the Moralis Web3 Data API and Python has never been easier. So, remember to sign up with Moralis and start leveraging the power of the Web3 industry today! By familiarizing yourself with this programming interface, you can build Web3-based Python projects in a heartbeat.
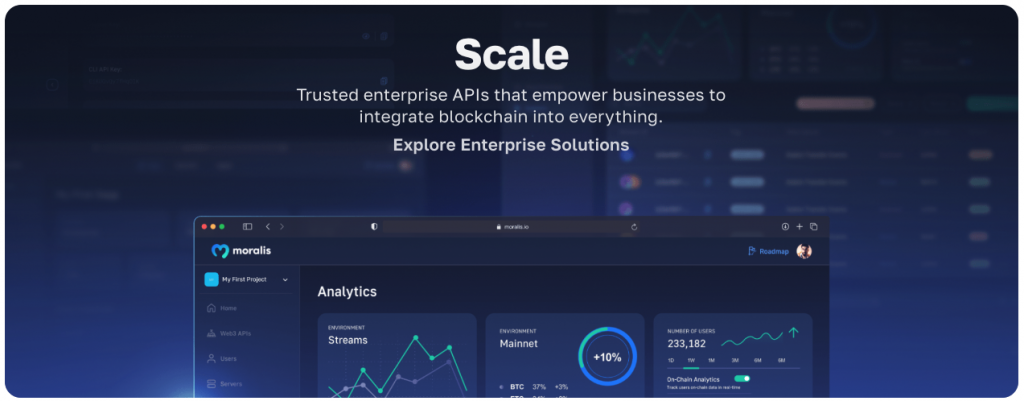
Overview
Today’s article will explore the Python-compatible Web3 Data API from Moralis and how to use this interface for cryptocurrency development. We will demonstrate the accessibility of the Web3 Data API by showing you how to use this tool to query blockchain data with Python. More specifically, we will cover the following five examples:
- How to pull cryptocurrency prices
- How to get historical ERC-20 token prices
- How to query wallet token balances
- How to get wallet token transfers
- How to query ERC-20 metadata by contract
If this sounds exciting and you want to jump straight into the coding part, click here to immediately navigate to the ”Python API for Cryptocurrency – Get Crypto Data” section!
In addition to the main tutorial, we will initiate the article by answering the question, ”is there a Python API to pull cryptocurrency prices, balances, and other blockchain data?”. In doing so, we will briefly explore the intricacies of the Python-compatible Web3 Data API and what it entails. Along with the Web3 Data API, Moralis also features another Python-compatible API: the Web3 Streams API. With this interface, you can effortlessly set up your own stream to monitor any blockchain event. If you want to learn how to use this API with Python to monitor cryptocurrency events, you are in luck, as we will cover just that in the ”Using the Moralis Streams API with Python” section!
Lastly, in the article’s final section, we will explore Python in a Web3 context and how Moralis can further elevate your blockchain development game. After all, what you will learn in this article only scratches the surface of what is possible with Moralis!
Also, remember to sign up with Moralis immediately, as you need an account to make calls to the various APIs!
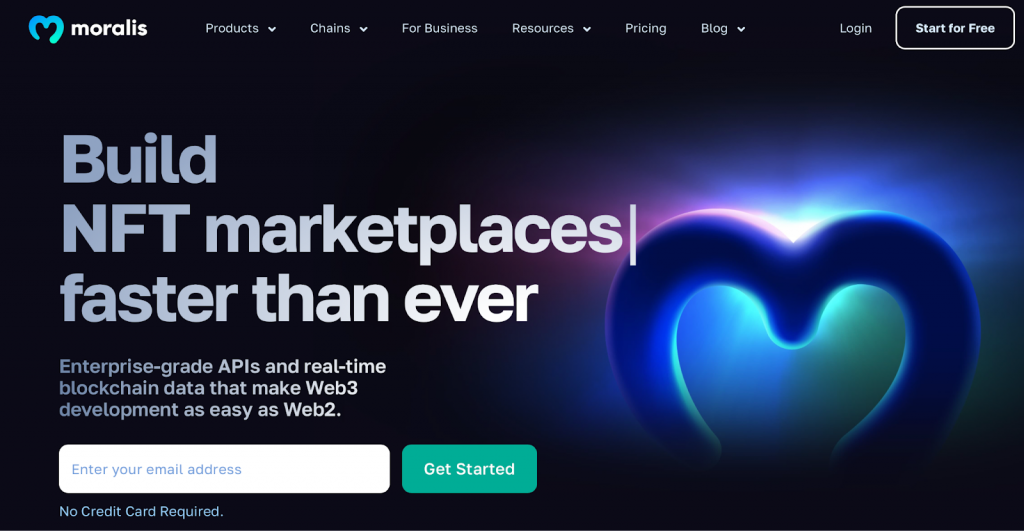
Is There a Python API to Pull Cryptocurrency Prices?
So, is there a Python API to pull cryptocurrency prices, balances, and other blockchain data? If you’re getting into Web3 Python development, you’ll be happy to hear that the answer to the aforementioned question is a resounding yes!
The Moralis Web3 Data API suite is fully Python-compatible. This means you can utilize the Python SDK to effortlessly make API calls to any of the Moralis endpoints. Consequently, you can seamlessly use the Web3 Data API and Python to seamlessly pull cryptocurrency prices, get all ERC-20 tokens owned by an address, query transfers by wallet, and much more!
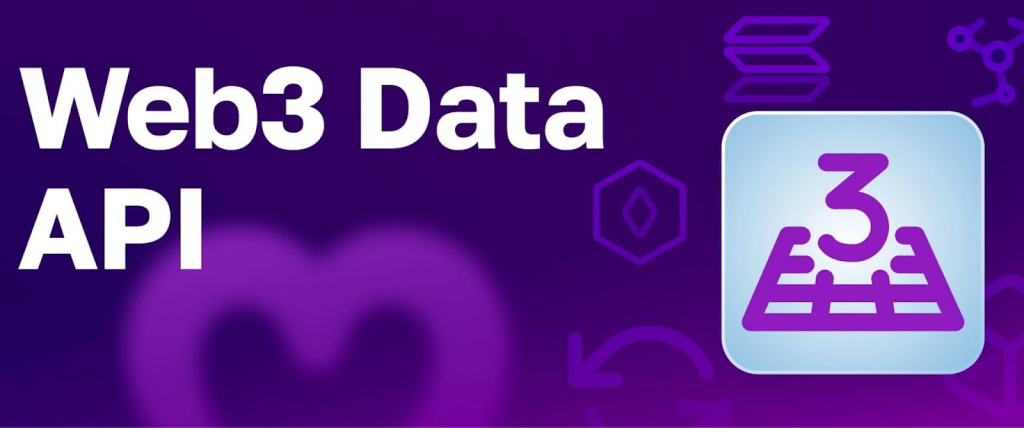
Furthermore, the Web3 Data API from Moralis is chain-agnostic, meaning that the interface supports a variety of different blockchain networks. This includes EVM-compatible chains such as Ethereum, Polygon, BNB Chain, and other networks like Aptos and Solana. As such, you can seamlessly use Moralis APIs and Python to, for instance, pull cryptocurrency prices across multiple different blockchain networks.
For example, if you are interested in Solana development, you might find our article exploring the Solana Python API interesting!
So, how exactly does this work? If you want the answer to this question, join us in the next section as we explore how to get crypto data using the Moralis Data API and Python!
Python API for Cryptocurrency – Get Crypto Data
In the following sections, we will dive deeper into how you can use the Web3 API to get cryptocurrency data with Python. In doing so, we will explore five prominent examples:
- How to pull cryptocurrency prices
- How to get historical ERC-20 token prices
- How to query wallet token balances
- How to get wallet token transfers
- How to query ERC-20 metadata by contract
However, before jumping straight into the first example showing you how to pull cryptocurrency prices, we must take care of a few prerequisites. These prerequisites are universal for each of our examples. So, make sure to cover them before continuing!
Moralis Web3 Data API with Python – Prerequisites
Before you progress, make sure to have the following ready:
- Install Python and PIP – Download and install Python and PIP.
- Set Up Moralis – Sign up with Moralis and acquire your Web3 API key. Once you register, you can find your key by logging in and navigating to the ”Web3 APIs” tab:
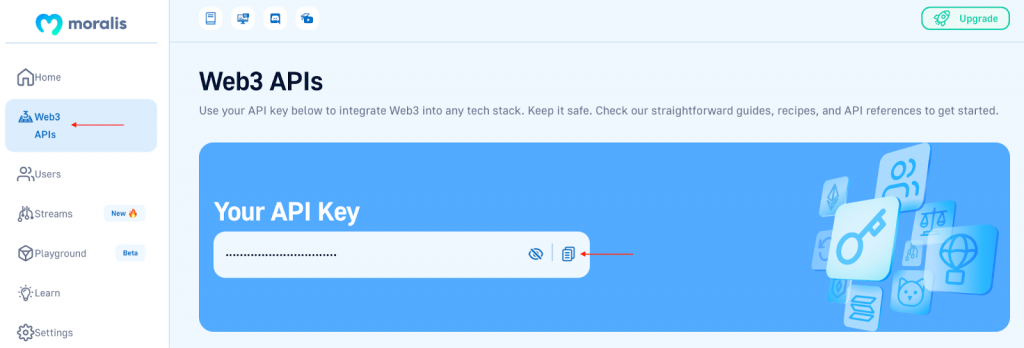
- Create a Python Project – Create a new Python project with an ”index.py” file. Install the Moralis SDK by opening a new terminal and running the following command in the project’s root folder:
pip install moralis
With the prerequisites covered, you are now ready to explore the various Moralis Web3 Data API endpoint. So, let us start by covering how you can pull cryptocurrency prices with Python!
How to Pull Cryptocurrency Prices
When using the Moralis Web3 Data API and Python, all you need to pull cryptocurrency prices of ERC-20 tokens is a single call to the get_token_price()
endpoint. So, open the ”index.py” file you created in the previous section and add the following code snippet:
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "address": "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599", "chain": "eth" } result = evm_api.token.get_token_price( api_key=api_key, params=params, ) print(result)
From here, you need to make a few minor configurations to the code. First, replace YOUR_API_KEY
with the Moralis API key you fetched when taking care of the prerequisites. Next, specify the chain
and address
parameters to fit your preferences.
That’s it; you should now be able to run the script using the following command:
python index.py
In return, you should find yourself with a response looking something like this:
{ "nativePrice": { "value": "13753134139373781549", "decimals": 18, "name": "Ether", "symbol": "ETH" }, "usdPrice": 16115.165641767926, "exchangeAddress": "0x1f98431c8ad98523631ae4a59f267346ea31f984", "exchangeName": "Uniswap v3" }
Congratulations! It is as easy as that to pull cryptocurrency prices when working with the Moralis Data API and Python!
Get Historical ERC-20 Token Prices
Next, we will look closer at how you can query historical ERC-20 token prices with the Web3 Data API and Python. To do so, we will use the same get_token_price()
endpoint; however, this time, you need to specify a range and query one block at a time through a for
loop. As such, you can go ahead and input the following into the ”index.py” file:
from moralis import evm_api api_key = "YOUR_API_KEY" historicalPrice = [] for to_block in range(16323500, 16323550, 10): params = { "address": "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599", "chain": "eth", "to_block": to_block } result = evm_api.token.get_token_price( api_key=api_key, params=params, ) historicalPrice.append(result) print(historicalPrice)
This time, you need to replace YOUR_API_KEY
with your Moralis key and specify the chain
and address
parameters, along with your preferred range. Once you are done with these configurations, you can once again run the script with the following terminal input:
python index.py
In return, you get a response containing an array of different prices from various points in time. Here is an example containing two prices:
[ { "nativePrice": { "value": "642828540698243", "decimals": 18, "name": "Ether", "symbol": "ETH" }, "usdPrice": 0.7811524052648599, "exchangeAddress": "0x1f98431c8ad98523631ae4a59f267346ea31f984", "exchangeName": "Uniswap v3" }, { "nativePrice": { "value": "642828540698243", "decimals": 18, "name": "Ether", "symbol": "ETH" }, "usdPrice": 0.7811524052648599, "exchangeAddress": "0x1f98431c8ad98523631ae4a59f267346ea31f984", "exchangeName": "Uniswap v3" }, ]
How to Query Wallet Token Balances
For the third example, we will show you how to query wallet token balances. To get this information, we will make an API call to the get_wallet_token_balances()
endpoint. As such, copy the snippet below and input the code into your ”index.py” file:
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "address": "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d", "chain": "eth", } result = evm_api.token.get_wallet_token_balances( api_key=api_key, params=params, ) print(result)
You can follow the same pattern here and add your Moralis API key by replacing YOUR_API_KEY
. Next, modify the address
and chain
parameters to fit your development needs. From there, all that remains is running the code with the same terminal input:
python index.py
In return, this is what the response should look like:
[ { "token_address": "0xefd6c64533602ac55ab64442307f6fe2c9307305", "name": "APE", "symbol": "APE", "logo": null, "thumbnail": null, "decimals": 18, "balance": "101715701444169451516503179" }, { "token_address": "0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2", "name": "Wrapped Ether", "symbol": "WETH", "logo": "https://cdn.moralis.io/eth/0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2.webp", "thumbnail": "https://cdn.moralis.io/eth/0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2_thumb.webp", "decimals": 18, "balance": "85000000000000000" } ]
How to Get Wallet Token Transfers
Next, let us show you how to get wallet token transfers. Getting this data is simple when working with the Moralis Web3 Data API and Python. In fact, all you need is a single API call to the get_wallet_token_transfers()
endpoint:
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "address": "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599", "chain": "eth" } result = evm_api.token.get_wallet_token_transfers( api_key=api_key, params=params, ) print(result)
Once you add the snippet above to your ”index.py” file, you must alter the code by adding your API key and configuring the parameters to fit your needs. You can then run the script with the command below:
python index.py
When you run the code, you should find yourself with a response similar to the one shown below in your terminal:
{ "total": 126, "page": 0, "page_size": 100, "cursor": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJhZGRyZXNzIjoiMHgyMjYwZmFjNWU1NTQyYTc3M2FhNDRmYmNmZWRmN2MxOTNiYzJjNTk5IiwiY2hhaW4iOiJldGgiLCJhcGlLZXlJZCI6MTkwNjU5LCJsaW1pdCI6MTAwLCJ0b3BpYzMiOiI9Om51bGwiLCJ0b19ibG9jayI6IjExMTAwMDQ1IiwicGFnZSI6MSwidG90YWwiOjEyNiwib2Zmc2V0IjoxLCJ1YyI6dHJ1ZSwiaWF0IjoxNjY5NjQ2ODMzfQ.NIWg35DjoTMlaE6JaoJld24p9zBgGL56Zp8PPzQnJk4", "result": [ { "transaction_hash": "0x1f1c8971dec959d38bcaa5606eb474d028617752240727692cd5ef21a435d847", "address": "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599", "block_timestamp": "2022-11-19T20:01:23.000Z", "block_number": "16006313", "block_hash": "0x415f96c01f32d38046e250a357e471000c0876cc2122167056cf4c4c1113a522", "to_address": "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599", "from_address": "0xa9d1e08c7793af67e9d92fe308d5697fb81d3e43", "value": "4489517", "transaction_index": 48, "log_index": 66 } ] }
How to Query ERC-20 Metadata by Contract
For our last example of how you can use the Moralis Web3 Data API with Python, we will show you how to query ERC-20 metadata based on a contract address. To get this data, Moralis provides you with the get_token_metadata()
endpoint. To call this endpoint, enter the following code into your ”index.py” file:
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "addresses": ["0x1f9840a85d5af5bf1d1762f925bdaddc4201f984"], "chain": "eth" } result = evm_api.token.get_token_metadata( api_key=api_key, params=params, ) print(result)
Next, swap out YOUR_API_KEY
for your Moralis Web3 API key. From there, obtain the contract address you want to query and replace the parameters accordingly. You can then run the script by inputting python index.py
into your terminal and hitting enter.
As a result, you should receive a JSON response similar to this:
[ { "address": "0x1f9840a85d5af5bf1d1762f925bdaddc4201f984", "name": "Uniswap", "symbol": "UNI", "decimals": "18", "logo": "https://cdn.moralis.io/eth/0x1f9840a85d5af5bf1d1762f925bdaddc4201f984.webp", "logo_hash": "064ee9557deba73c1a31014a60f4c081284636b785373d4ccdd1b3440df11f43", "thumbnail": "https://cdn.moralis.io/eth/0x1f9840a85d5af5bf1d1762f925bdaddc4201f984_thumb.webp", "block_number": null, "validated": null, "created_at": "2022-01-20T10:39:55.818Z" } ]
Using the Moralis Streams API with Python
In addition to querying data, Moralis features another enterprise-grade, Python-compatible Web3 API for monitoring on-chain events: Moralis Streams. With this industry-leading interface, you can seamlessly set up your own streams to monitor blockchain events based on your filters!
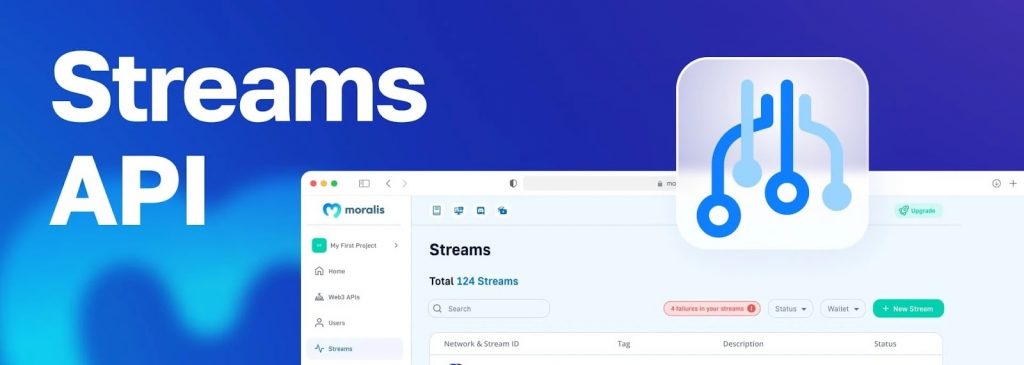
To learn more about the Moralis Streams API and how to monitor blockchain events using Python, check out the Moralis YouTube video below. In this clip, you initially get to explore the differences between working with Moralis Streams vs Web3 Py. At ”06:45”, our talented software engineer starts the Moralis Streams API Python tutorial, showing you how to monitor USDT transactions effortlessly:
You can also find the code used in the video above by checking out the following GitHub repository:
Complete Moralis Streams API Python Repository – https://github.com/MoralisWeb3/youtube-tutorials/tree/main/moralis-streams-vs-web3-py
Exploring Python for Cryptocurrency Development
There are many similarities between conventional Web2 and Web3 Python development. However, if you are new to the blockchain space, there are a few minor differences for you to consider. For instance, mastering libraries such as Web3.py can be highly beneficial. This is a library making it easier to interact with the Ethereum blockchain.
What’s more, there are additional development tools, such as Web3 infrastructure providers like Moralis, that can make your life as a Web3 Python developer easier. Moralis is an industry-leading Web3 infrastructure provider, supplying Python-compatible tools like Web3 APIs, SDKs, and more.
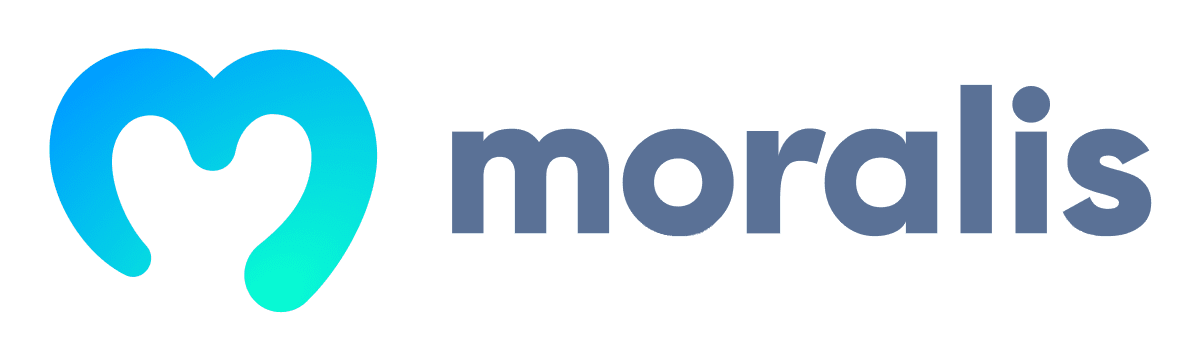
If you have followed along this far, you have now familiarized yourself with the Web3 Data API and Streams API. However, in doing so, we’ve only scratched the surface of what is possible with these interfaces, and there are various other endpoints and use cases to discover!
With these development resources, you can effortlessly start building more complex projects. If this is your ambition, check out either of our articles on how to create a DAO or how to build a Web3 Amazon clone!
So, if you are interested in taking your Web3 projects to the next step, remember to sign up with Moralis. In doing so, you can start using the full power of the blockchain industry and scale your projects effortlessly!
Summary – Best Python API for Cryptocurrency
In this tutorial, we taught you how to use the Python-compatible Web3 Data API to pull cryptocurrency prices, balances, and other blockchain data. More specifically, we covered the following five examples:
- How to pull cryptocurrency prices
- How to get historical ERC-20 token prices
- How to query wallet token balances
- How to get wallet token transfers
- How to query ERC-20 metadata by contract
If you have followed along this far, you now know how to use a Python API for cryptocurrency development. From here, it is now up to you to be creative and start building more sophisticated projects using the Web3 Data API!
If you found this tutorial helpful, you might also want to check out other development content here on the Web3 blog. For instance, learn how to create an ERC-721 NFT, explore the Arbitrum testnet, or get tokens using an Arbitrum Goerli faucet. In addition, if you want to take your Web3 development skills to the next level, make sure to check out Moralis Academy. There, you can find courses aimed at beginners and more experienced Web3 devs. For example, take the Ethereum Smart Contract Programming 201 course – perfect for anyone who wants to develop a career as a smart contract developer!
Remember to sign up with Moralis right now. Creating an account is entirely free, and you can start leveraging Moralis’ industry-leading APIs and the full power of blockchain technology in a heartbeat!