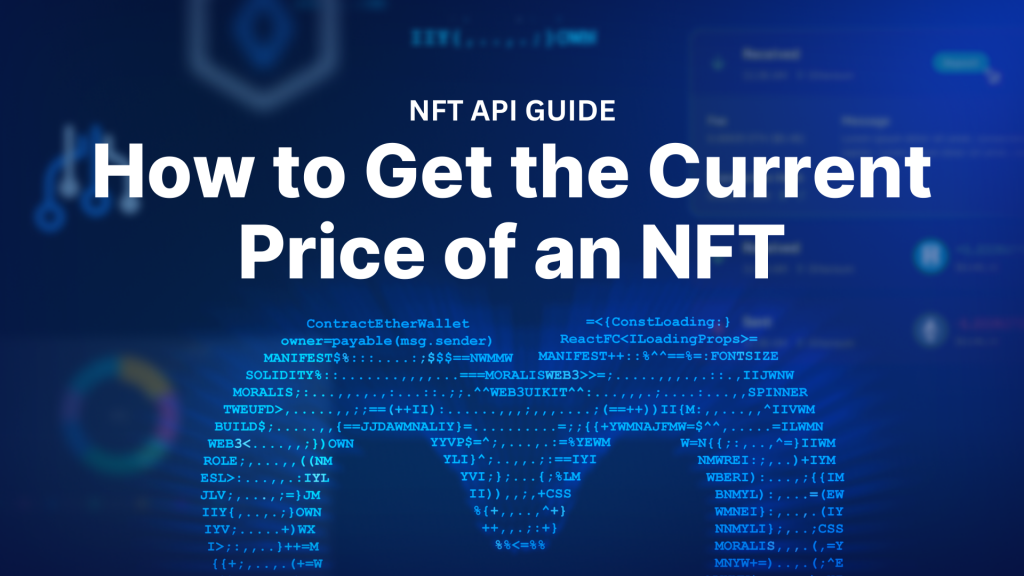
In this guide, we demonstrate how to get the current price of an NFT using the industry-leading NFT API from Moralis! We will also get the price of an NFT using the Market Data API and two of its endpoints. Thanks to the simplicity of Moralis’ powerful API suite, you can simply use single lines of code to accomplish this. So, if you’ve been looking for an answer to the “how to get current price of NFT” query, look no further!
- You can get the current price of an NFT when getting the top NFT collections by market cap using the
getTopNFTCollectionsByMarketCap()
endpoint:
const response = await Moralis.EvmApi.marketData.getTopNFTCollectionsByMarketCap();
- Get the current price of an NFT when getting top NFT collections by trading volume using the
getHottestNFTCollectionsByTradingVolume()
endpoint:
const response = await Moralis.EvmApi.marketData.getHottestNFTCollectionsByTradingVolume();
These two endpoints provide the following details:
- Rank of the collection
- Collection title
- Collection image
- Floor price in USD
- Floor price 24hr percent change
- Market cap in USD
- Market cap 24hr percent change
- Volume in USD
- Volume 24hr percent change
Another useful endpoint you will benefit from comes from the NFT API and its getNFTLowestPrice()
endpoint, enabling you to get an NFTs lowest price:
const response = await Moralis.EvmApi.nft.getNFTLowestPrice();
The above code snippets are all you need to give your dapps the power to access the current prices of NFTs. If you’ve used Moralis before, you already know exactly how to implement the above endpoint to get the most out of them. However, if you are new to this ultimate Web3 API provider, make sure to cover this guide from start to finish. Not only will it give you an answer to the query of how to “get current price of NFT“, but it will also help you to get going with Moralis. Plus, we’ll ensure you learn more about other powerful NFT API endpoints that can save you a ton of time and money.
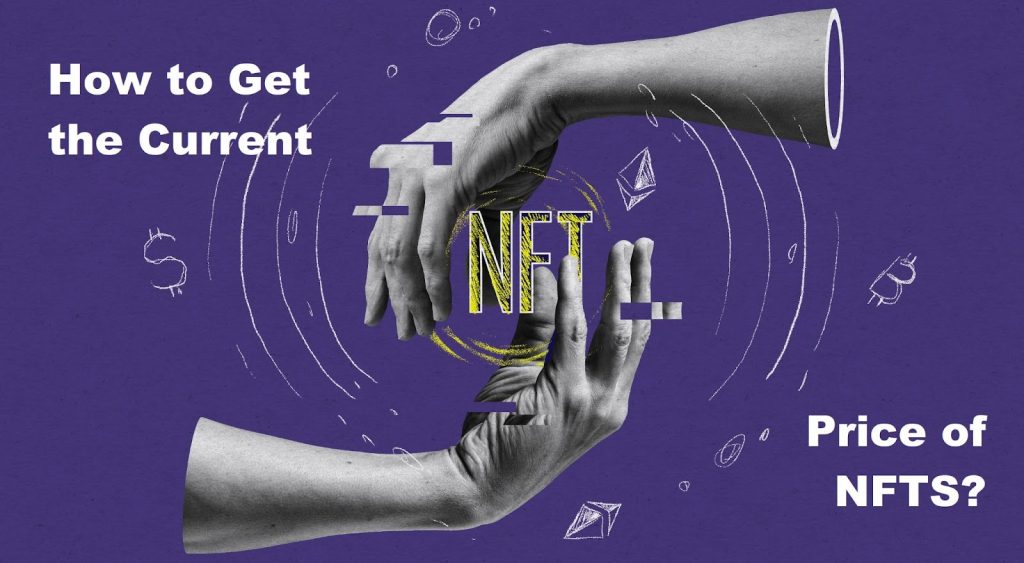
Overview
The “how to get current price of NFT” query is becoming increasingly popular. Therefore, we decided to provide the endpoints that allow developers to get the current NFT(s) price. Since showing you how to get the current price of a non-fungible token is the main point of this guide, we’ll dive straight into our simple tutorial. The tutorial will first cover the prerequisites required to use the endpoints highlighted at the outset of this guide. Then, the tutorial will lay out two simple steps to fetch the necessary data. You’ll soon see that implementing these endpoints is as straightforward as it gets!
Next, we’ll ensure you get acquainted with the getTopNFTCollectionsByMarketCap()
and getHottestNFTCollectionsByTradingVolume()
endpoints. And for those not ready to use the Pro or Business plan – which is required for the previous endpoints – we’ll also present an alternative option.
We’ll also cover some popular use cases for our highlighted “current NFT price” endpoints. This may spark some valuable ideas on how you can make the most of Moralis. In the final part of this article, we’ll move beyond teaching you how to get the current price of NFTs. After all, there are many other pieces of on-chain data regarding NFTs that you can put to good use. Hence, we’ll do a proper overview of the Moralis NFT API.
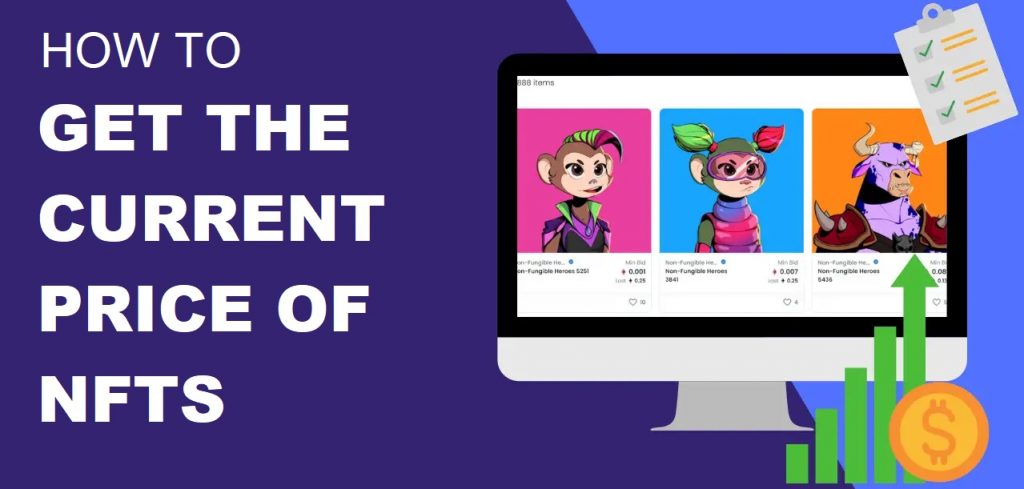
Tutorial: How to Get the Current Price of an NFT with Moralis
The process of implementing the getTopNFTCollectionsByMarketCap()
and getHottestNFTCollectionsByTradingVolume()
endpoints is more or less the same. In fact, the implementation steps are similar when using any of Moralis’ Web3 APIs. Therefore, we will guide you through the exact steps for only one of the two endpoints.
Prerequisites
In order to use any of the Moralis Web3 APIs, you need to set things up properly. Fortunately, the setup process is straightforward and only requires classic programming tools:
Programming Language:
- NodeJS (v14+)
- Python
Package Manager:
- NPM/Yarn or Pip
You will also need your Moralis account in order to access your Moralis API key.
While you can access most of Moralis’ API endpoints with a free account, that is not the case for getTopNFTCollectionsByMarketCap()
and getHottestNFTCollectionsByTradingVolume()
. So, make sure to also upgrade to the Pro or Business plan.
For a more detailed guide on how to cover these prerequisites, check out the Moralis Getting Started documentation page. Under the Getting Started section, you can also find quickstart guides for specific frameworks:
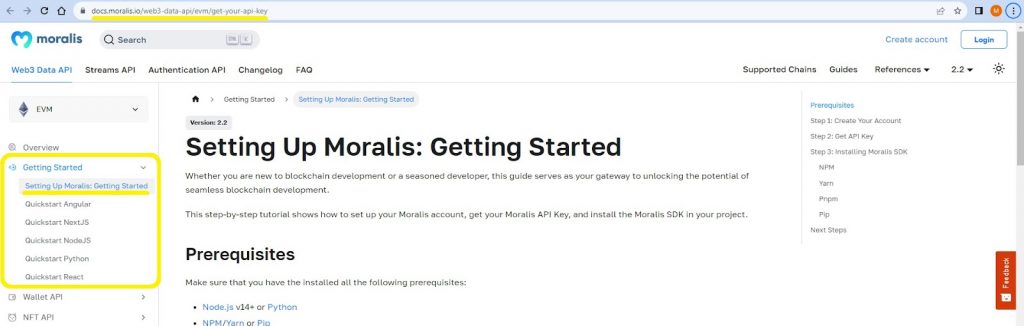
Step 1: Implement the Right Endpoint
Now, you have to decide which of the two “get NFT price” endpoints you want to use. For the sake of this tutorial, we’ll go with getHottestNFTCollectionsByTradingVolume()
.
The script running the required endpoint must first import Moralis and EvmChain
. Below these import
lines, you get to run your app and initiate Moralis. Furthermore, you must also enter your Moralis API key.
Aside from your API key, the runApp
async function also includes the endpoint.
The exact lines of code vary depending on the framework you use; however, you can find the scripts that will do the trick below. So, copy the appropriate script and replace the YOUR_API_KEY
placeholder with your Moralis API key. Here are some examples of the scripts showcasing “how to get current price of NFT”:
- JavaScript:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const response = await Moralis.EvmApi.marketData.getHottestNFTCollectionsByTradingVolume(); console.log(response.raw); }; runApp();
- TypeScript:
import Moralis from "moralis"; import { EvmChain } from "@moralisweb3/common-evm-utils"; const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const response = await Moralis.EvmApi.marketData.getHottestNFTCollectionsByTradingVolume(); console.log(response.raw); }; runApp();
- Python:
from moralis import evm_api import json api_key = "YOUR_API_KEY" result = evm_api.market_data.get_hottest_nft_collections_by_trading_volume( api_key=api_key, ) print(result)
Step 2: Run Your Script
Now that you have one of the above example scripts in place, it’s time to run the script. As such, enter one of the following commands that matches your choice:
- JavaScript:
node index.js
- TypeScript:
ts-node index.ts
- Python:
python index.py
As soon as you run your script, you’ll be able to see a JSON response in your terminal. Here’s an example response:
[ { "rank": 1, "collection_title": "Bored Ape Yacht Club", "collection_image": "https://market-data-images.s3.us-east-1.amazonaws.com/tokenImages/0x2f1ef58880d01489b8d2c9ba759cf7b14db4ee8a55fd6e6b222ad050e7e00fb0.png", "volume_usd": "8261189.224011", "volume_24hr_perecent_change": "17.09", "floor_price_usd": "79591.5288", "floor_price_24hr_percent_change": "0.05", "average_price_usd": "39717.255884668266" }, { "rank": 2, "collection_title": "Mutant Ape Yacht Club", "collection_image": "https://market-data-images.s3.us-east-1.amazonaws.com/tokenImages/0xab57ba0f4ce4424e60c477627f6551790b8946d45720240a3d258956e436f2e5.png", "volume_usd": "3374077.733755", "volume_24hr_perecent_change": "6.29", "floor_price_usd": "16028.433180000002", "floor_price_24hr_percent_change": "-0.69", "average_price_usd": "15915.461008278302" }, { "rank": 3, "collection_title": "CryptoPunks", "collection_image": "https://market-data-images.s3.us-east-1.amazonaws.com/tokenImages/0x8e4d149625faffbdb3b63eb36668a4b470714c0e6c765e66200095a4ccb0234e.png", "volume_usd": "1835336.891", "volume_24hr_perecent_change": "30.13", "floor_price_usd": "90913.539", "floor_price_24hr_percent_change": "3.43", "average_price_usd": "101963.16061111112" } ]
The example response above shows that the floor price is just one of many useful market data insights. The same is true for the getTopNFTCollectionsByMarketCap()
endpoint.
Get NFT Price Endpoints
After covering the above tutorial, you already know that getHottestNFTCollectionsByTradingVolume()
and getTopNFTCollectionsByMarketCap()
answer the query of “how to get current price of NFT”. And, while you can learn all you need to know about these endpoints by running them with your scripts and analyzing their responses, you can also explore them in advance using their respective API reference pages:
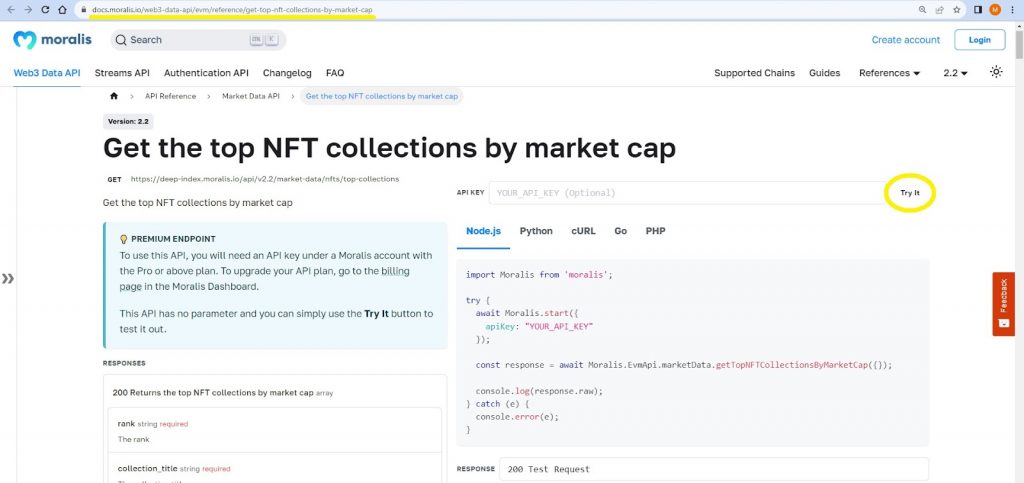
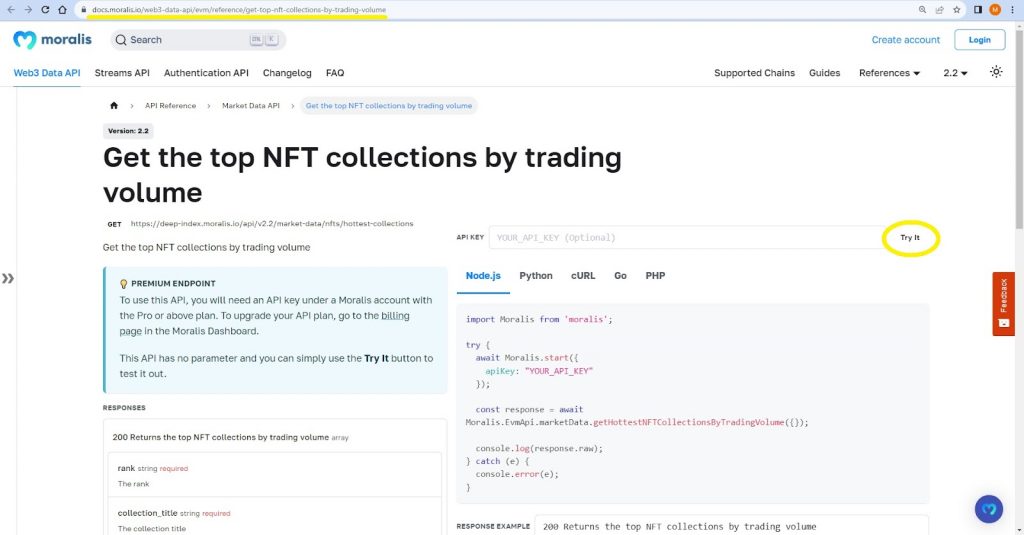
As indicated in the above two screenshots, both reference pages allow you to try the endpoints. Nonetheless, these are the details that the endpoints offer:
- Rank of the collection
- Collection title
- Collection image
- Floor price in USD
- Floor price 24hr percent change
- Market cap in USD
- Market cap 24hr percent change
- Volume in USD
- Volume 24hr percent change
Feel free to visit the above-linked reference pages and try running the scripts for different dev frameworks.
How to Get the Current Price of an NFT with an Alternative NFT API Endpoint
There is also an alternative API endpoint that you can access with a free Moralis account. To that end, we are presenting you with the NFT API’s getNFTLowestPrice()
endpoint. It lets you fetch the lowest executed price for an NFT contract for the last X days for transactions paid in ETH.
Unlike the above two endpoints, this one takes in some required and optional parameters. The required parameter comes in the form of the NFT contract address that you wish to focus on. By default, the endpoint focuses on the Ethereum network, but you can use it for other supported chains by adding a proper “chain to query” parameter.
The getNFTLowestPrice()
endpoint also allows you to enter the number of days to look back to find the lowest price. The default option is seven days. Plus, the marketplace
parameter enables you to determine which NFT marketplace you want to focus on.
To give this endpoint a try, feel free to visit its API reference page. If you need help implementing it, check out the “How to get lowest price of an NFT by Marketplace” documentation page. However, using this endpoint is the same as for any other Moralis API endpoint.
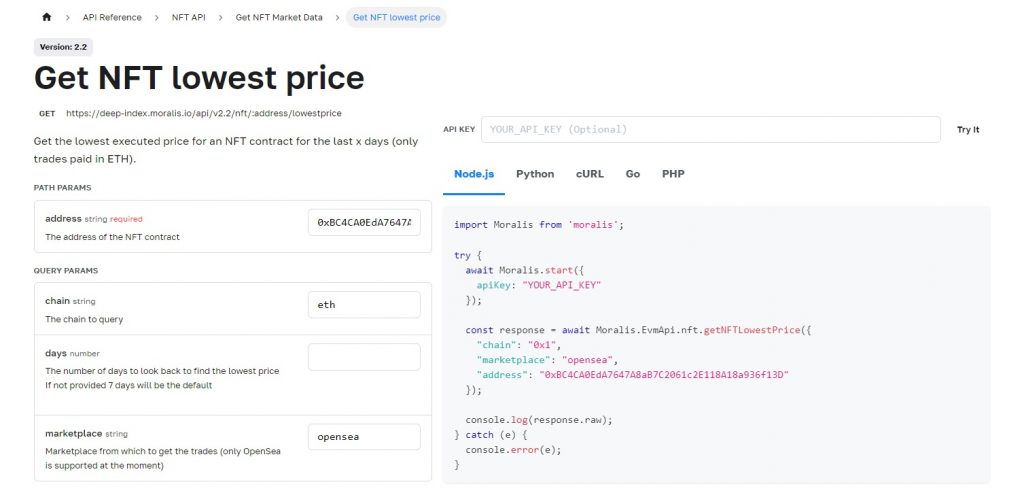
Use Cases for Current NFT Price
As you can imagine, many different use cases exist for the above-outlined API endpoints. After all, users interested in NFTs often want to know currency prices. So, you can put the above-offered details to good use by creating all sorts of dapps that incorporate NFTs.
However, the most typical use case that requires NFT prices comes in the form of an NFT marketplace. And if you wish to build such a dapp, we encourage you to check out our NFT marketplace development guide before moving any further.
Aside from NFT marketplaces, any dapp that supports NFT can benefit from the ability to also display a token’s price. For example, even if you are building an eye-catching NFT gallery, you could offer users price insights as they hover over the NFT images.
All in all, these are the most popular use cases for the “current NFT price” endpoints and the entire NFT API fleet:
- NFT marketplaces
- NFT auction sites
- Portfolio apps
- NFT-based authentication
Before we move on, it’s worth mentioning that aside from getting NFT prices, Moralis also allows you to fetch cryptocurrency prices. If that interests you, check out the Price API.
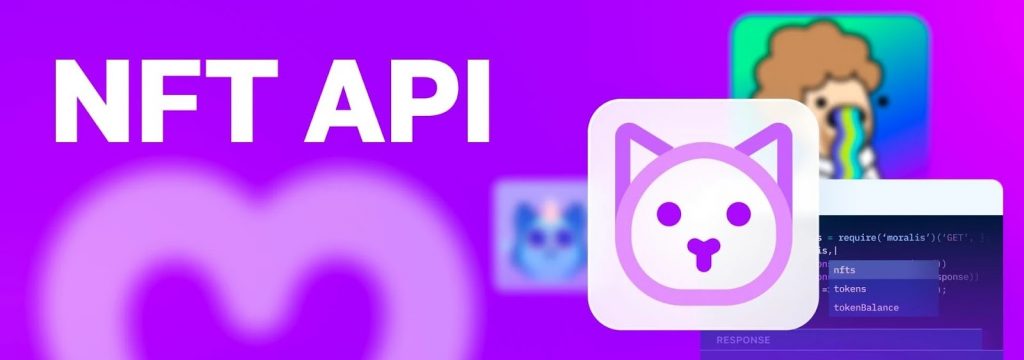
Going Beyond the Current Price of an NFT – Meet the Most Powerful NFT API
Beyond giving developers easy access to current NFT prices, the Moralis NFT API includes many other powerful and useful features. For instance, you can easily detect verified NFT collections and empower users to see which NFT collections are verified by NFT marketplaces like OpenSea.
Another great example comes in the form of NFT spam protection. The latter allows you to protect your users from scams and ensure a much better user experience.
These are just two specific use cases of the ultimate NFT API that Moralis offers. After all, this ultimate tool for building dapps around NFTs provides multiple out-of-the-box solutions. It makes fetching NFT metadata, NFT collection metadata, NFT ownership details, NFT transfers and more extremely straightforward.
In addition, it supports ERC-721 and ERC-1155, as well as non-standard NFTs (such as CryptoPunks).
The core benefits of the Moralis NFT API include:
- Enriched Metadata – Access fully enriched and normalized metadata on NFT collections and individual tokens through a single API call.
- Real-Time NFT Transfer Data – Get all the latest NFT transfer data, whether you need transfer history for specific NFTs, wallets, or tracking real-time transfers.
- Instant NFT Ownership Data – Track NFT ownership across the blockchain with just one API call, enabling token-gated content and enhanced creator control.
- Advanced Spam Detection – Protect your platform from undesirable NFTs with collection spam indicators.
- On-Chain Pricing Data – Incorporate on-chain pricing data within your dapps, such as last sale prices and lowest “sold for” prices.
- Optimized Image Previews – Benefit from dynamically sized image previews and image conversions to user-friendly formats.
- Lightning-Fast Speed – Experience unmatched performance for fetching NFTs powered by Moralis’ blazing-fast CDNs.
- Alerts and Notifications – Plug and play with our Streams API to get real notifications on NFT trades, NFTs mints, and marketplace orders.
Why Use Moralis’ NFT API
The core and most obvious reason why you should use the NFT API from Moralis lies in the above-listed features. After all, they allow you to create killer NFT dapps without reinventing the wheel. As such, you can focus more of your time and resources on delivering the best possible user experience (UX).
The same applies to the rest of Moralis’ Web3 API fleet. This is also why several of the leading Web3 companies use them. Moreover, accessing the same backend power as the leading Web3 project is as good of a “WHY” as it gets!
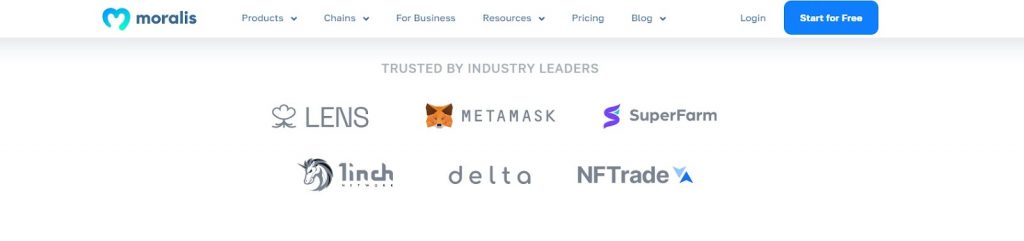
Moralis offers several case studies that further prove the magnitude of its impact. For instance, Unizen saved $200,000 by plugging in Moralis APIs. Another example is Drecom, which saved five months of dev time with Moralis. If you wish to explore these case studies, use the “NFT API” link at the top of this article.
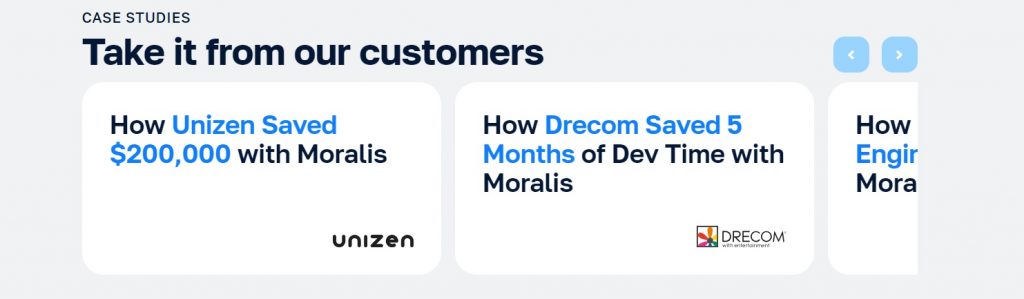
Are you ready to build the next big thing? Dive into the Moralis NFT API documentation and start BUIDLing!
How to Get the Current Price of an NFT – Summary
In today’s guide, you learned how to get the current price of an NFT without breaking a sweat. You now know that to do this, you can deploy the power of Moralis’ Market Data API. After all, the latter offers the getTopNFTCollectionsByMarketCap()
and getHottestNFTCollectionsByTradingVolume()
endpoints that will get the job done. Plus, you also learned how to implement these two endpoints in minutes using classic dev tools.
Another alternative addressing the “how to get current price of NFT” query comes from the NFT API’s getNFTLowestPrice()
endpoint. Unlike the other two outlined endpoints, this one allows you to focus on one specific NFT address at the time. You can access it with your free Moralis account!
You also learned about the different use cases for the ultimate NFT API. So, make sure to use Moralis’ powerful tools to create the next big thing with time and resources to spare. However, if you’d like to build up your Web3 knowledge and increase your confidence before developing, explore the Moralis blog. You may even wish to take a more professional approach to your crypto education with Moralis Academy.