If you’d like to learn how to build a Web3 ChatGPT dapp to mint NFTs, this article is for you! The ChatGPT dapp we’re about to build will enable you to query ChatGPT and convert the provided answer into an NFT. Sounds challenging? Fortunately, it’s pretty straightforward, thanks to OpenAI’s API and the Moralis Web3 API. Now, regarding the implementation of the ChatGPT functionality, the following lines of code do most of the heavy lifting:
app.get("/", async (req, res) => { const { query } = req; try { const response = await openai.createCompletion({ model: "text-davinci-003", prompt: query.message, max_tokens: 30, temperature: 0, }); return res.status(200).json(response.data); } catch (e) { console.log(`Something went wrong ${e}`); return res.status(400).json(); } });
In order to mint chats as NFTs, you must store them in a decentralized manner. This is where you can use IPFS via the Moralis IPFS API. Here’s the snippet of code that covers that part:
app.get("/uploadtoipfs", async (req, res) => { const { query } = req; try { const response = await Moralis.EvmApi.ipfs.uploadFolder({ abi: [ { path: "conversation.json", content: { chat: query.pair }, }, ], }); console.log(response.result); return res.status(200).json(response.result); } catch (e) { console.log(`Something went wrong ${e}`); return res.status(400).json(); } });
Of course, as far as the actual NFT minting goes, you also need a proper smart contract. So, if you are interested in creating your Web3 ChatGPT dapp by implementing the above-presented lines of code and creating a suitable smart contract, follow along in this article or watch the video above. Just create your free Moralis account and follow our lead!
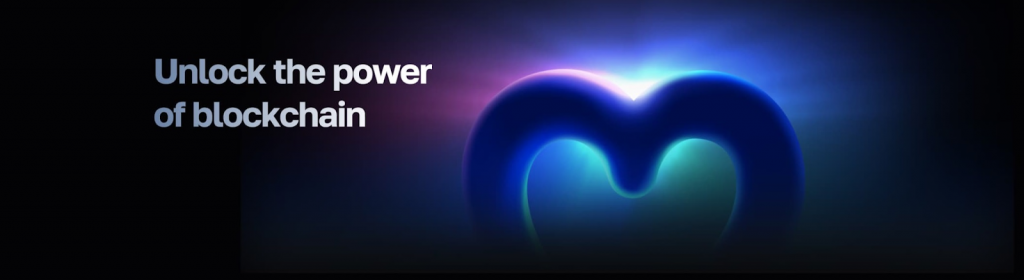
Overview
The majority of today’s article consists of a tutorial demonstrating how to create a Web3 ChatGPT dapp using our code. You will learn how your JavaScript skills can cover both the frontend and backend portions of your ChatGPT dapp. Along the way, you’ll also learn how to use tools such as OpenAI’s API, the Moralis Web3 Data API, and wagmi. You’ll also learn the basics of Solidity. After all, you need to deploy your instance of our example smart contract to create your ChatGPT minter.
For the sake of this tutorial, we’ll be using the Goerli testnet. As such, make sure to connect your MetaMask wallet to this Ethereum testnet and equip your wallet with some Goerli ETH. A reliable Goerli faucet will do the trick.
As a bonus, we’ll also show you how to use the Moralis NFT API to fetch and view the details of NFTs minted with your Web3 ChatGTP dapp. Below the tutorial, you can also find more details about ChatGPT and its use cases.
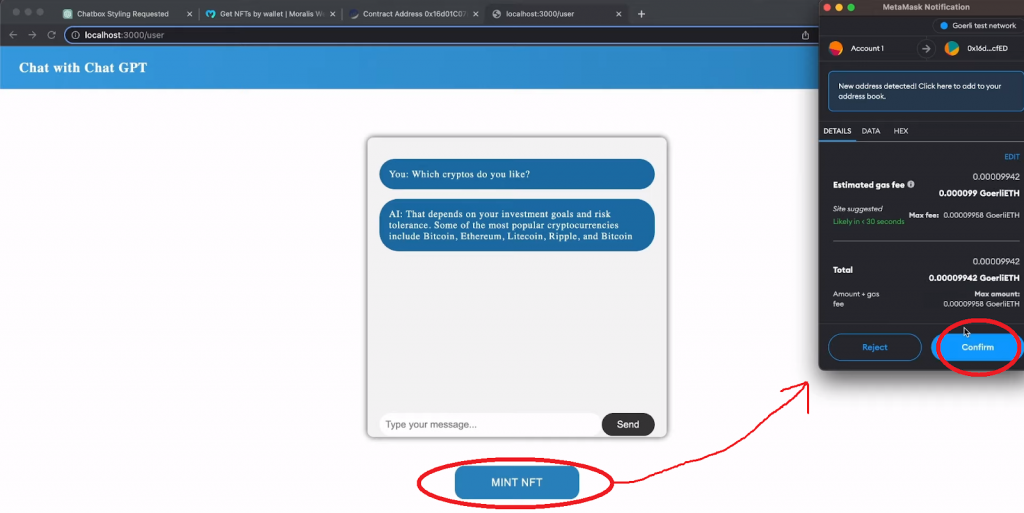
Tutorial: Build Your Web3 ChatGPT NFT Minter Dapp
If you were to build your Web3 ChatGPT minter dapp from scratch, you’d need to complete the following steps:
- Create a NodeJS app and an Express server.
- Install all required dependencies for your backend: CORS, dotenv, Express, Moralis, and OpenAI.
- Create a proper backend “index.js” script covering the backend functionalities of incorporating ChatGPT and the Moralis IPFS API as outlined above.
- Build a frontend NextJS app.
- Install all required frontend dependencies: Moralis, wagmi, NextAuth, etc.
- Code several “.jsx” and “.js” scripts.
- Write and deploy a smart contract that mints a chat once you hit the “Mint NFT” button in the above screenshot.
However, instead of taking on all these steps, you can simply access our “moralis-chatgpt” GitHub repo and clone the entire code. Once you do that and open the project in Visual Studio Code (VSC), you’ll be looking at three folders: “backend”, “hardhat”, and “nextjs_moralis_auth”:

Note: Moving forward, we will proceed as if you’ve cloned our project, and we will carry out a code walkthrough of the most significant scripts.
ChatGPT NFT Minting Smart Contract
If you are familiar with Hardhat, you can take the same path as we did and use Hardhat to create your instance of our smart contract. However, you can also use Remix IDE using your favorite browser to compile and deploy the required smart contract. Either way, use our example smart contract template (“MessageNFT.sol“) located inside the “hardhat/contracts” folder:
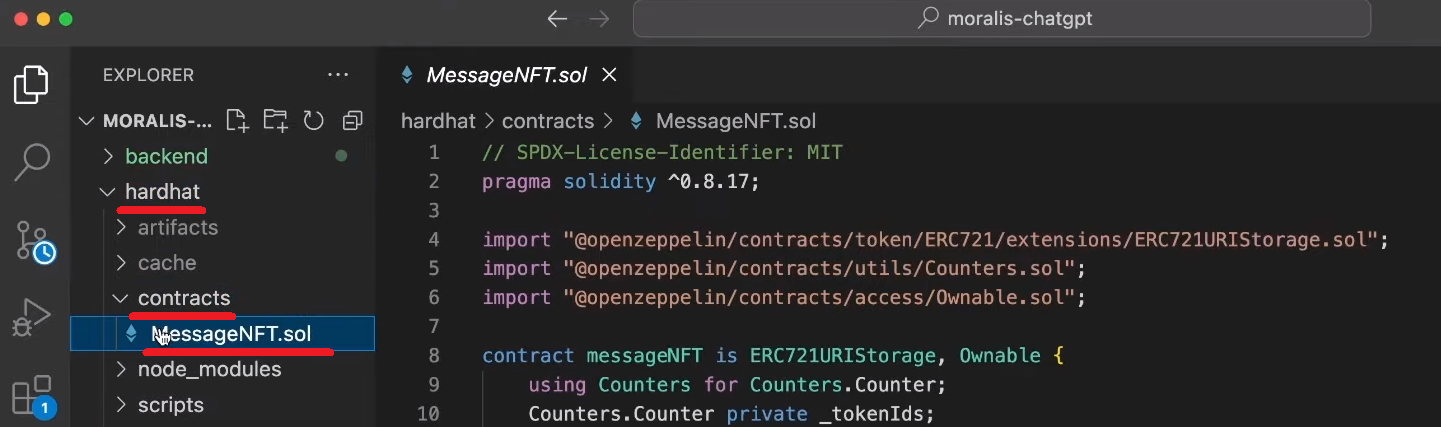
Like all Solidity smart contracts, “MessageNFT.sol” starts with an MIT license and a pragma line:
// SPDX-License-Identifier: MIT pragma solidity ^0.8.17;
Next, it imports three verified smart contracts from OpenZeppelin to inherit the ERC-721 standard, the ability to count NFTs and assign NFT IDs, and functionality that allows only the owner of the smart contract to mint NFTs:
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol"; import "@openzeppelin/contracts/utils/Counters.sol"; import "@openzeppelin/contracts/access/Ownable.sol";
Following the imports, the script defines the contract name and contract type (ERC-721). Inside the contract, the “constructor” gets executed when the contract is deployed, and the “mintNFT” function gets executed whenever this contract is called to mint a relevant NFT:
contract messageNFT is ERC721URIStorage, Ownable { using Counters for Counters.Counter; Counters.Counter private _tokenIds; constructor() ERC721("Chapt GPT Conversation", "CGPTC") {} function mintNFT(address recipient, string memory tokenURI) public onlyOwner returns (uint256) { _tokenIds.increment(); uint256 newItemId = _tokenIds.current(); _mint(recipient, newItemId); _setTokenURI(newItemId, tokenURI); return newItemId; } }
Looking at the “mint” function parameters, you can see that it takes in the wallet address of the recipient and token URI. The former will grab the connected wallet’s address, and the latter will fetch the URI that the IPFS API returns after uploading a chat in question.
Note: When deploying the above smart contract, make sure to focus on the Goerli testnet.
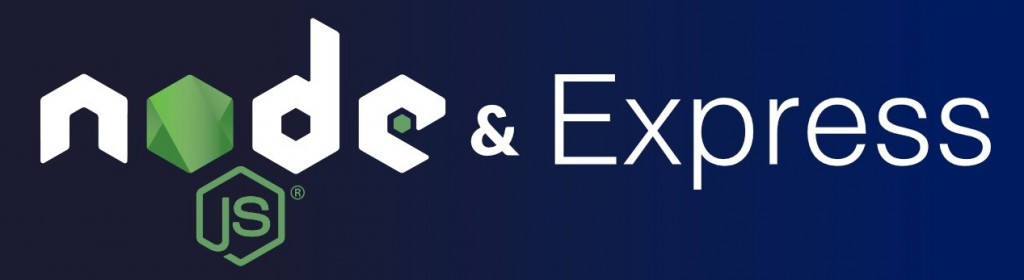
Backend of the Web3 ChatGPT Minter Dapp
Inside the “backend” folder, you can find the “index.js”, “package-lock.json”, and “package.json” files. You can open the latter to see which dependencies are required by this project. Aside from installing the required dependencies, you also need to create a “.env” file. Inside that file, you want to store your Moralis Web3 API key under the “MORALIS_API_KEY” variable.
To obtain your API key, you’ll need a Moralis account. So, in case you haven’t done so yet, create your free Moralis account now. Then, log in and copy your API key from your admin area:

With your Web3 API key in place, you’ll be able to utilize the backend “index.js” script. After all, this is the script that includes the snippets of code outlined in the introduction. With that said, let’s take a closer look at “index.js”.
At the top, the script imports all relevant dependencies using CORS and Express and imports your API key:
const express = require("express"); const app = express(); const port = 5001; const Moralis = require("moralis").default; const cors = require("cors"); require("dotenv").config(); app.use(cors()); app.use(express.json()); const MORALIS_API_KEY = process.env.MORALIS_API_KEY;
Looking at the lines of code above, you can see that your backend will run on localhost 5001. After importing Moralis and your API key, the following snippet (which you can find at the bottom of “index.js”) initializes Moralis:
Moralis.start({ apiKey: MORALIS_API_KEY, }).then(() => { app.listen(port, () => { console.log(`Listening for API Calls`); }); });
The script also imports the OpenAI API configuration lines provided by the OpenAI documentation:
const { Configuration, OpenAIApi } = require("openai"); const configuration = new Configuration({ apiKey: process.env.OPENAI_API_KEY, }); const openai = new OpenAIApi(configuration);
This is also all the surrounding code that will make the snippets of code from the intro work properly. So, let’s take a closer look at those two Express server endpoints.
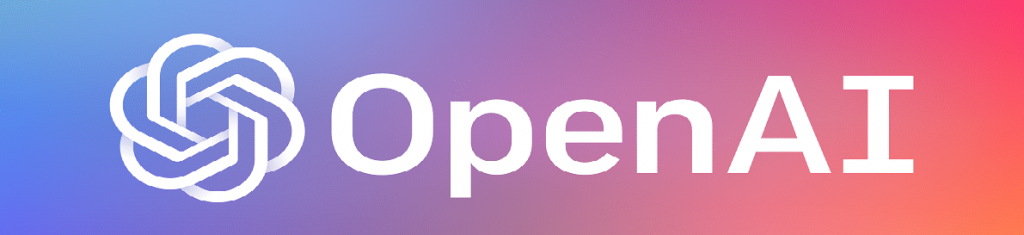
Root Express Server Endpoint
When building a Web3 ChatGPT dapp that mints your chats into NFTs, your backend needs to cover ChatGPT functionality. This is where the OpenAI API enters the scene:
app.get("/", async (req, res) => { const { query } = req; try { const response = await openai.createCompletion({ model: "text-davinci-003", prompt: query.message, max_tokens: 30, temperature: 0, }); return res.status(200).json(response.data); } catch (e) { console.log(`Something went wrong ${e}`); return res.status(400).json(); } });
The above lines of code fetch the message from your entry field on the frontend, pass it to ChatGPT, and return ChatGPT’s reply.
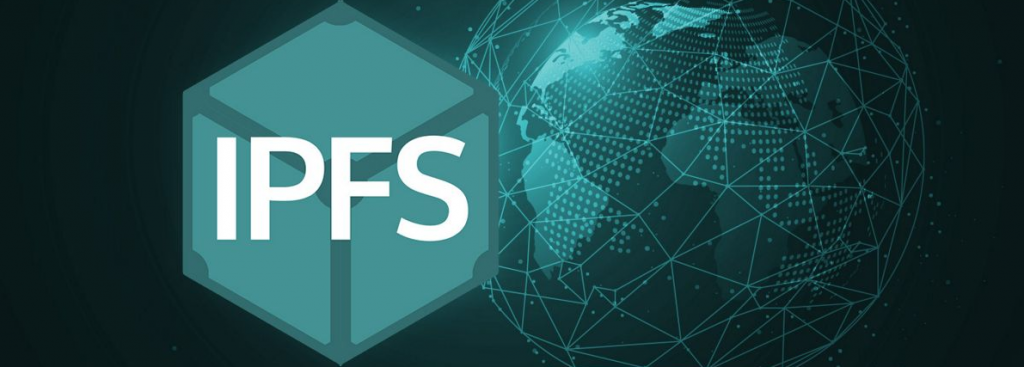
The “/uploadtoipfs” Express Server Endpoint
When creating NFTs, you don’t store the NFT metadata and the NFT-representing files on the blockchain. Instead, you use cloud storage solutions providing you with URIs, which are stored on the blockchain. Of course, you could use any centralized storage solution. However, if you want to take a preferable decentralized approach, you should use one of the leading Web3 storage solutions. IPFS is arguably the best choice when you aim to make files public. Because of this, our backend “index.js” script utilizes IPFS via the IPFS API from Moralis. Here are the lines of code covering the “uploadtoipfs” endpoint:
app.get("/uploadtoipfs", async (req, res) => { const { query } = req; try { const response = await Moralis.EvmApi.ipfs.uploadFolder({ abi: [ { path: "conversation.json", content: { chat: query.pair }, }, ], }); console.log(response.result); return res.status(200).json(response.result); } catch (e) { console.log(`Something went wrong ${e}`); return res.status(400).json(); } });
Above, you can see the “Moralis.EvmApi.ipfs.uploadFolder“ method that uses “conversation.json” as an IPFS path and the currency ChatGPT conversation as the corresponding content.
At this point, you should’ve already deployed your instance of the “MessageNFT.sol” smart contract and have the above-presented backend up and running. Thus, it’s time to tackle the final piece of the “Web3 ChatGPT dapp” puzzle: the frontend.
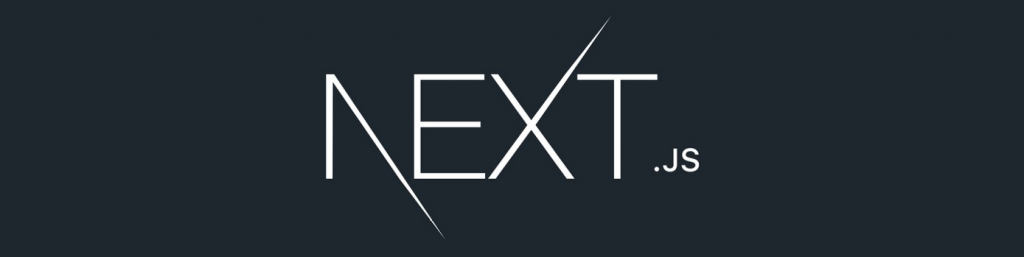
Frontend of the Web3 ChatGPT Minter Dapp
Inside the “_app.jsx” script, located in the “nextjs_moralis_auth/pages” folder, you can see how we wrap “WagmiConfig” and “SessionProvider” around our app. This enables you to use authentication across the entire app:
function MyApp({ Component, pageProps }) { return ( <WagmiConfig client={client}> <SessionProvider session={pageProps.session} refetchInterval={0}> <Component {...pageProps} /> </SessionProvider> </WagmiConfig> ); }
Of course, to make the above work, you need to import the proper providers at the top.
Inside the “signin.jsx” script, our code renders the header and the “Connect” button. Via “handleAuth“, you can connect or disconnect MetaMask. Once connected, the frontend of the Web3 ChatGPT dapp uses the “user.jsx” page, which contains a different header. The “user.js” script also contains the “loggedIn.js” component, which bridges the data between the backend and the frontend. At the bottom of this component, the “loggedIn.js” script renders your frontend:
return ( <section> <section className={styles.chat_box}> <section className={styles.chat_history} id="chatHistory"></section> <section className={styles.message_input}> <input type="text" id="inputField" placeholder="Type your message..." onChange={getMessage} /> <button onClick={sendMessage}>Send</button> </section> </section> {showMintBtn && ( <button className={styles.mint_btn} onClick={mint}> MINT NFT </button> )} </section> ); }
Note: For a more detailed code walkthrough of the “loggedIn.js” script, use the video at the top of this article, starting at 5:53. This is also where you can learn how we used ChatGPT to generate the styling code for our frontend.
Final Build – ChatGPT Minter Demo
Here’s what our ChatGPT NFT minter dapp looks like before connecting MetaMask:

Once we click on the “Authenticate via MetaMask” button, MetaMask pops up and asks us to sign the authentication message:
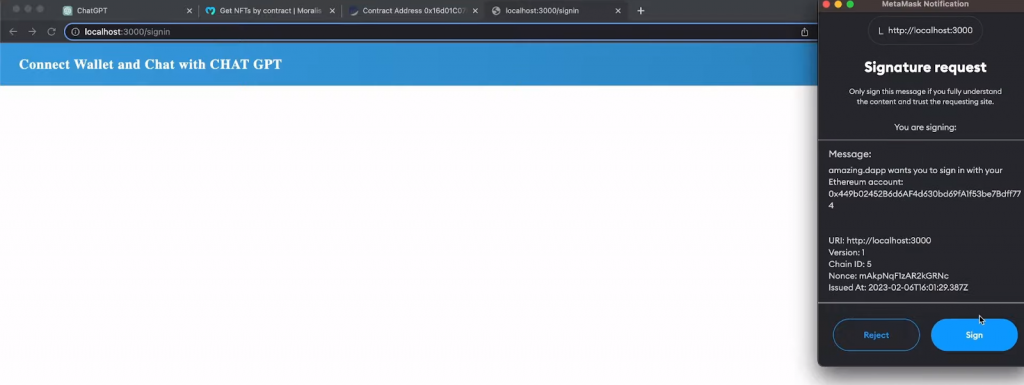
After signing the above signature request, our dapp displays a ChatGPT box and changes the header:
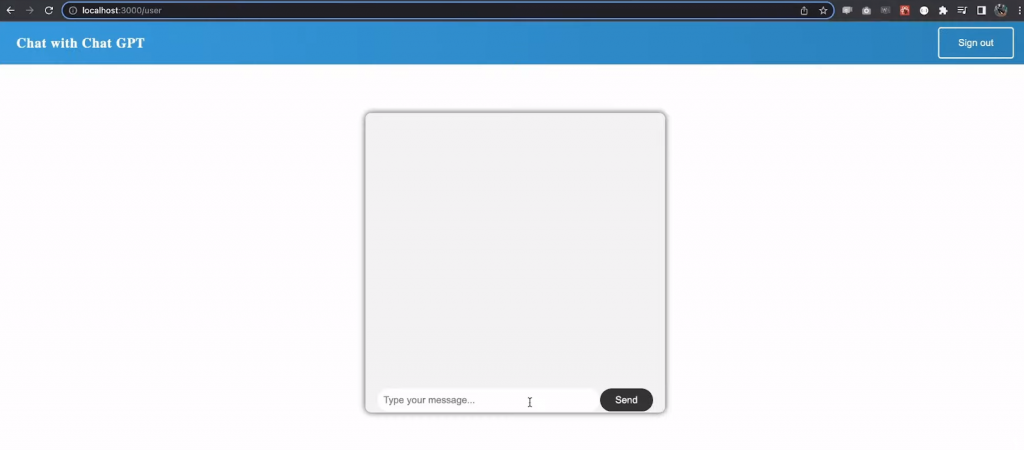
Once we type a message in the entry field and hit “Send”, ChatGPT replies. This also activates the “Mint NFT” button at the bottom:
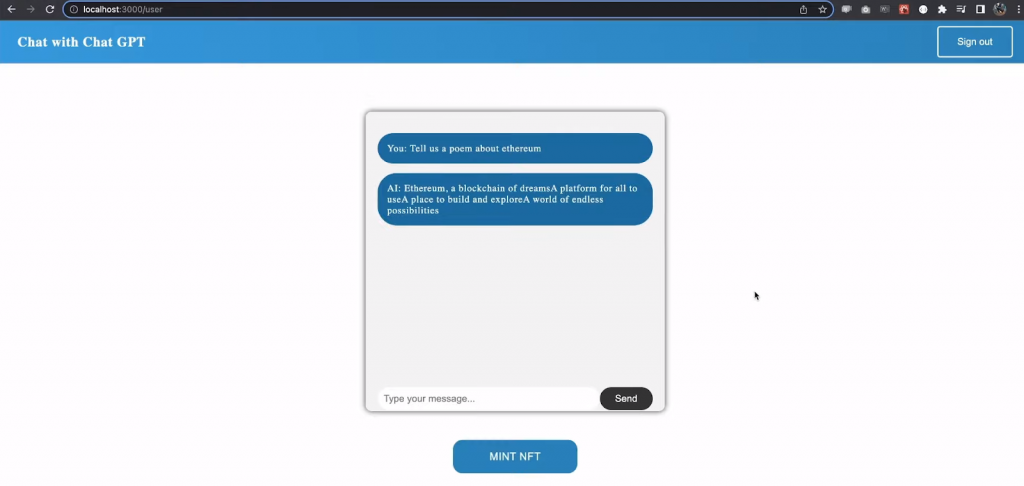
If we decide to convert our chat into an NFT, we need to click on the “Mint NFT” button and then confirm the minting transaction with our MetaMask:
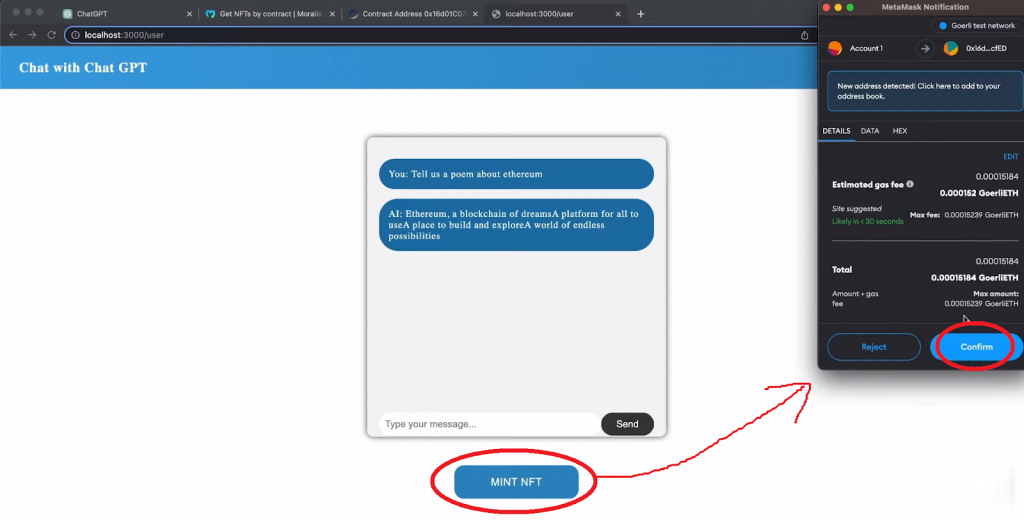
As soon as our transaction goes through, we can view our new ChatGPT NFT on Etherscan or OpenSea. Of course, we can also use the “Get NFTs by contract” API reference page. In that case, we just need to paste in our smart contract’s address and select the Goerli chain:
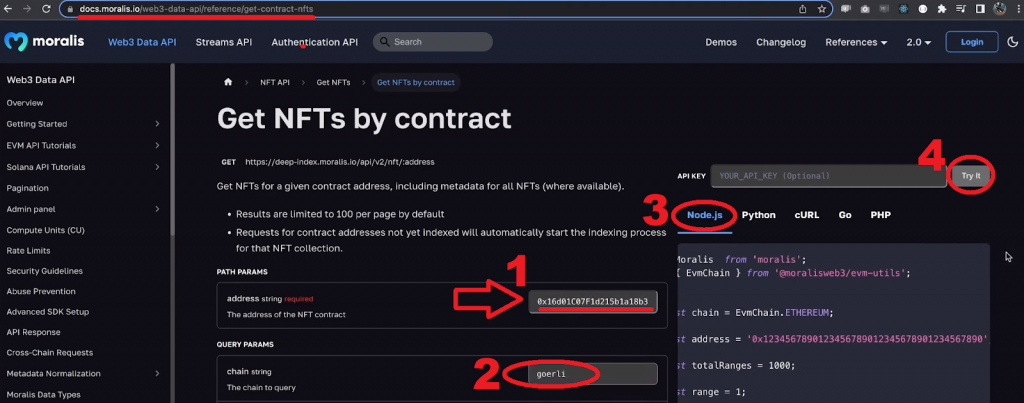
As we scroll down the “Get NFTs by contract” API reference page, we see the response, which contains the details regarding our NFT:

What is ChatGPT?
ChatGPT is an advanced chatbot developed by OpenAI on top of its GPT-3 family. However, who best to explain what ChatGPT is than ChatGPT itself:
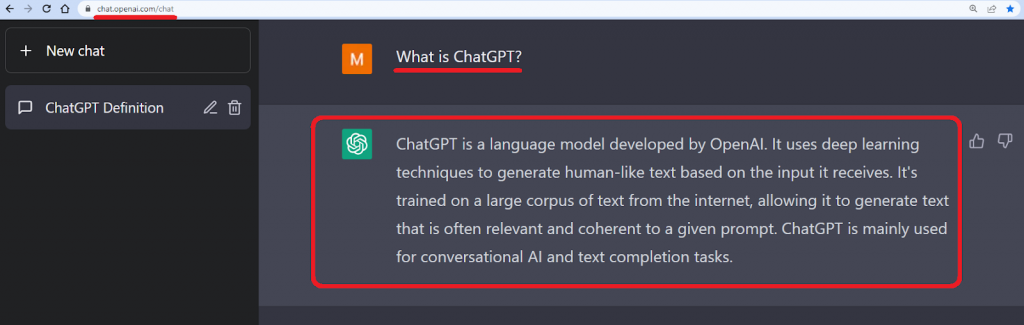
Keep in mind that ChatGPT is in its early stages and contains some limitations. For instance, it occasionally provides incorrect information. For example, it may provide harmful or biased content, and it has limited knowledge of current affairs. With that in mind, ChatGPT must be used cautiously, and its answers must be checked properly.
Still, the power of this tool is quite impressive. After all, it speaks most of the natural languages (though it’s most accurate in English) as well as all the leading computer programming languages. Let’s look at some typical ChatGPT use cases.
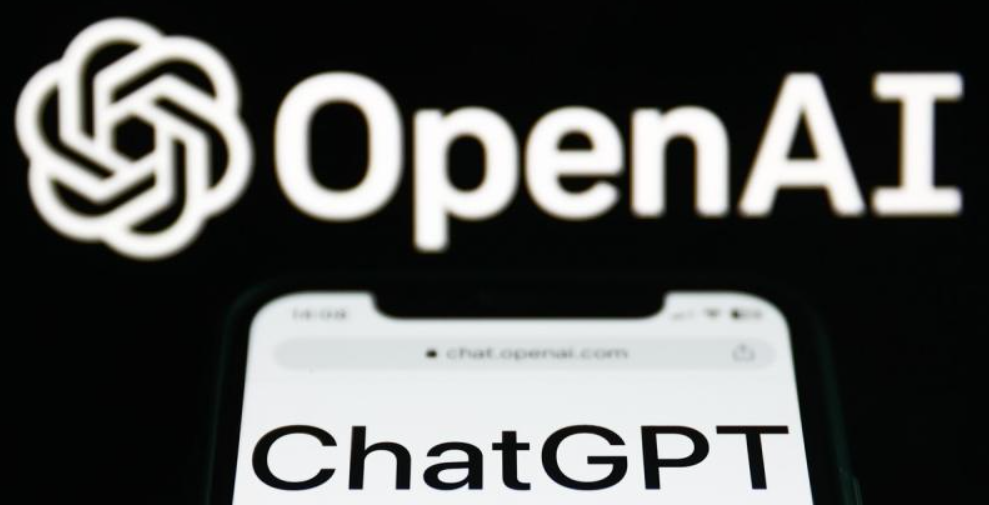
Web3 ChatGPT Use Cases
In many ways, this advanced AI tool is well on its way to becoming for writing what the calculator is for math – a highly powerful tool. Here are some common examples of what users around the globe are using ChatGPT for:
- Development/programming to degenerate Web2 and Web3 code or code templates and find/fix code errors
- Content development
- Marketing and sales pitches
- Accounting and data analytics
The above are just some of the most common ChatGPT use cases. Of course, we can also use OpenAI’s API and implement the power of ChatGPT into all sorts of dapps (like we did in today’s example). Moreover, since ChatGPT has already proven itself as a highly reliable source of information and advanced synthesizing capabilities, experts, such as psychologists and psychiatrists, have reported using it as an assisting tool.

How to Build a Web3 ChatGPT Dapp – Summary
In today’s article, you had a chance to use our example project and guidance to create your instance of our Web3 ChatGPT NFT minting dapp. Using our scripts, you were able to have your smart contract, backend, and frontend ready in minutes. Essentially, you just had to deploy your instance of our smart contract with Hardhat or Remix, install the required frontend and backend dependencies, obtain your Moralis Web3 API key and store it in a “.env” file, and launch your frontend and backend apps. Finally, you also had an opportunity to check out our example dapp demo and see the power of one of the Moralis NFT API endpoints. Nonetheless, we also explained what ChatGPT is and its common use cases.
Moving forward, we encourage you to use this article as an idea generator and as a proof-of-concept example and go out and build unique dapps that incorporate ChatGPT. If you need to expand your Web3 development knowledge and skills first, make sure to use the Moralis docs, our blockchain development videos, and our crypto blog. Some of the latest topics revolve around Ethereum development tools, using IPFS with Ethereum, developing blockchain applications, smart contract security, Solana blockchain app development, Aptos testnet faucet, and much more. In addition, if you are interested in becoming blockchain-certified, Moralis Academy is the place to be. Once enrolled, make sure to first get your blockchain and Bitcoin fundamentals in order.