How do you program smart contracts and implement them? Specifically, how do you accomplish that for the Solana network? Follow along in this article as we demonstrate a three-step process of how to complete the above tasks. The lines of code that will make up your Solana smart contract, or Solana program, will look like this:
use solana_program::{ account_info::AccountInfo, entrypoint, entrypoint::ProgramResult, pubkey::Pubkey, msg, }; entrypoint!(process_instruction); pub fn process_instruction( program_id: &Pubkey, accounts: &[AccountInfo], instruction_data: &[u8] ) -> ProgramResult { msg!("Hello, world!"); Ok(()) }
But the lines of code above are useless unless you have the required prerequisites set in place. Thus, read on as we break down the process step by step on setting up the prerequisites, constructing the contract, and also providing you with a video walkthrough to finalize the process. As a result, you’ll understand how to program smart contracts and implement them on Solana!
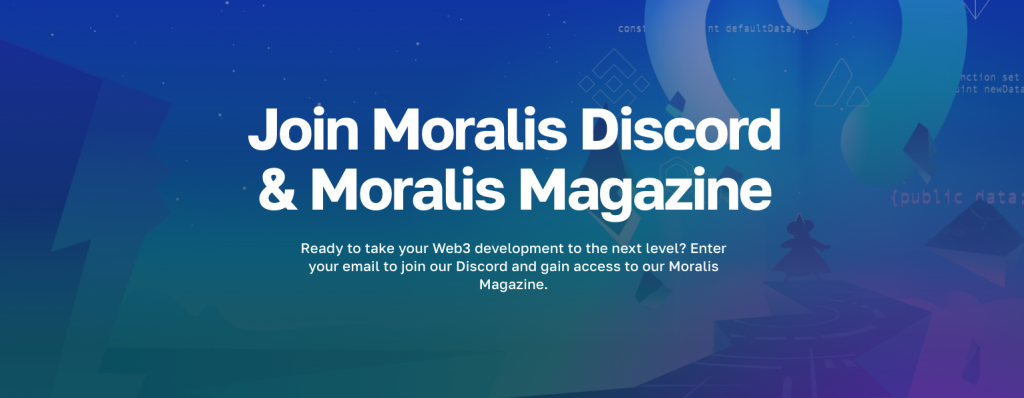
Overview
Moving forward, we’ll first ensure that you understand what smart contracts are. Then, we’ll focus on the programming languages that you will need to use to program smart contracts. This is where we’ll encounter the first major difference between Solana and EVM-compatible chains. After all, programming smart contracts on Solana requires a different approach than Ethereum programming. Moreover, in this article, we will focus on the Solana network. Yet, we’ll look at some smart contract examples for both Ethereum and Solana to ensure you properly understand their key components. Last but not least, we’ll take on a step-by-step tutorial showing how to program smart contracts on Solana. This is also where you’ll use the ultimate Solana API brought to you by Moralis. Hence, make sure to have your free Moralis account ready.

Introduction to Smart Contracts
If you look at the image above, you will see Ethereum’s logo. That’s because Ethereum was the first programmable chain and, thus, the network that came up with the concept of smart contracts. However, these days, we have several reputable programmable blockchains, many of which support smart contracts. Moreover, when it comes to EVM-compatible blockchains, “smart contracts” is the correct term. On the other hand, when it comes to smart contracts on Solana, they are technically called “programs”.
So, whether we talk about “smart contracts” or on-chain “programs”, they are essentially one and the same. Until we focus on how to program smart contracts on Solana or other chains, the differences don’t really matter. After all, they are both pieces of software running on a blockchain designed to trigger specific pre-defined actions when corresponding conditions are met. As such, smart contracts have the power to create trustless contracts and eliminate intermediaries. In turn, there are countless use cases where these on-chain programs are poised to make a huge difference in the future.
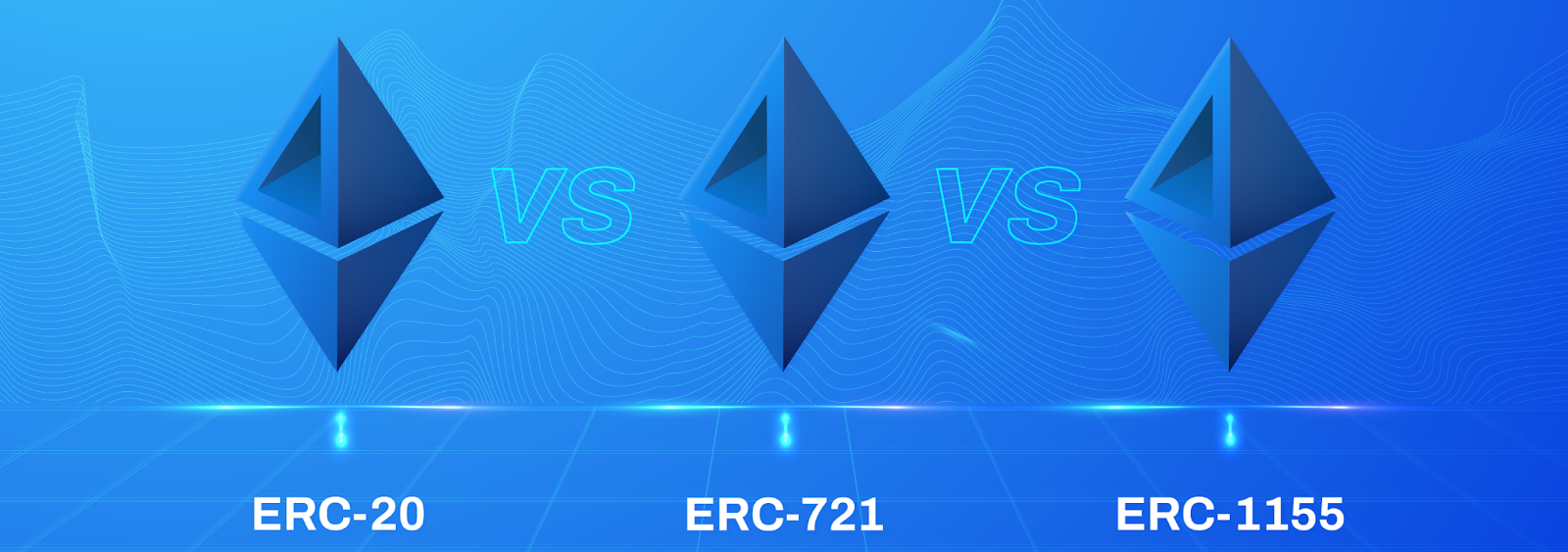
Furthermore, it’s worth pointing out that there’s a smart contract behind every on-chain transaction. Hence, all token (fungible and non-fungible) minting is done by deploying smart contracts. Also, note that there are specific standards that smart contracts must comply with. For instance, on the Ethereum network and EVM-compatible chains, ERC-20, ERC-721, and ERC-1155 smart contracts are used to mint tokens and control their transactions. In addition, these standards also enable devs to use verified smart contract templates.
Now that you know what smart contracts or on-chain programs are, we can focus on the programming languages used to create them. That’s where the smart contract programming task differs between Solana and EVM-compatible chains.
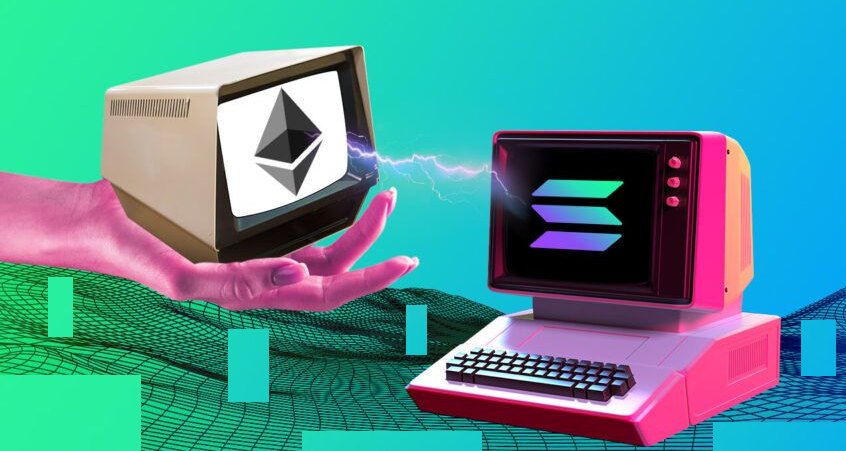
What Programming Language is Used for Smart Contracts?
If you decide to write smart contracts for Ethereum or other EVM-compatible chains, you’ll use the Solidity or Vyper programming languages. Among the two options, Solidity is far more popular. As such, there are more resources, more smart contract templates, etc., for Solidity. Thus, if you decide to develop projects for EVM-compatible chains, we encourage you to start with Solidity.
On the other hand, if you want to learn how to program smart contracts on Solana from scratch, you can’t use Solidity. The programming languages for the Solana chain are Rust, C, and C++, with Rust being the preferred language among developers. Hence, this is the language we will use in the “how to program smart contracts on Solana” tutorial below.

It’s also worth noting that each program on Solana has a unique and identifiable ID to a unique owner that can upgrade the executable code:
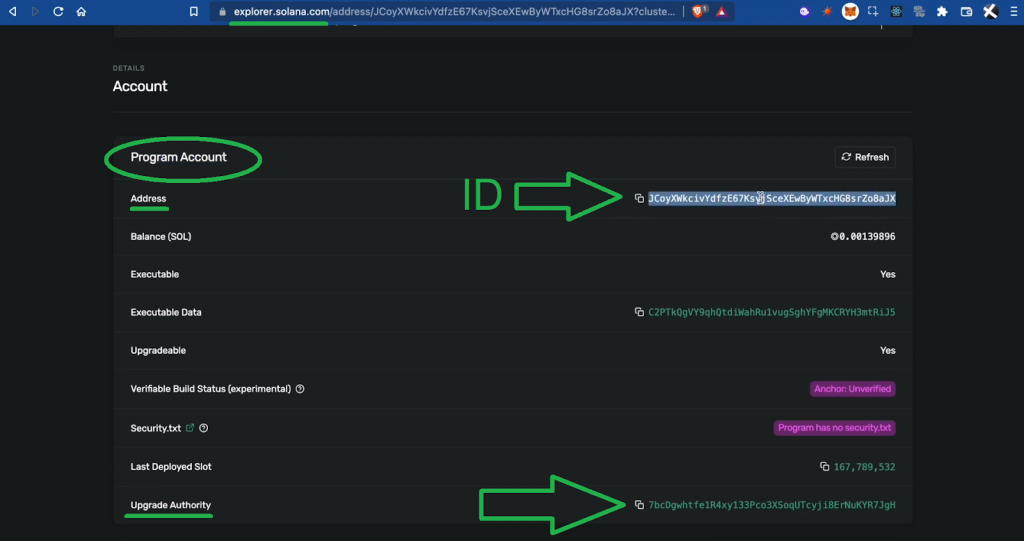
Nonetheless, aside from different programming languages, smart contract development on Solana also requires different tools. For instance, you can’t use the MetaMask Web3 wallet to pay transaction fees on Solana. Also, you can’t use Hardhat or Remix to compile, deploy and verify smart contracts. Instead, you must use tools designed for Solana programming. For one, you need the Solana CLI, and you also need to use a Solana Web3 wallet. You’ll learn more about the tools needed to create Solana programs in the tutorial below.
Note: If you want to learn Rust programming, Moralis Academy has a great course.
Smart Contract Examples
When it comes to finding smart contract examples, Ethereum offers way more templates. After all, the Ethereum blockchain has been around for many years and has a much larger community. However, this also means that Solana offers countless opportunities for new contracts to be developed.

Moreover, smart contracts can be used for various purposes. However, the majority of smart contract examples focus on these use cases:
- Crypto token creation (minting) and transactions
- Document preservation and accessibility
- Administrative payments and billing
- Payrolls
- Taxation
- Pensions
- Insurance
- Bill payments
- Statistics collation
- Health and agricultural supply chains
- Real estate and crowdfunding
- Identity management
That said, we will take a look at the lines of code for a Solana example program in the next section. Below, we focus on two token smart contracts for Ethereum. Also, note that you can find countless verified EVM-compatible smart contracts at OpenZeppelin.
Here is a basic ERC-20 smart contract:
// contracts/GLDToken.sol // SPDX-License-Identifier: MIT pragma solidity ^0.8.0; import "@openzeppelin/contracts/token/ERC20/ERC20.sol"; contract GLDToken is ERC20 { constructor(uint256 initialSupply) ERC20("Gold", "GLD") { _mint(msg.sender, initialSupply); } }
Here’s an example of a basic ERC-721 smart contract:
// contracts/GameItem.sol // SPDX-License-Identifier: MIT pragma solidity ^0.8.0; import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol"; import "@openzeppelin/contracts/utils/Counters.sol"; contract GameItem is ERC721URIStorage { using Counters for Counters.Counter; Counters.Counter private _tokenIds; constructor() ERC721("GameItem", "ITM") {} function awardItem(address player, string memory tokenURI) public returns (uint256) { uint256 newItemId = _tokenIds.current(); _mint(player, newItemId); _setTokenURI(newItemId, tokenURI); _tokenIds.increment(); return newItemId; } }

Tutorial: How to Program Smart Contracts on Solana
Follow the steps covered herein and use the video below for more details on how to complete this tutorial. In short, learning how to program smart contracts on Solana involves the following three steps:
- Install Rust and the Solana CLI
- Create and Deploy an Example Solana Program
- Create a Testing Dapp to Call the Smart Contract
Regarding the first step, you must basically follow the steps outlined in the Solana docs. However, things get a bit more interesting in the second step. Of course, our example Solana program will be pretty straightforward; however, if you have big ideas, this is where you can create all sorts of smart contracts. Though, deploying your smart contract is the same for the simplest and the most complex lines of code.
As far as the third step goes, you’ll have a chance to create an actual dapp. Using our repo, you can do this in minutes. However, you can also use the ultimate Ethereum boilerplate as a starting point and apply the tweaks to create your unique dapp. Moreover, we’ll show you how to obtain your Moralis Web3 API key. After all, the latter is also the gateway to the ultimate Solana API. Finally, you’ll be able to make calls to your example program from your dapp.

Step 1: Install Rust and the Solana CLI
Note: If you prefer to follow along with video instructions, make sure to use the moving picture below, starting at 2:09. Moreover, in case this is not your first rodeo with Solana, and you already have these two tools installed, feel free to skip this step.
Start by opening a new Unix terminal and enter this command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
After running the above command, among several installation types, you’ll need to select one type. For the sake of this tutorial, we encourage you to go with the default option. As such, simply enter “1”:

The process may take some time to finish, but that’s it for the Rust installation. Hence, you can proceed with the Solana CLI installation (3:26). First, enter this command:
sh -c "$(curl -sSfL https://release.solana.com/stable/install)"
With the installation finished, set the “env path“. You can do the latter in your terminal logs:

Moving on, you must create a new local Solana key pair (local wallet). As such, enter these two commands:
mkdir ~/my-solana-wallet
solana-keygen new --outfile ~/my-solana-wallet/my-keypair.json
Then, you’ll be able to acquire your wallet address with the following:
solana address
Next, you also need to set the devnet cluster:
solana config set --url https://api.devnet.solana.com
Last but not least, you must get some test SOL to cover the transactions on Solana devnet. This is the command that will airdrop you one SOL:
solana airdrop 1
The above command line also concludes the initial setup.
Step 2: Create and Deploy an Example Solana Program
Note: Alternatively, use the video below, starting at 5:07.
This step is the gist of the “how to program smart contracts on Solana” quest. We’ll be using the Visual Studio Code (VSC) IDE, and we encourage you to do the same to avoid any unnecessary confusion. Of course, any other IDE will also do the trick.
Inside VSC, create a new folder (our example: “Solana-contract”) and launch a new terminal:

Then, create a new Rust project using the following command:
cargo init hello_world --lib
The above command will add a Cargo library to your project’s folder:

Next, go into the “hello_world” folder. You can do this by entering the following command:
cd hello_world
Once in the above folder, proceed by updating the lines of code in the “Cargo.toml” file. Simply copy-paste the following lines of code:
[lib] name = "hello_world" crate-type = ["cdylib", "lib"]
This is what it looks like in VSC:

Then, open the “lib.rs” file inside the “src” folder and delete its content. Moving forward, add the Solana program package by entering the command below:
cargo add solana_program
Lines of Code for an Example Solana Program
At this point, you have everything ready to add the lines of code that will make up your Solana smart contract (Solana program). As such, paste the following lines of code into “lib.rs:
use solana_program::{ account_info::AccountInfo, entrypoint, entrypoint::ProgramResult, pubkey::Pubkey, msg, }; entrypoint!(process_instruction); pub fn process_instruction( program_id: &Pubkey, accounts: &[AccountInfo], instruction_data: &[u8] ) -> ProgramResult { msg!("Hello, world!"); Ok(()) }
Note: For the code walkthrough, use the video below.
With the above code in place, you can proceed by building the project. As such, enter this command into your terminal:
cargo build-bpf
Once the above command builds the project, you can deploy your Solana program using the “solana program deploy” command. You need to complement this command with a target path. Accordingly, enter this command:
solana program deploy ./target/deploy/hello_world.so
As a result, you should get your program ID (you’ll use that in step three):

With the first two steps under your belt, you already know how to program smart contracts on Solana. Hence, it’s time to also learn how to utilize Solana programs.
Step 3: Create a Testing Dapp to Call the Smart Contract
As promised, this is where we hand you over to the video tutorial below, starting at 9:38. Essentially, you just need to clone the code from GitHub, add your Moralis Web3 API key and your Solana program ID:
Clone our code:
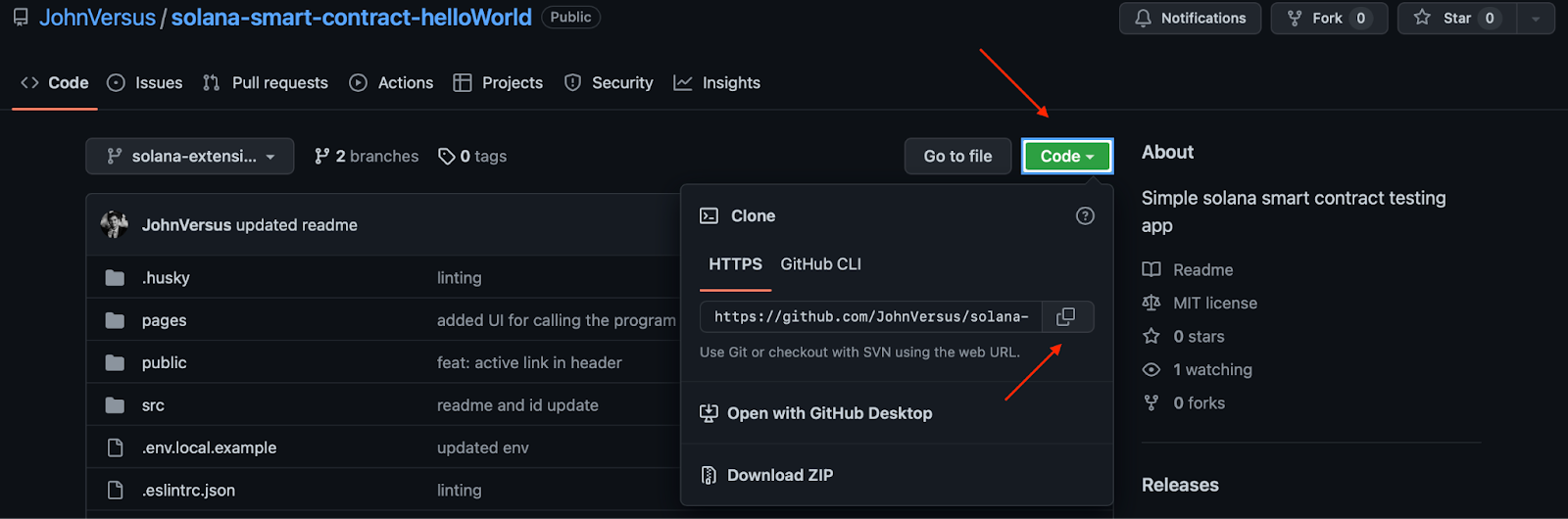
Obtain your Web3 API key (you need a Moralis account for that) and paste it into the “.env.local” file:
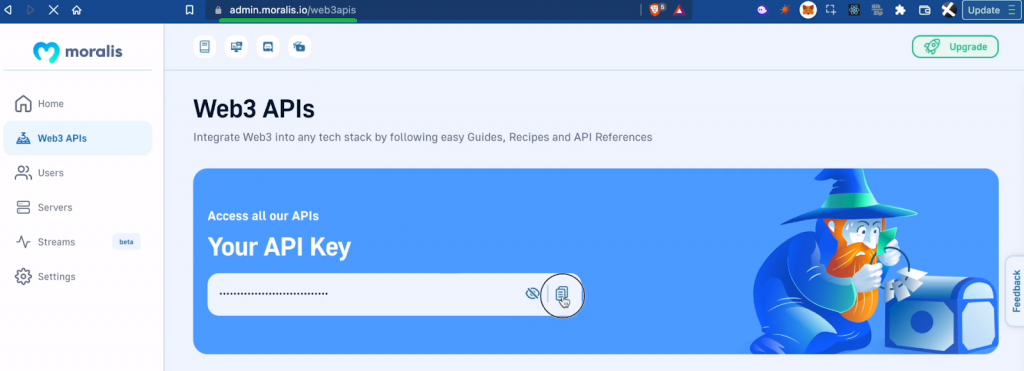
Paste your Solana program ID (end of step two) into the designated line of the “HelloWorld.tsx” file:

Also, make sure to play around with your new Solana dapp to test if it runs your smart contract.
How to Program Smart Contracts and Implement Them on Solana – Summary
In today’s article, you had a chance to learn how to program smart contracts on Solana. However, before we took on the tutorial, we covered the basics. As such, you learned that smart contracts are executable clusters of code stored on a blockchain. These lines of code can read and modify on-chain accounts. You also learned that smart contracts on Solana are referred to as programs. Furthermore, we explained that Solana and EVM-compatible chains use different smart contract programming languages. The latter use Solidity and Vyper, while Rust is the most popular option for Solana. We also looked at some smart contract examples. Last but not least, you had a chance to follow our lead and create and deploy your own Solana program. Moreover, you also had an opportunity to experience the power of Moralis by creating your dapp in minutes.
If you are interested in learning more about Solana or Ethereum development, make sure to visit the Moralis documentation, the Moralis YouTube channel, and the Moralis blog. These resources contain a perfect combination of tutorials and simple explanations that can help you become a Web3 developer for free. Some of our latest articles show you how to add dynamic Web3 authentication to a website, how to use Firebase as a proxy API for Web3, how to integrate blockchain-based authentication, how to create dapps using a NodeJS Web3 example, and much more.
Additionally, you may be interested in a more professional approach to your crypto education. If so, Moralis Academy is what you need. There, you’ll become blockchain certified, which will help you go full-time crypto markedly quicker.