In this article, we’ll conduct an in-depth comparison of the ERC721 and ERC1155 standards. While both standards mainly serve NFTs, there are some distinct differences between the two. One of these is that ERC1155 is not just an NFT standard. However, as our ERC721 vs ERC1155 comparison will reveal, ERC721 is, in many cases, a more suitable choice for single NFT projects.
To give you a taste of the ERC721 vs ERC1155 comparison, feel free to explore the following smart contract examples:
- ERC721 smart contract example:
// contracts/GameItem.sol // SPDX-License-Identifier: MIT pragma solidity ^0.6.0; import "@openzeppelin/contracts/token/ERC721/ERC721.sol"; import "@openzeppelin/contracts/utils/Counters.sol"; contract GameItem is ERC721 { using Counters for Counters.Counter; Counters.Counter private _tokenIds; constructor() public ERC721("GameItem", "ITM") {} function awardItem(address player, string memory tokenURI) public returns (uint256) { _tokenIds.increment(); uint256 newItemId = _tokenIds.current(); _mint(player, newItemId); _setTokenURI(newItemId, tokenURI); return newItemId; } }
- ERC1155 smart contract example:
// contracts/GameItems.sol // SPDX-License-Identifier: MIT pragma solidity ^0.6.0; import "@openzeppelin/contracts/token/ERC1155/ERC1155.sol"; contract GameItems is ERC1155 { uint256 public constant COPPER = 0; uint256 public constant CRYSTAL = 1; uint256 public constant ELDER_SWORD = 2; uint256 public constant KNIFE = 3; uint256 public constant WAND = 4; constructor() public ERC1155("https://game.example/api/item/{id}.json") { _mint(msg.sender, COPPER, 10**18, ""); _mint(msg.sender, CRYSTAL, 10**27, ""); _mint(msg.sender, ELDER_SWORD, 1, ""); _mint(msg.sender, KNIFE, 10**9, ""); _mint(msg.sender, WAND, 10**9, ""); } }
Do you feel like you already know who the winner is in the ERC721 vs ERC1155 battle? If so, you are probably eager to start building dapps incorporating tokens following these two standards. In that case, make sure to create your free Moralis account and start BUIDLing!
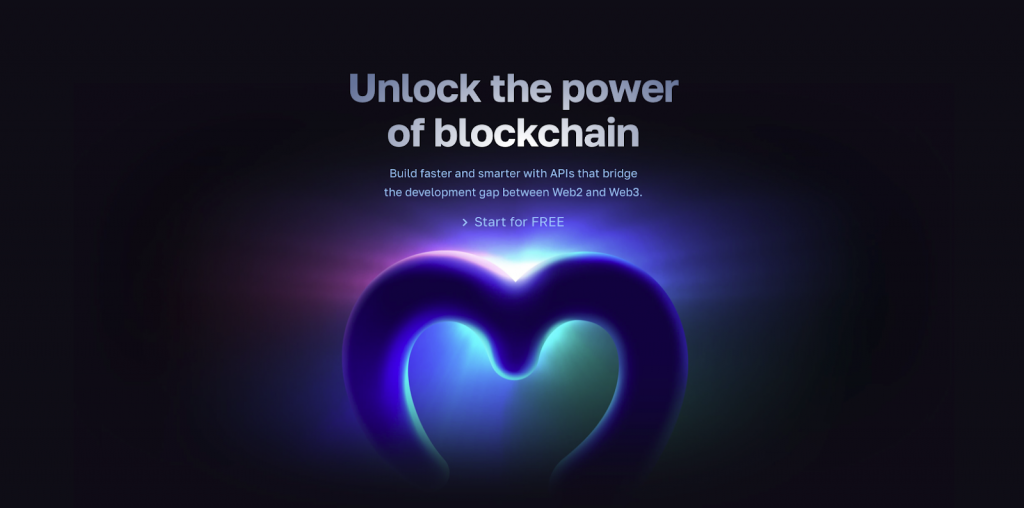
Overview
Our ERC721 vs ERC1155 comparison will be the heart of today’s article. However, before we can place the two standards side by side and point out their major difference, we need to cover the basics. We need to make sure that you have a proper understanding of each standard individually. Therefore, we will answer the “what is ERC721?” and “what is ERC1155?” questions moving forward. Before that, we’ll also ensure that you know what ERC standards are in the first place.
But even before we start exploring ERC standards, we want to examine the possibilities of working with them. After all, unless you are interested in creating your tokens, you do not need to know all the details of today’s comparison. You can simply start building dapps that target existing tokens of these standards and learn more about them on the go.
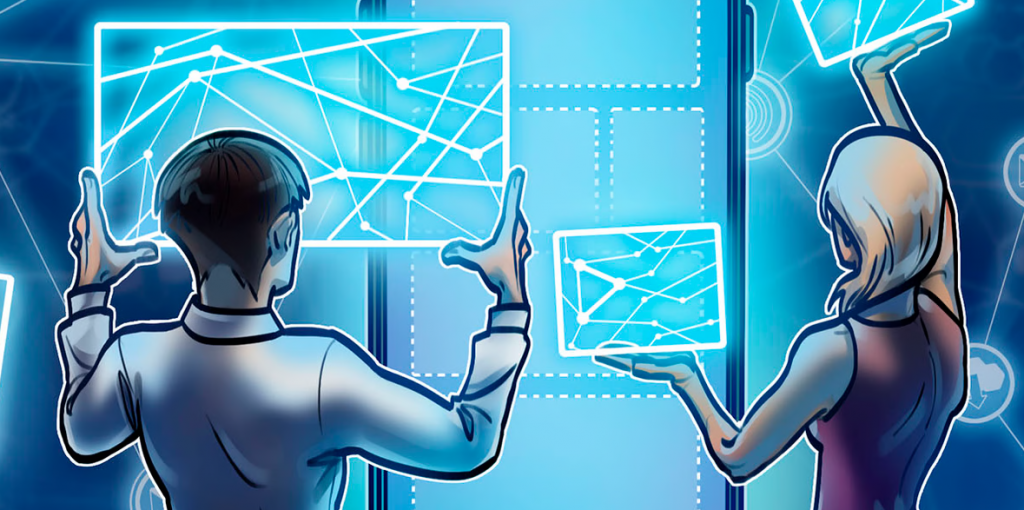
Working with the ERC721 and ERC1155 Token Standards
There are two main ways to work with the ERC721 and ERC1155 standards. On the one hand, you can focus on creating tokens. On the other hand, you can focus on creating user-friendly, engaging dapps around existing tokens that follow the two standards. Of course, you will eventually create your own tokens and build dapps that focus on them. However, the exact path you need to take will most likely reflect your existing skills and interests. So, let’s look more closely at each of the two paths.
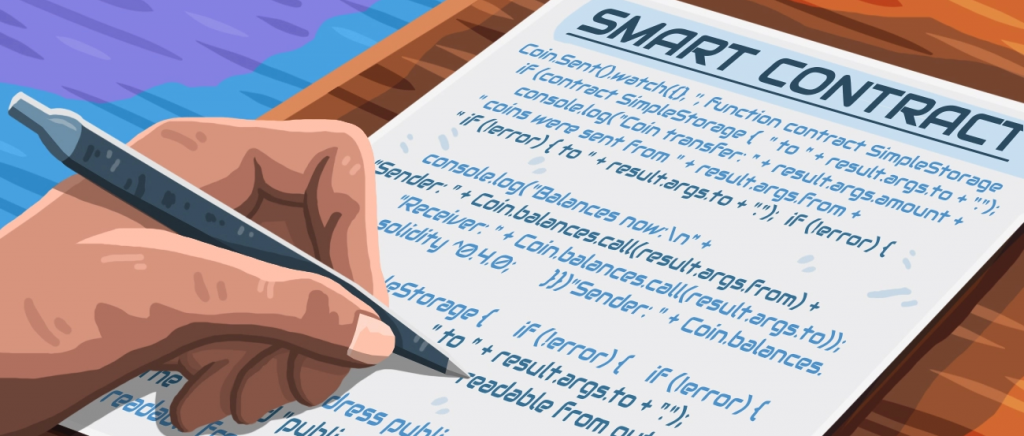
Creating Tokens with ERC721 and ERC1155 Contracts
When you create ERC721 or ERC1155 tokens, you are most likely interested in NFTs. However, with ERC1155, you can create all types of tokens, not just NFTs. That said, remember that when NFTs are in question, you must create or outsource your NFT-representing files and upload them to a proper Web3 storage solution. Then, you must create/generate your NFTs’ metadata files and store them in a decentralized manner. Finally, you can write your smart contract following the ERC721 or ERC1155 standard. In the latter case, you include the URI link to the metadata.
To write smart contracts, you will need to know the basics of Solidity, which is an Ethereum programming language. While mastering Solidity is relatively tricky, getting started with smart contract programming is not that complicated. A good smart contract programming tutorial or a proper course, such as the “Ethereum Smart Contract Programming 101” course from Moralis Academy, can get you on the right track. Plus, open-source resources, such as OpenZepplin, can provide you with verified smart contract templates. As a result, you don’t need to start from scratch.
Building Dapps Around ERC721 and ERC1155 Tokens
Whether you decide to create your tokens or focus on utilizing the existing ones, you will want to reach users by building engaging dapps. They come in all sorts of forms, from simple portfolio trackers to NFT marketplaces, Web3 games, and much more. However, you don’t want to waste time and energy building infrastructure from scratch and reinventing the wheel. Fortunately, you can rely on reputable node and API providers to cover a great portion of your backend needs, and the best Web3 provider for this is Moralis.
Thanks to its cross-platform interoperability, Moralis enables you to join the Web3 revolution with your legacy programming skills and tools. For instance, you can use your JavaScript proficiency to start building killer dapps. Or, you can use Moralis to bring the power of blockchain to platforms such as PlayFab, Firebase, Supabase, AWS Lambda, etc. Moralis’ Web3 APIs bridges the development gap between Web2 and Web3, enabling developers build faster and smarter!
Web3 Data, Authentication, and Streams
Moralis’ Web3 Data API lets you fetch any on-chain data. With the Auth API, you can easily implement Web3 sign-ins. Finally, with the Streams API, you can listen to on-chain events in real-time. So, with this powerhouse, you can build any dapp you can think of.
Aside from targeting ERC721 and ER1155 tokens, you can also use the ultimate ERC20 token API to incorporate ERC20 tokens. For instance, with the best ERC20 token balance API, you can fetch the balance of ERC20 tokens. You can also get contract logs for Ethereum and so much more. Moreover, if you want a tutorial on how to get wallets native crypto balance, then read our “Get Wallet Balance” article. In that case, you’ll be doing most of the heavy lifting with the following snippet of code:
const response = await Moralis.EvmApi.balance.getNativeBalance({ address, chain, });
When working with Moralis’ APIs, you’ll cover most of the blockchain-related backend with short code snippets similar to the one above. As the above “chain” parameter indicates, you can focus on all leading blockchains. After all, Moralis is all about cross-chain interoperability. What’s more, you can access this powerful provider with a free Moralis account!
Exploring ERC Standards
ERC (Ethereum Request for Comment) is a set of technical documents. The latter contains guidelines for developing various types of smart contracts. ERC standards define functions for each token type and facilitate the interaction between smart contracts and applications.
Anyone can create an ERC since Ethereum is an open-source, decentralized network. To do this, one must undergo the process of an “Ethereum improvement proposal” (EIP). Via this document, a developer needs to express proposed features and processes for the Ethereum blockchain network. Then, Ethereum’s core developers assess and examine the proposal. If the community finds the proposal valuable, it will be accepted, finalized, and eventually implemented. The implementation of an EIP turns the initial document into an ERC standard. The latter then serves as a guideline for other devs creating their own tokens. Furthermore, they create a foundation for tokens by providing minimum standard interface requirements.
Moving forward, we will explore ERC721 and ERC1155 further to get you ready for the ERC721 vs ERC1155 comparison. However, it’s worth pointing out that there are several other ERC standards. Here’s a list of the most popular ERC standards:
- ERC20
- ERC165
- ERC721
- ERC223
- ERC621
- ERC777
- ERC827
- ERC884
- ERC865
- ERC1155
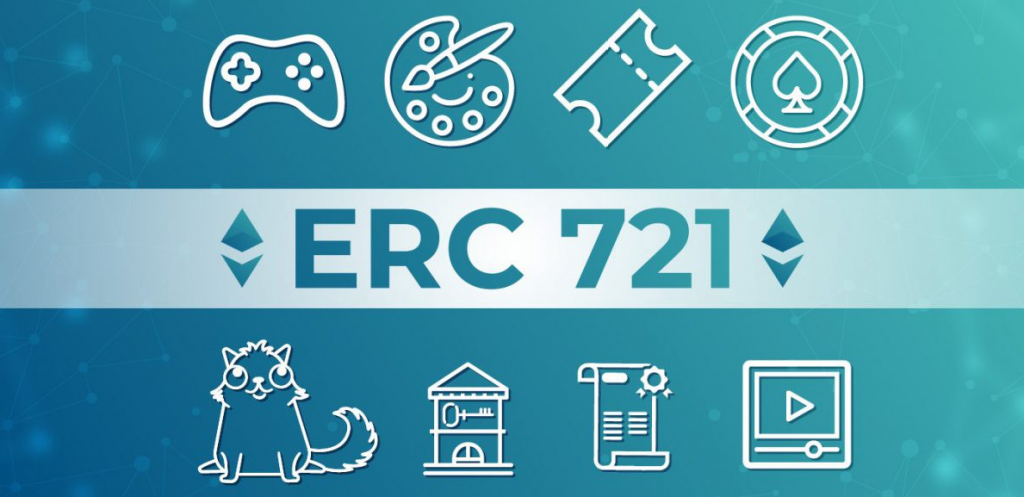
What is ERC721?
ERC721 is a standard focusing on non-fungible tokens (NFTs) in the Ethereum ecosystem. “ERC721” also refers to smart contracts as well as tokens that follow this standard. Ethereum Request for Comments 721 ensures that tokens originating from an ERC721 contract implement a minimum interface. It achieves this by ensuring the following:
- All ERC721 tokens are transferable between accounts
- It’s possible to fetch token balances
- Anyone can check the total supply of a token
Plus, a contract must implement specific events and methods to qualify as an ERC721 contract. You can find these events and methods listed in the “ERC721 Contract” article.
The ERC721 standard originates from Ethereum, but it isn’t universally limited to this blockchain. All blockchains utilizing EVM (Ethereum Virtual Machine) typically support this and other ERC contracts.
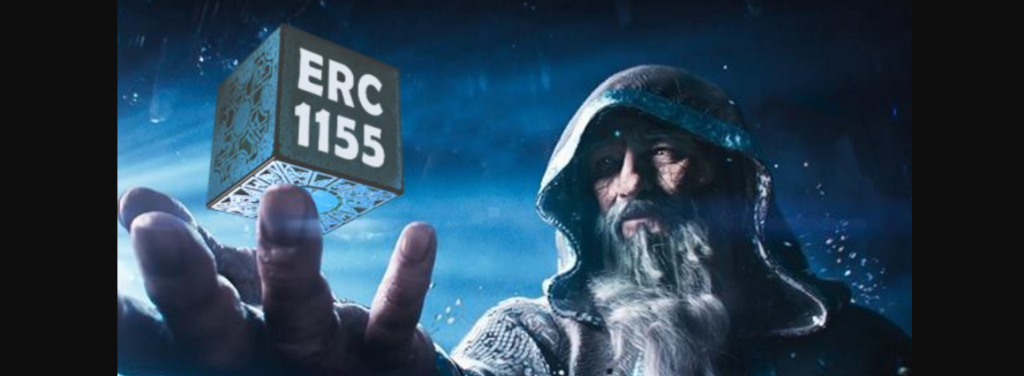
What is ERC1155?
ERC1155 is another standard that focuses on NFTs. However, this standard also targets fungible tokens (FTs), and semi-fungible tokens (SFTs). This makes it the first standard for multi-token minting and transaction management. In addition, ERC1155 is a universal token standard that supports transfers of different types of tokens in a single instance. Plus, just like with “ERC721”, “ERC1155” also refers to smart contracts and tokens marching to the beat of this standard.
The Enjin team submitted the EIP proposal that became ERC1155. Their proposal drew concepts and ideas from several existing token standards, especially ERC20 and ERC721. So, it’s not surprising that ERC1155 contracts merge NFTs and FTs under the same roof. This means that a single contract can manage different types of tokens, reducing the number of smart contracts deployed and minimizing transaction volume. This is the key to expanding NFT utility.
The above-explained universal nature and increased on-chain efficiency made ERC1155 the gold standard for NFTs. Also, with ERC1155, users can create new tokenized items without deploying new contracts. This is how NFT marketplaces can offer “lazy” minting. All in all, ERC1155 takes blockchain development to the next level. Essentially, every new token could easily use this standard, and projects focusing on production should do so. But since ERC20 (for FTs) and ERC721 (for NFTs) bring simplicity to the table, it makes sense to cover simple feats using these two standards.
If you want to dive deeper into this universal token standard, make sure to read our “ERC 1155 NFTs – What is the ERC-1155 Standard?” article. This is also where you can find out what semi-fungible tokens are.
Now that you know the definition of both NFT standards, it’s time we do the ERC721 vs ERC1155 comparison.
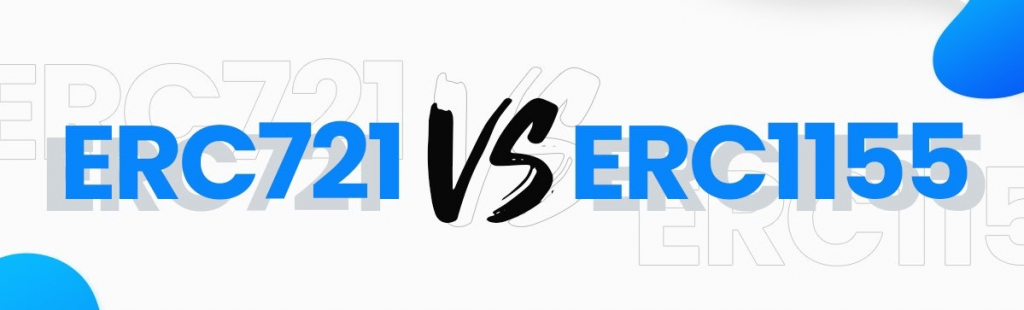
ERC721 vs ERC1155 – Full Comparison
- When?
- ERC721 became an official Ethereum token standard in January 2018.
- ERC1155 became an official Ethereum token standard in June 2019.
- Who?
- William Entriken, Jacob Evans, Nastassia Sachs, and Dieter Shirley (CTO of Dapper Labs) are the co-authors of the ERC721 standard.
- The Enjin team is behind the ERC1155 standard.
- What?
- ERC721 manages NFTs – it allows Web3 wallets to own, trade, and manage these types of tokens.
- ERC1155 does the same (in a more advanced manner) for NFTs, FTs, and SFTs.
Technicalities
- Metadata:
- ERC721 doesn’t require a standard for metadata connected to tokens and only supports static metadata. The latter is stored directly on-chain (in the smart contract) for each token ID. This increases deployment costs and limits flexibility.
- ERC1155 requires standardized metadata for its tokens. Plus, multiple URIs can be linked to smart contracts – smart contracts do not store the actual metadata.
- Ease of use:
- ERC721 requires individual smart contracts for each token.
- A single ERC1155 smart contract supports multiple tokens, even different types of tokens, in a single contract.
- Batch transfer:
- ERC721 doesn’t support batch transfer.
- ERC1155 supports batch transfers with a single smart contract. This can reduce transaction costs and time.
- Semi-fungible tokens:
- ERC721 doesn’t support semi-fungible tokens.
- ERC1155 supports the conversion of fungible tokens into NFTs and vice versa.
- Asset security:
- ERC721 makes it impossible to revert transactions after transferring. If assets are transferred to the wrong address, they’re lost.
- ERC1155 introduces the “safe transfer” function, which enables the verification of transaction validity and enables the reversing of wrong transactions.
- Decentralized exchange (DEX) support:
- ERC721 has no DEX support.
- ERC1155 has built-in code that supports DEXs by fulfilling certain smart contract aspects, which makes ERC1155 tokens serviceable for DEXs.
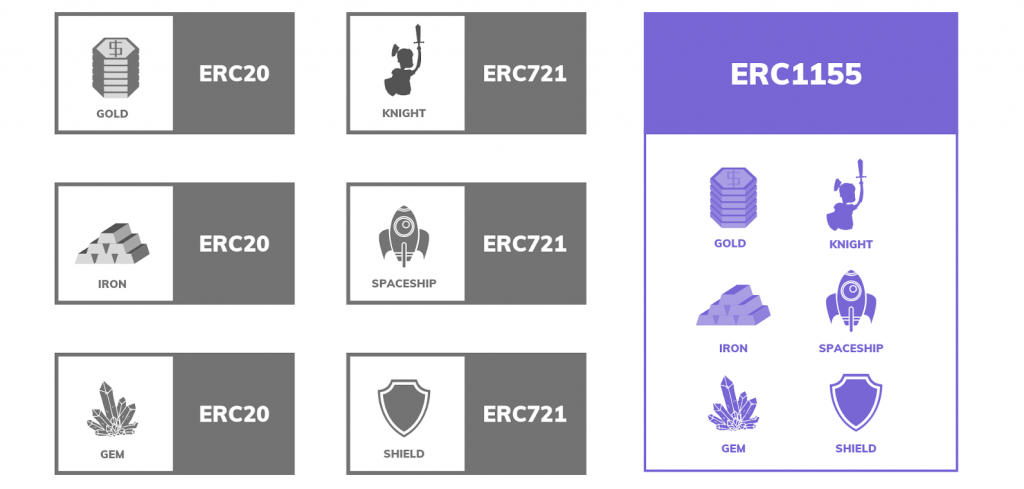
Major Differences
You can see the major differences between the two NFT standards in the above ERC721 vs ERC1155 comparison. Aside from more technical deviations, the core difference is indicated by the above image. While ERC721 only handles NFTs, ERC1155 combines the power of ERC721 and ERC20. Aside from letting you deploy NFTs, FTs, and SFTs in a single smart contract, it also supports a higher level of security, lower gas fees, enhanced support for DEXs, less workload, and dynamic metadata. Consequently, ERC1155 is, in all ways, an improvement of ERC721.
ERC721 was and continues to be a valid NFT standard, but ERC-1155 introduced greater efficiency, flexibility, and security. These improvements allow developers and users of ERC1155 tokens to save time and reduce costs. If existing non-ERC1155 projects want to benefit from these expanded functionalities, they should consider migrating to the new universal standard. After all, when it comes to production, ERC1155 is the gold standard. Still, ERC721 and ERC20 remain simpler and, thus, better options if you want to release individual NFTs and FTs without any advanced utility. So, consider the nature of your project and let it tell you which standard to use.
ERC721 vs ERC1155 – Full-On ERC721 and ERC1155 Comparison – Summary
This article taught you all you need to know to start working with the ERC721 and ERC1155 token standards. You now know that the best way to incorporate these types of tokens is by using Moralis’ powerful Web3 APIs. You also learned that to create your tokens, you need to deploy your smart contracts that comply with these standards. We also listed the most popular ERC standards and looked closely at ERC721 and ERC1155. Finally, we did a detailed ERC721 vs ERC1155 comparison. This is where you learned about the main differences between the two standards and what makes ERC1155 an overall superior option.
If you want to explore these standards further, use the links outlined throughout the article. However, if you are ready to start building cool dapps, let Moralis’ resources help you. The Moralis documentation, our blockchain development videos, and our crypto blog offer everything you need to become a dapp developer for free. These are also the places to access countless tutorials. For example, you can learn what “mint NFT from contract” means. Aside from tutorials, these outlets cover various blockchain development topics. One of our latest articles answers the “what is a Goerli faucet?” question. Another explores how to use a Sepolia testnet faucet, which you will need when you are ready to test your dapps. Of course, you can also take a more professional approach to your Web3 development journey by enrolling in Moralis Academy.