Today’s tutorial demonstrates how to get the ERC20 token balance of an address with the Moralis Token API. Thanks to the accessibility of this tool, all you need is a single API call to the getWalletTokenBalances()
endpoint. Here’s an example of what it can look like:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const address = "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d"; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.token.getWalletTokenBalances({ address, chain, }); console.log(response.toJSON()); }; runApp();
All you have to do is replace YOUR_API_KEY
with your Moralis API key, configure the address
and chain
parameters to fit your request, and run the code. In return, you’ll get an array of tokens containing the balance for each:
[ { "token_address": "0x3c978fc9a42c80a127863d786d8883614b01b3cd", "symbol": "USDT", "name": "USDTOKEN", "logo": null, "thumbnail": null, "decimals": 18, "balance": "10000000000000000000000", "possible_spam": true }, { "token_address": "0xdac17f958d2ee523a2206206994597c13d831ec7", "symbol": "USDT", "name": "Tether USD", "logo": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7.png", "thumbnail": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7_thumb.png", "decimals": 6, "balance": "102847", "possible_spam": false }, //... ]
That’s it; when working with Moralis, it’s easy to get the ERC20 token balance of an address. From here, you can now use this data and effortlessly integrate it into your Web3 projects!
If you’d like to learn more about how this works, join us in this article or check out the official documentation on how to get ERC20 token balance by a wallet.
Also, remember to sign up with Moralis, as you’ll need an account to follow along in this tutorial. You can register entirely for free, and you’ll gain immediate access to the industry’s leading Web3 APIs!
Overview
An essential aspect of most blockchain applications – including wallets, decentralized exchanges (DEXs), portfolio trackers, and Web3 analytics tools – are ERC20 token balances. Consequently, no matter what decentralized application (dapp) you’re building, you’ll likely need the ability to effortlessly get the ERC20 token balance of an address. However, querying and processing this data without proper development tools is a tedious and time-consuming task. Fortunately, you can now avoid the challenging parts by leveraging the Moralis Token API to get the ERC20 token balance of an address in no time!
In today’s article, we’ll start by going back to basics and briefly examine what a token balance is. From there, we’re also going to explore ERC20, as this is the most popular standard for fungible tokens. Next, we’ll jump straight into the tutorial and show you how to seamlessly get the ERC20 token balance of an address using the Moralis Token API. To top things off, we’ll also show you how to use the Token API to get the spender allowance of an ERC20 token!
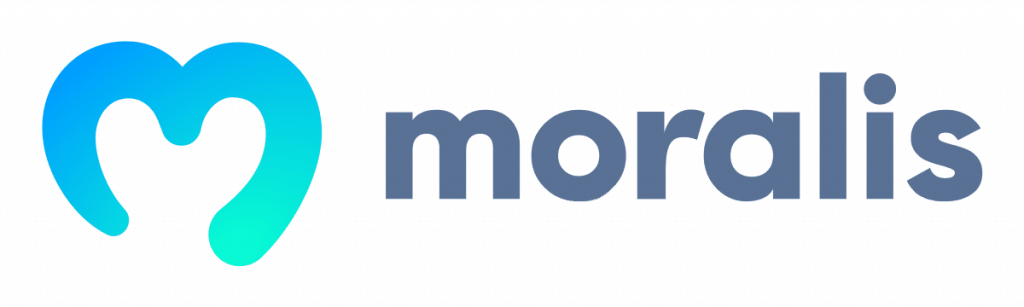
If you already know what a token balance is and what the ERC20 token standard entails, feel free to skip straight into the ”Tutorial: How to Get the ERC20 Token Balance of an Address” section!
Also, if you’re serious about building Web3 projects, you’ll likely want to check out some other tools Moralis offers besides the Token API. For instance, two other amazing interfaces are Moralis’ free NFT API and the free crypto Price API. With these tools, you can seamlessly build Web3 projects in a heartbeat!
So, if you want access to the industry’s leading Web3 APIs, remember to sign up with Moralis so you can start leveraging the true power of blockchain technology today!
What is a Token Balance?
A token balance refers to the amount of a particular cryptocurrency an account or wallet holds. In the crypto world, there are two primary fungible token types: native and non-native tokens. Let’s look at each a little bit deeper:
- Native Tokens: A native token is a blockchain network’s base cryptocurrency. All independent blockchains have their own native token. And they have a lot of utility within their respective ecosystems. For instance, a native token is often used to reward validators/miners and pay for transaction fees. A prominent example is ETH, which is the native token of the Ethereum network.
- Non-Native Tokens: Non-native tokens are created on top of an existing network – such as Ethereum – using smart contracts. These tokens are typically created by a Web3 project and are only used within its own borders. Examples of non-native tokens include DAI, USDT, and USDC.
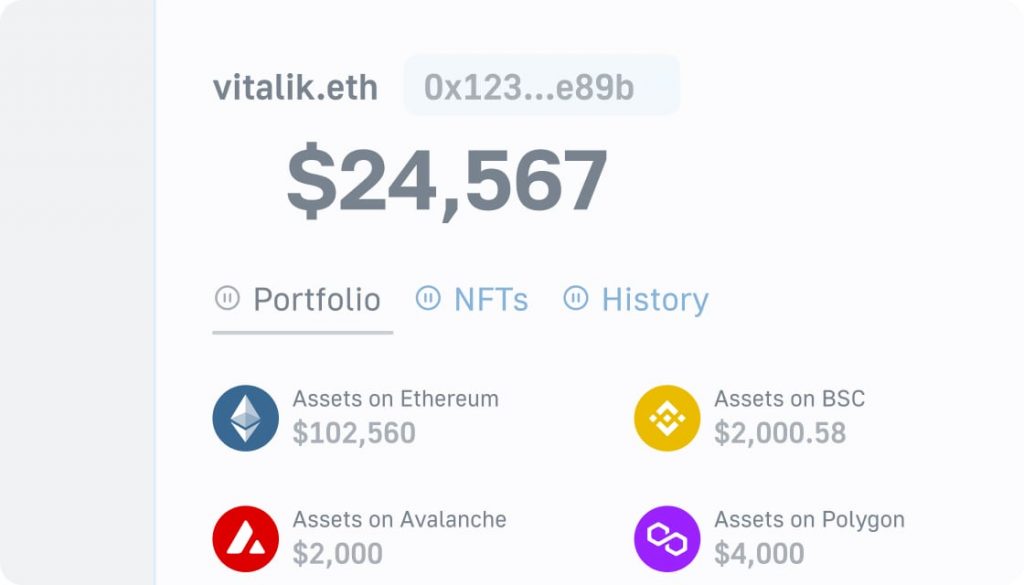
So, when talking about a token balance, we typically refer to the amount of a particular native or non-native token an account or wallet holds. Moreover, even though there are various types of fungible tokens, they mostly follow the ERC20 token standard. But what exactly does this mean? And how does ERC20 work?
What is ERC20?
ERC20, or Ethereum Request for Comment 20, is a technical standard for smart contracts on the Ethereum blockchain and other EVM-compatible networks. This standard defines a set of rules, functions, and events that a token must implement for it to be considered ERC-20 compliant.
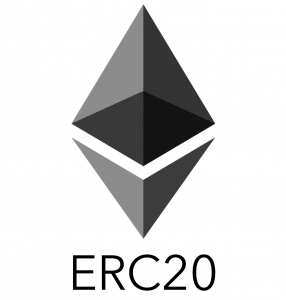
The functions and events outlined in the ERC20 token standard provide a common interface for all tokens so they can be easily accessed, recognized, and used. For instance, one of the functions is balanceOf()
, ensuring that it’s possible to seamlessly get a user’s balance of any ERC20 token.
Nevertheless, this standard interface ensures a unified ecosystem where all tokens are compatible with one another, along with decentralized applications (dapps) and other Web3 projects. Some prominent examples of ERC20 tokens include ETH, USDC, USDT, SHIB, and many others!
Also, while ERC20 is the most popular and well-used token standard, it’s far from the only one. Two additional standards are ERC721 and ERC1155, which are used to regulate NFTs on the Ethereum blockchain. If you’d like to learn more about these, check out our article on ERC721 vs. ERC1155!
Nevertheless, now that you have a better understanding of what ERC20 entails, let’s jump straight into the tutorial and show you how to get a wallet’s ERC20 token balance!
Tutorial: How to Get the ERC20 Token Balance of an Address
The best and most straightforward way to get the ERC20 token balance of a user is to leverage the Moralis Token API. This API provides real-time ERC20 token data, allowing you to fetch prices, transfers, and, of course, balances with a single line of code!
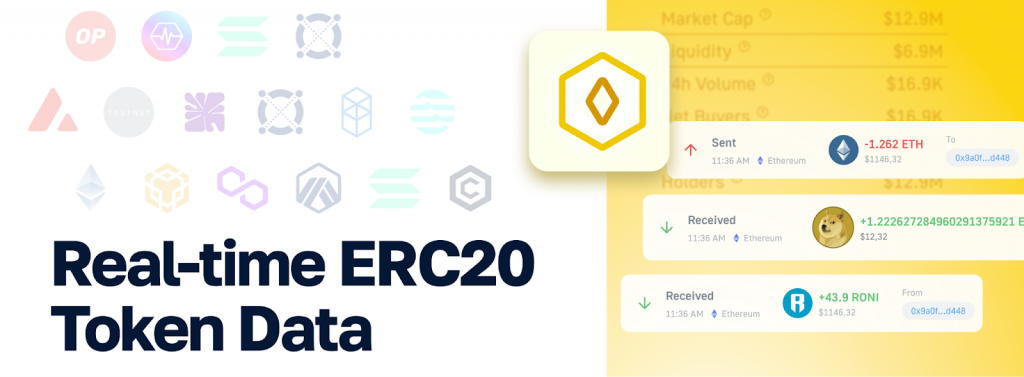
The API is also cross-chain compatible, supporting ten-plus EVM chains, including Ethereum, BNB Smart Chain, Polygon, and many others. As such, with Moralis, it has never been easier to build crypto wallets, portfolio trackers, or Web3 token analytics tools!
Nevertheless, to highlight the accessibility of this tool, we’ll take the following sections to show you how to get the ERC20 token balance of a user in three straightforward steps:
- Get an API Key
- Write a Script
- Run the Code
But before we jump into the first step of the tutorial, you’ll have to take care of a few prerequisites!
Prerequisites
In this tutorial, we’ll show how to get the ERC20 token balance of a user with JavaScript. However, you can also use TypeScript and Python if you prefer. But if this is the case, note that the steps outlined in this tutorial might differ slightly.
Nevertheless, before you get going, you’ll need to have the following ready:
- Node v.14+
- NPM/Yarn
From here, you need to install the Moralis SDK. To do so, set up a new project in your integrated development environment (IDE) and run the following terminal command in your project’s root folder:
npm install moralis @moralisweb3/common-evm-utils
That’s it; you’re now ready to jump into the first step of this tutorial on how to get the ERC20 token balance of a user!
Step 1: Get an API Key
You’ll initially need a Moralis API key, which is required for making calls to the Token API. Fortunately, you can get your API key for free. All you have to do is sign up with Moralis by clicking on the ”Start for Free” button at the top right of the Moralis homepage:
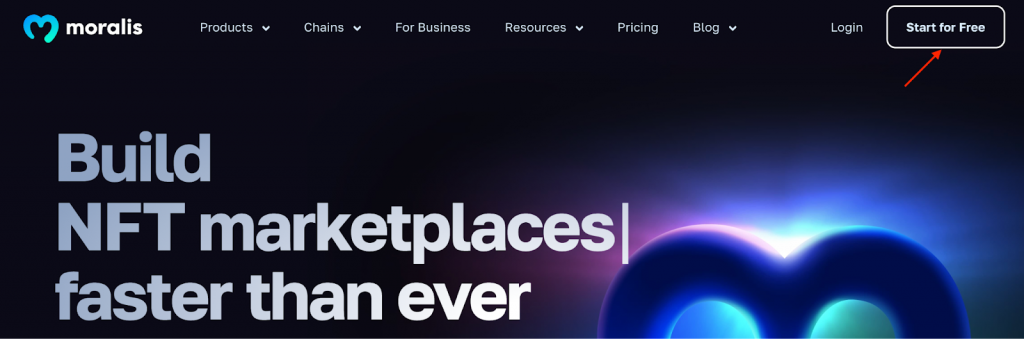
Once you’re done setting up an account and your first project, you can find the key by navigating to the ”Settings” tab. From there, you can simply scroll down and copy your Moralis API key:
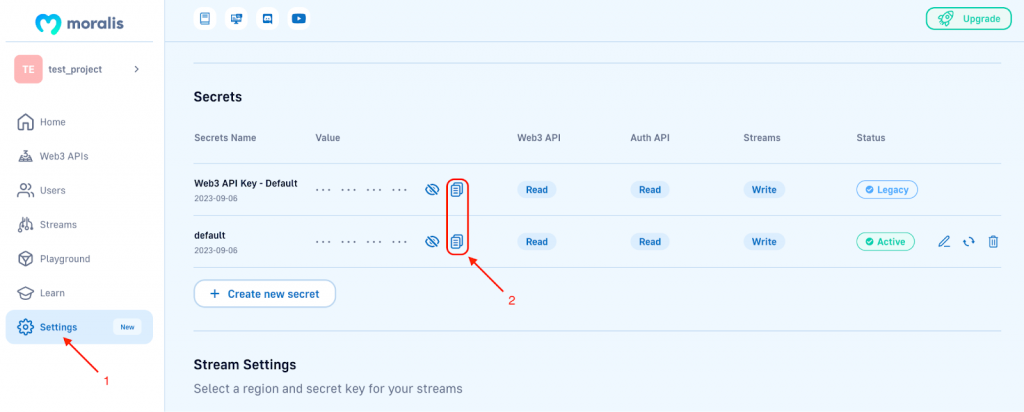
Save the key, as you’ll need it in the next step!
Step 2: Write a Script
With a Moralis API key at hand, you’re ready to start coding. As such, set up a new ”index.js” file in your project’s root folder and add the following code:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const address = "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d"; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.token.getWalletTokenBalances({ address, chain, }); console.log(response.toJSON()); }; runApp();
This is all the code you need to get the ERC20 token balance of a user. However, you need to make a few minor configurations before you can run the script.
First of all, replace YOUR_API_KEY
with the key you copied in the previous step:
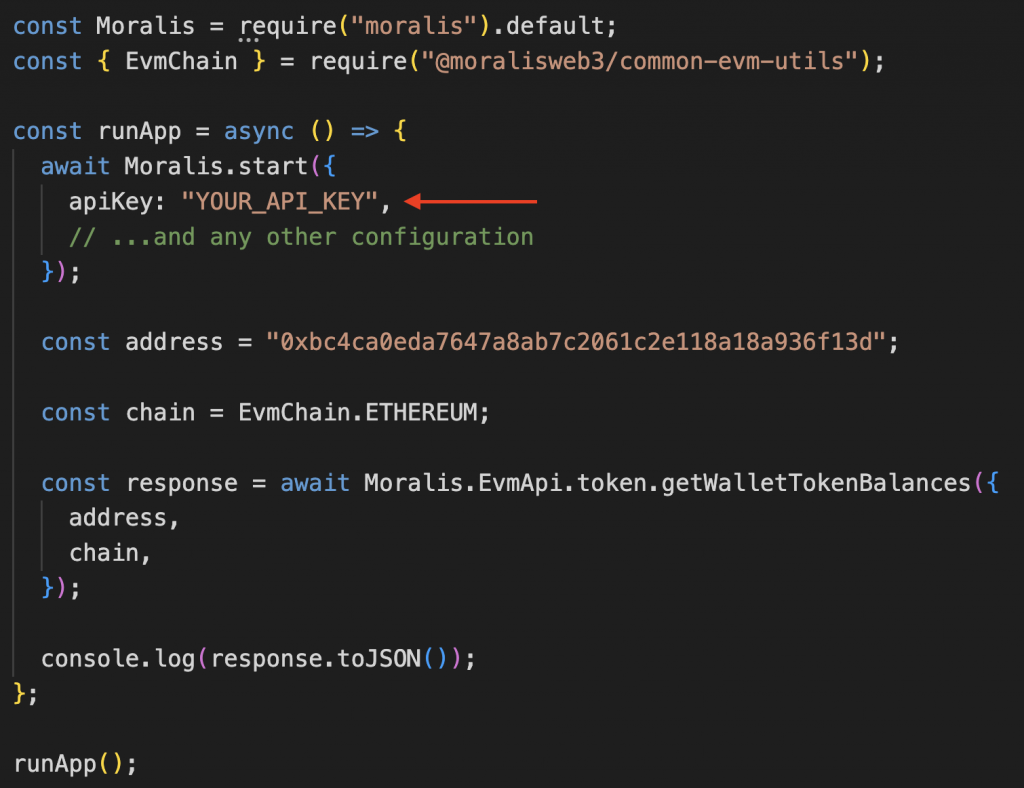
Next, you need to configure the address
and chain
parameters to fit your request. For the address
const, add the wallet address from which you want to get the ERC20 token balance. And for chain
, you can switch the network if you wish to query a blockchain other than Ethereum:
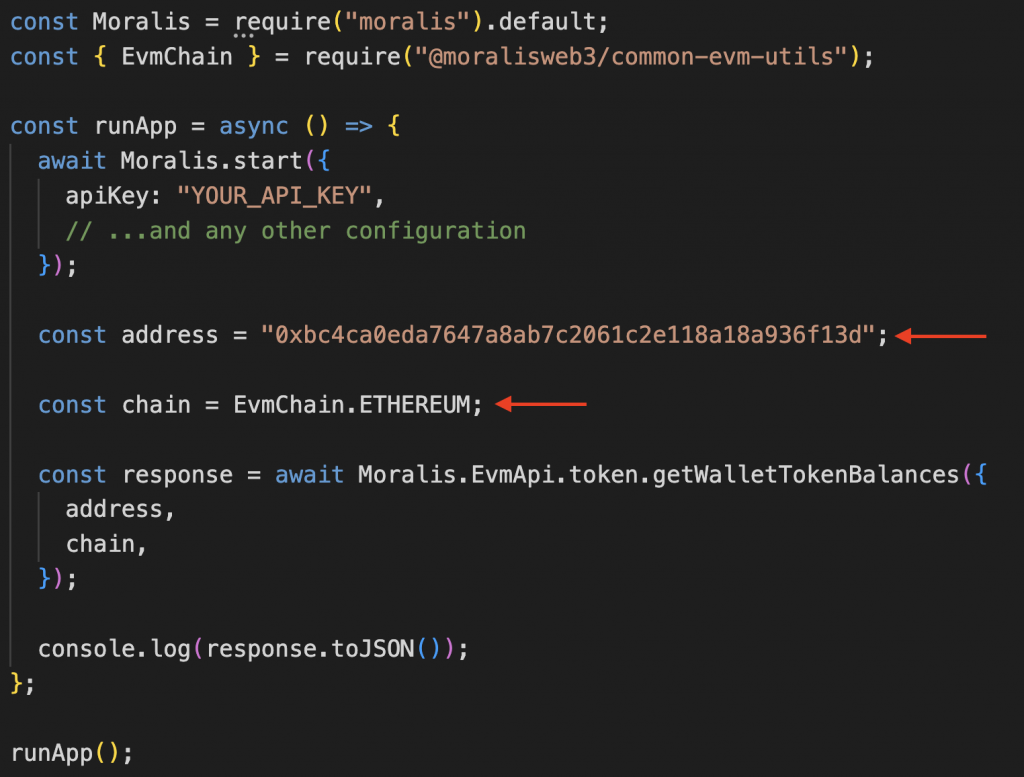
From there, we then pass the parameters when calling the getWalletTokenBalances()
endpoint:

This is it; that’s all the code you need to get the ERC20 token balance of a user. All that remains from here is running the script!
Step 3: Run the Code
To run the script, simply open a terminal, cd
into the project’s root folder, and execute the following command:
node index.js
In return, you’ll get a response containing an array of ERC20 tokens along with the balance for each cryptocurrency. Here’s an example of what it might look like:
[ { "token_address": "0x3c978fc9a42c80a127863d786d8883614b01b3cd", "symbol": "USDT", "name": "USDTOKEN", "logo": null, "thumbnail": null, "decimals": 18, "balance": "10000000000000000000000", "possible_spam": true }, { "token_address": "0xdac17f958d2ee523a2206206994597c13d831ec7", "symbol": "USDT", "name": "Tether USD", "logo": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7.png", "thumbnail": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7_thumb.png", "decimals": 6, "balance": "102847", "possible_spam": false }, //... ]
From here, you can now effortlessly integrate the ERC20 token balance data into your projects!
How to Get Spender Allowance of an ERC20 Token
In addition to getting the ERC20 token balance of a user, the Moralis Token API also allows you to query the spender allowance of an ERC20 token. But what exactly is the spender allowance?
ERC20 token allowance refers to the amount that a spender is allowed to withdraw on behalf of the owner. This is common when interacting with dapps like Uniswap, giving the platform the right to transfer the tokens you hold in your wallet.
So, how can you get the spender allowance of an ERC20 token?
Well, thanks to the accessibility of the Moralis Token API, you can follow the same steps from the ”Tutorial: How to Get the ERC20 Token Balance…” section. The only difference is that you need to call the getTokenAllowance()
endpoint instead of getWalletTokenBalances()
.
As such, if you want to get the spender allowance of an ERC20 token, simply replace the contents in your ”index.js” file with the following code:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.start({ apiKey: "YOUR_API_KEY", // ...and any other configuration }); const chain = EvmChain.ETHEREUM; const address = "0x514910771AF9Ca656af840dff83E8264EcF986CA"; const ownerAddress = "0x7c470D1633711E4b77c8397EBd1dF4095A9e9E02"; const spenderAddress = "0xed33259a056f4fb449ffb7b7e2ecb43a9b5685bf"; const response = await Moralis.EvmApi.token.getTokenAllowance({ address, chain, ownerAddress, spenderAddress, }); console.log(response.toJSON()); }; runApp();
From here, you’ll then need to add your API key by replacing YOUR_API_KEY
:
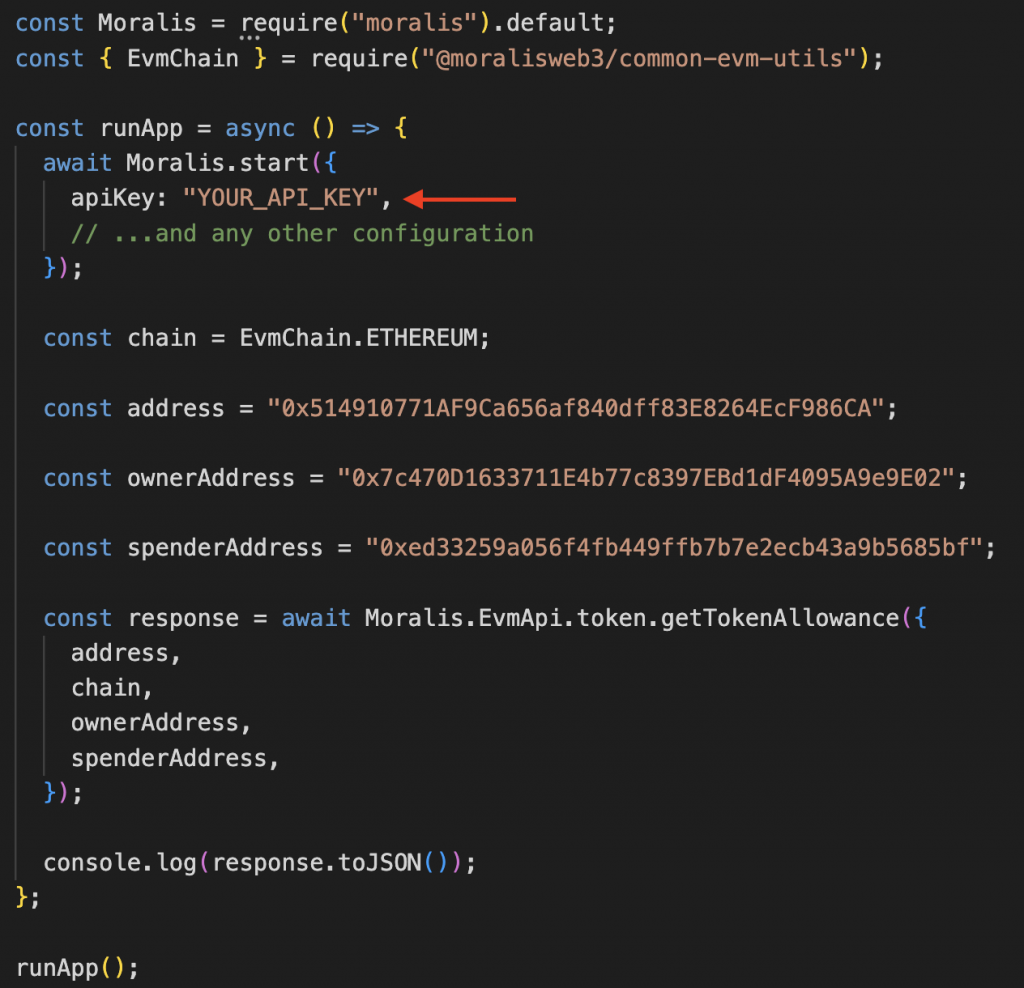
Next, you must configure the parameters, and as you’ll quickly notice, there are a few more this time. For the address
parameter, you need to add the token address. For ownerAddress
, add the address of the token owner. And for spenderAddress
, add the address of the token spender:
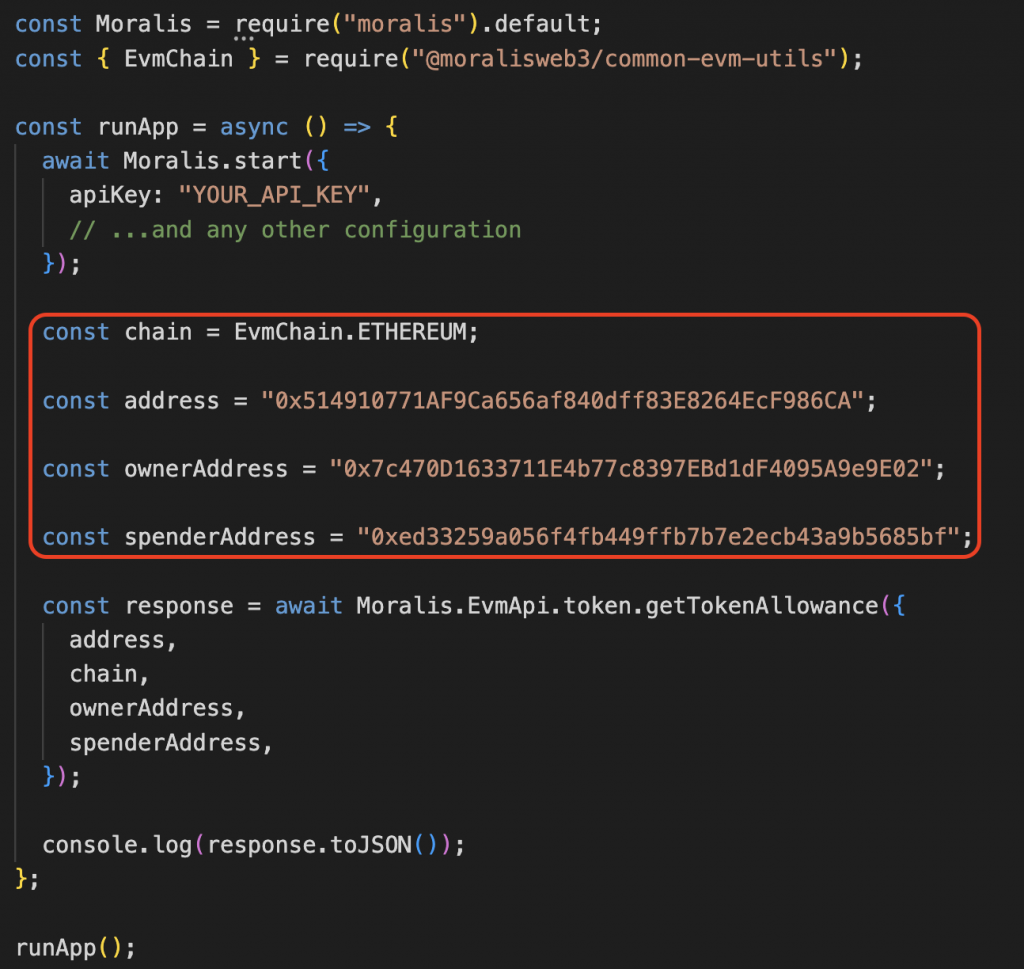
We then pass these parameters when calling the getTokenAllowance()
endpoint:
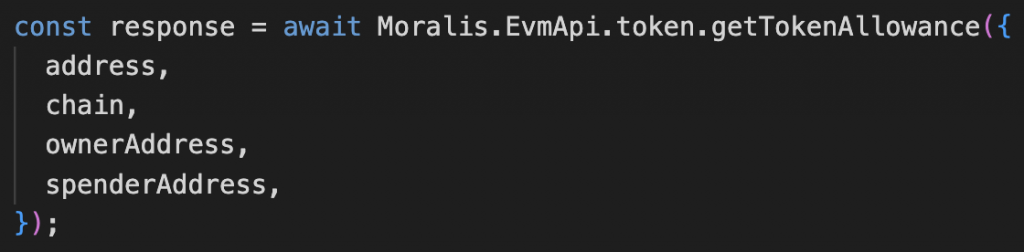
Once you run the code, you’ll get a response looking something like this:
{ "allowance": "0" }
And that’s it! This is how easy it is to get the spender allowance of an ERC20 token when working with Moralis!
For a more detailed breakdown of how this works, check out the get spender allowance of an ERC20 token documentation!
Summary: How to Get the Balance of ERC20 Tokens
In today’s article, we introduced you to ERC20 token balances. In doing so, we explained what they are and how you can get the ERC20 token balance of a user with the Moralis Price API in three steps:
- Get an API Key
- Write a Script
- Run the Code
If you have followed along this far, you now know how to get the ERC20 token balance of any address. You can now use this newly acquired skill to start building your first Web3 project!
If this is your ambition, then you should know that the Token API works like a dream together with our suite of additional crypto APIs. You can, for instance, combine the Token API with the Moralis NFT API to integrate NFT data into your projects. If you’d like to learn more about this, check out our articles on how to get NFT ERC721 on-chain metadata or how to get all NFT tokens owned by a user address.

Also, if you liked this tutorial, consider checking out additional content here on the Web3 blog. For example, dive into account abstraction, explore the industry’s leading NFT image API, or learn how to write a smart contract in Solidity!
Lastly, don’t forget to sign up with Moralis if you want to access the industry’s leading Web3 APIs. Creating an account is free, and as a user of Moralis, you’ll be able to build projects faster and smarter!