Today’s tutorial will show you how to create a Python backend dapp that utilizes Solana API endpoints from Moralis. You’ll be able to get native, fungible, and NFT balances and portfolios by wallet addresses. You’ll also learn how to get NFT metadata and token prices. Thanks to Moralis’ Solana Python API, you can, for example, fetch wallet balances with the following code snippet:
@app.post("/getWalletbalance") def getWalletbalance(): body = request.json params = { "address": body["address"], "network": body["network"] } result = sol_api.account.balance( api_key= moralis_api_key, params = params ) return result
The lines of code for the other five endpoints are pretty similar. Essentially, they just replace “getWalletbalance” and “sol_api.account.balance“. So, do you want to learn how to work with Moralis’ Solana Python API and implement the above lines of code, including the other five Solana API endpoints? If so, make sure to create your free Moralis account and follow our lead!
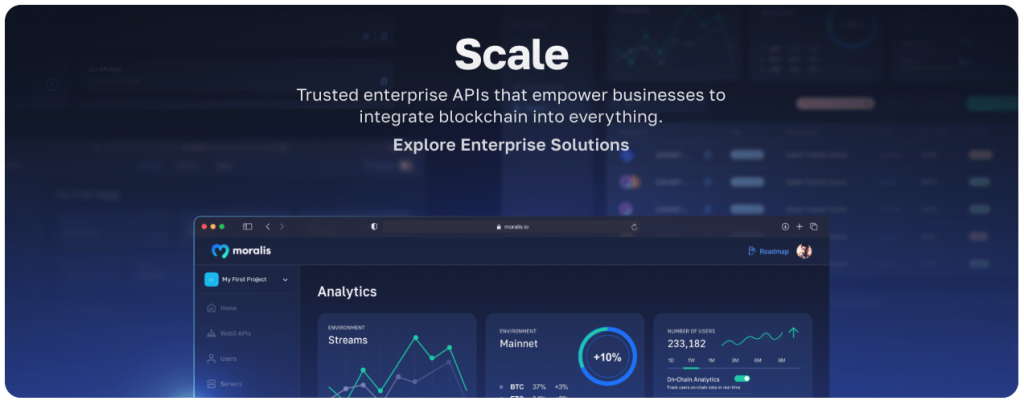
Overview
The core of today’s article will be our Solana Python API tutorial. By following our steps, you’ll learn to complete the initial setup and implement all current Moralis Solana APIs. By cloning our existing frontend dapp, you will also be able to test the backend functionalities. Since we already have a NodeJS backend dapp that covers the same six endpoints, this tutorial also demonstrates how to easily transition from NodeJS to Python. So, if you want to learn how to use the Solana API in Python, roll up your sleeves and follow our steps.
The sections below the tutorial include the theoretical aspects of today’s topic. This is where you can learn what Solana and Python are and discover more details about Moralis’ Solana Python API and how Moralis can further elevate your Web3 development game on Solana and other popular programmable chains. After all, the endpoints you’ll implement by completing today’s tutorial are just a small part of what Moralis offers.

Solana Python API Tutorial
As mentioned above, we will use the frontend we created as part of our Solana JavaScript development. This means that we will simply replace our NodeJS backend with Python without affecting the frontend.

So, here is our simple frontend enabling you to utilize the power of the Solana API endpoints. Of course, the actual functionality depends on the backend that we will focus on herein. Here is a screenshot of our frontend dapp:

Note: If you’re interested in exploring the code behind the frontend dapp, use the video at the top, starting at 1:05. Plus, you can access the complete frontend script – “index.html” – on GitHub. In fact, in order to test the backend that you’re about to build using Python, we encourage you to clone our frontend.
Before we show you how to use the Solana Python API, you must complete the necessary setups. That said, the following section focuses specifically on today’s example project; if you prefer to use more general “Python and Web3” setup instructions, make sure to use our Web3 Python documentation page.
Python Backend Setup
You should already have a “frontend” folder inside your “Solana API demo” project that contains the above-mentioned “index.html” script. If you decided to clone our NodeJS backend, you might have a “backend” folder there as well. This is what we are starting with:

Our first step is to create a “python-backend” folder. We do this by entering the following command into our terminal:
mkdir python-backend
Then, we “cd” into this new folder:
cd python-backend
Next, we create a new virtual environment for installing and using Python modules. We do this with the command below:
Python3 -m venv venv
Before we can use the virtual environment, we also need to activate it:
For that purpose, we run the following command:
source venv/bin/activate
Then, we also need to install Flask, Flask CORS, Moralis, and Python “dotenv” modules:
pip install flask flask_cors moralis python-dotenv
Once the above modules are installed, our virtual environment is ready to be used. As such, we can proceed with setting up the environment variables. This is where we’ll store the Moralis Web3 API key, which is the key to accessing the power of Moralis’ Solana Python API. So, in case you haven’t created your Moralis account yet, make sure to do so now. Then, you’ll be able to copy your Web3 API key from your admin area in two clicks:
If you have your NodeJS backend files in the same project, you can simply copy the “.env” file from there and paste it into your “python-backend” folder. Otherwise, create a new “.env” file. Inside that file, you need to have the “MORALIS_API_KEY” variable that holds the above-obtained API key as a value.
Now that we have our virtual environment ready, we can focus on implementing Solana Python API endpoints.
How to Use the Solana API in Python
To implement the Solana Python API endpoints, we need to create a new “index.py” file inside our “python-backend” folder. At the top of that script, we import the above-installed packages:
from flask import Flask, request from flask_cors import CORS from moralis import sol_api from dotenv import dotenv_values
Next, we need to ensure this script obtains our Web3 API key from the “.env” file:
config = dotenv_values(".env") moralis_api_key = config.get("MORALIS_API_KEY")
We define a variable “app” using Flask in the following line, and we also include “CORS” in that variable:
app = Flask(__name__) CORS(app)
We want our backend dapp to run on port 9000 (the same as our NodeJS backend). That way, we don’t need to modify the URL in our frontend. So, we add the following code snippet at the bottom of our script:
if __name__ == "__main__": app.run(debug=True, host="0.0.0.0", port=9000)
With the above lines of code in place, we can start implementing Moralis’ Solana API endpoints:
- Balance API endpoints:
- Get native balance by wallet
- Get token balance by wallet
- Get portfolio by wallet
- Token API endpoints:
- Get token price
- NFT API endpoints:
- Get NFTs by wallet
- Get NFT metadata
Instead of starting from scratch, you can always copy code snippets for each endpoint from the API reference pages inside the Moralis documentation. Here’s an example:

Implementing Solana Python API Endpoints
In our “index.py” script, just below the “CORS(app)” line, we need to define routes and functions for each endpoint. Starting with the “get native balance by wallet” endpoint, the lines of code in the intro do the trick.
With “@app.post(“/getWalletbalance”)“, we create a new route in Python. Then, we use “def getWalletbalance():” to define the function for this endpoint. Inside the function, we read the JSON data with “body = request.json“. Next, we define the endpoint’s parameter (all Moralis Solana API endpoints only require the “address” and “network” parameters). Then, we use the “sol_api.account.balance” method on our parameters and Web3 API key and store its data under the “result” variable. Finally, we return results by returning the “result” variable. Again, here are the lines of code for the “getWalletbalance” endpoint:
@app.post("/getWalletbalance") def getWalletbalance(): body = request.json params = { "address": body["address"], "network": body["network"] } result = sol_api.account.balance( api_key= moralis_api_key, params = params ) return result
Other endpoints follow the exact same principles; we only need to change the routes, function names, and methods accordingly. Below are the snippets of code for the remaining five Solana Python API endpoints.
- The lines of code for the “getTokenbalance” endpoint:
@app.post("/getTokenbalance") def getTokenbalance(): body = request.json params = { "address": body["address"], "network": body["network"] } result = sol_api.account.get_spl( api_key= moralis_api_key, params = params ) return result
- The lines of code for the “getNfts” endpoint:
@app.post("/getNfts") def getNfts(): body = request.json params = { "address": body["address"], "network": body["network"] } result = sol_api.account.get_nfts( api_key= moralis_api_key, params = params ) return result
- The lines of code for the “getPortfolio” endpoint:
@app.post("/getPortfolio") def getPortfolio(): body = request.json params = { "address": body["address"], "network": body["network"] } result = sol_api.account.get_portfolio( api_key= moralis_api_key, params = params ) return result
- The lines of code for the “getNFTMetadata” endpoint:
@app.post("/getNFTMetadata") def getNFTMetadata(): body = request.json params = { "address": body["address"], "network": body["network"] } result = sol_api.nft.get_nft_metadata( api_key= moralis_api_key, params = params ) return result
- The lines of code for the “getTokenPrice” endpoint:
@app.post("/getTokenPrice") def getTokenPrice(): body = request.json params = { "address": body["address"], "network": body["network"] } result = sol_api.token.get_token_price( api_key= moralis_api_key, params = params ) return result
Note: You can access the complete “index.py” script in our GitHub repo.
Testing Our Python Backend
While inside the “python-backend” folder, we use our terminal to run the “index.py” script with the following command:
Python3 index.py
This starts our backend on port 9000. Next, we need to start our frontend. We do this with the “Live Server” extension in Visual Studio Code (VSC) by right-clicking our “index.html” script in the file tree:
Then, we use our frontend dapp by entering the required values, selecting the network type, and hitting the trigger button. Here are two examples:
- The “Get Native Balance by Wallet” feature:
- The “Get Token Balance by Wallet” feature:
Of course, the other four features work in the same manner.
Exploring Solana, Python, and the Leading Web3 API Provider
The following sections are for those of you who are new to Solana or Python programming. These sections simply explain what Solana and Python are, and once you know the basics, you can further explore how Moralis can aid in your Solana programming endeavors.
What is Solana?
Solana is a public and open-source programmable blockchain that supports smart contracts. The latter are called “programs” on Solana. Via these on-chain programs, Solana also supports the creation of fungible and non-fungible tokens (NFTs) and all sorts of dapps (decentralized applications). If you are familiar with Ethereum or any other programmable chain, you probably know that they usually maintain native coins. Solana is no exception with its native coin, “SOL”. The SOL asset primarily provides network security via Solana’s hybrid DeFi staking consensus. SOL is also the currency used to cover transaction fees on Solana and can serve as a means to transfer value on the Solana chain.
Anatoly Yakovenko and Raj Gokal are the two leading developers who launched Solana back in 2017. Both Yakovenko and Gokal are still significantly involved with Solana Labs – a technology company that builds products, tools, and reference implementations to further expand the Solana ecosystem.
If you are interested in learning more about the Solana network, read one of our past articles that dive deeper into the “what is Solana?” topic.
What is Python?
Python is a popular object-oriented, high-level programming language bellowed by numerous developers. After all, it has a rather long history – it’s been on the screen since 1991. Plus, there are many resemblances between Python and other programming languages, such as Ruby, Scheme, Perl, and Java. Python was designed by the developer Guido van Rossum, who sought to make it as straightforward as possible. Those who know and use Python claim it is rather easy to get started with, easy to learn, and easy to use. Moreover, according to “python.org“, this programming language lets you work quickly and integrate systems more effectively.
Compared to JavaScript, which continues to be the most popular programming language, Python has fewer users. However, it is among the top programming languages and is finding its way into Web3 development as well. Aside from Solana dapps, you can also use Python for Ethereum development and all other EVM-compatible chains.

Solana Python API by Moralis
If you took on today’s tutorial, you had a chance to learn about the current Moralis Solana API endpoints. You even had an opportunity to implement them using the Solana Python API – the tool that enables you to develop dapps on Solana using Python. As such, you now know how to fetch NFT metadata, wallet portfolios, token balances, SPL token prices, and more. As such, you can use this amazing tool to create killer dapps. For instance, you can build NFT marketplaces, token price feeds, portfolio apps, and even Web3 games. Moralis also supports both the Solana mainnet and Solana devnet. The latter enables you to test your dapps before taking them live.
There’s another great tool you can use when creating decentralized applications – Moralis’ Web3 Auth API. The latter enables you to implement Web3 signups and logins on Solana and many other blockchains. So, aside from the Solana Python API, Moralis enables you to use Python to create dapps on all leading blockchains. When you focus on Ethereum and EVM-compatible chains, you can also listen to smart contracts and real-time wallet events with the Moralis Streams API. This further expands the range of functionalities you can cover with your dapps.
If you’d like to explore other development topics and follow along in other step-by-step tutorials, make sure to check out how to “create ERC20 token” and get Goerli ETH. Or, explore how to easily calculate gwei to ether using an already-developed calculator!
Solana Python API – How to Use the Solana API in Python – Summary
Solana is one of the most popular non-EVM-compatible blockchains. At the same time, Python continues to gain popularity among Web3 developers. With that in mind, knowing how to make the most of a reliable Solana Python API can make all the difference when developing dapps. Thanks to today’s tutorial, you know that to start working with this powerful API tool, you only need a free Moralis account.
If you are serious about Solana development, you should also know how to answer the “what is a Solana wallet?” question. In time, you’ll also want to explore Solana smart contract building. However, you can start by looking at some Solana smart contract examples. After all, the right kind of verified contract is one of the essential Solana NFT mint tools and arguably the easiest way to create NFTs.
Whether you want to focus on building dapps on Solana or any other leading blockchain, Moralis has your back. You can use JS, Python, or many other Moralis SDKs to join the Web3 revolution. Plus, Moralis’ YouTube channel and crypto blog can help you become a Web3 developer for free.
However, you may be interested in taking a more professional approach to your blockchain development. In that case, you should consider enrolling in Moralis Academy. There, you’ll get to attend many pro-grade courses, such as the “Solana Programming 101” course!