Are you looking for the easiest way to get all owners of an ERC20 token? If so, then you’re exactly where you need to be! In today’s guide, we’ll show you how to get sorted holders of a token in a heartbeat using Moralis’ Token API. If you immediately want to jump straight into the code, check out our ERC20 Owners endpoint in action down below:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch( "https://deep-index.moralis.io/api/v2.2/erc20/0x7d1afa7b718fb893db30a3abc0cfc608aacfebb0/owners?chain=eth&order=DESC", options ) .then((response) => response.json()) .then((response) => console.log(response)) .catch((err) => console.error(err));
In return for running the code above, you’ll get an array comprising all owners of the token in question in descending order – cryptocurrency whales first! What’s more, the response is enriched with additional data, such as owner percentages, the USD value of individual holdings, etc. Here’s an example of what it might look like:
{ "cursor": "eyJhbGciOiJIUz//...", "page": 1, "page_size": 100, "result": [ { "balance": "3704449652939473320142469218", "balance_formatted": "3704449652.939473320142469218", "is_contract": false, "owner_address": "0x5e3ef299fddf15eaa0432e6e66473ace8c13d908", "owner_address_label": "Polygon (Matic): PoS Staking Contract", "usd_value": "3489442144.205111503601074219", "percentage_relative_to_total_supply": 37.044496529394735 }, //... ] }
Getting sorted holders of a token doesn’t have to be more challenging than that. However, if you want a more detailed breakdown, please check out the documentation page for our ERC20 Owners endpoint or join us in this guide.
Also, if you wish to get all owners of an ERC20 token yourself, don’t forget to sign up with Moralis. You can create an account for free, and you’ll gain instant access to our premier suite of development tools!
Leading Web3 APIs?
Overview
Identifying the owners of an ERC20 token has been quite a bothersome task from a conventional perspective. Typically, this process requires manually monitoring ownership changes and transactions across diverse protocols, creating a workflow that is both time-intensive and complex. Surely, there must be a more efficient method, right?
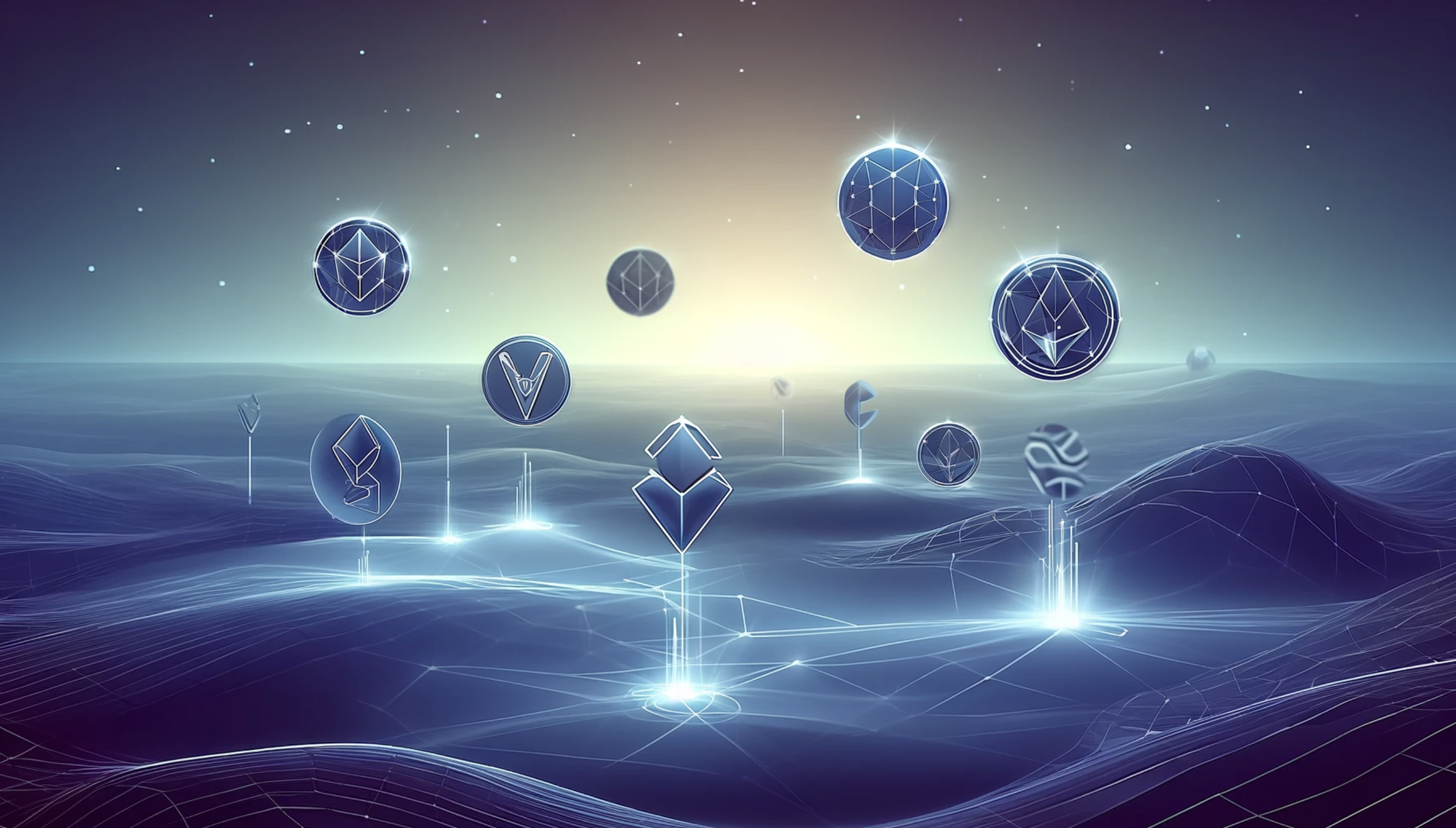
At Moralis, we set out to transform this traditionally difficult process into a simple task you can complete without breaking a sweat. And this is precisely what our ERC20 Owners endpoint allows you to do. So, if you’d like to explore the easiest way to get sorted holders of a token, then this read is for you. Let’s jump straight into it by introducing Moralis!
What is Moralis? – The Easiest Way to Get All Owners of an ERC20 Token
Moralis is the industry’s leading Web3 data provider, supplying the resources you need to build sophisticated dapps. In our suite of development tools, you’ll find everything from top-tier blockchain nodes to ten+ use case-specific APIs. What’s more, Moralis currently powers blockchain and crypto projects for millions of end users all across the globe!
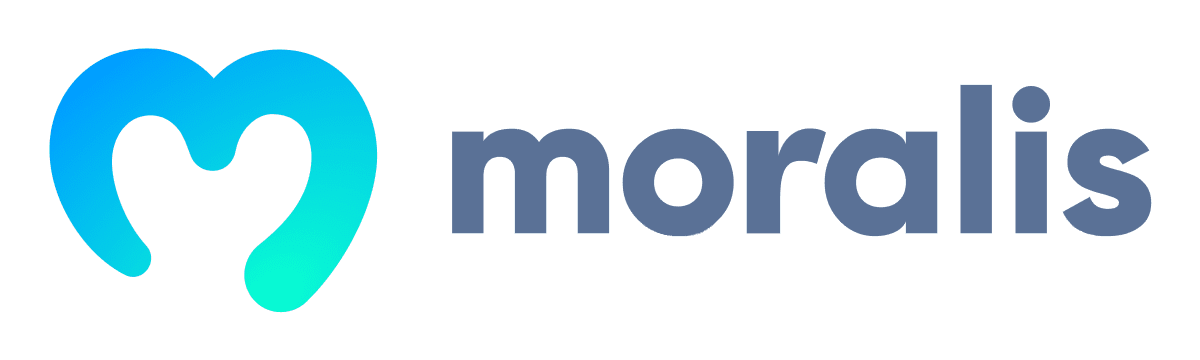
So, why should you use Moralis when building dapps?
- One API – All the Data: All our APIs are designed to minimize the requests you need to build dapps. This means you get more data with fewer API calls when working with Moralis, leading to lower costs and enhanced efficiency.
- Streamline Your Development Experience: Moralis’ APIs are intuitive and fully cross-chain. As such, you don’t have to bother with multiple providers for various chains when using our APIs, giving you a significantly more accessible development experience.
- Trusted By Industry Leaders: Moralis is used by 100,000+ developers and trusted by industry leaders like MetaMask, Blockchain.com, and Opera.

Nevertheless, that gives you an overview of Moralis. Let’s now dive a bit deeper into our Web3 API suite and explore the Token API – the best tool to get all owners of an ERC20 token!
Moralis’ Token API and the ERC20 Owners Endpoint
Moralis’ Token API is the industry’s #1 tool for ERC20 token data, supporting every single token across all major chains. This includes stablecoins, meme coins, LP tokens, and everything in between. So, if you’re looking to integrate ERC20s into your dapps, then the Token API is the tool for you!
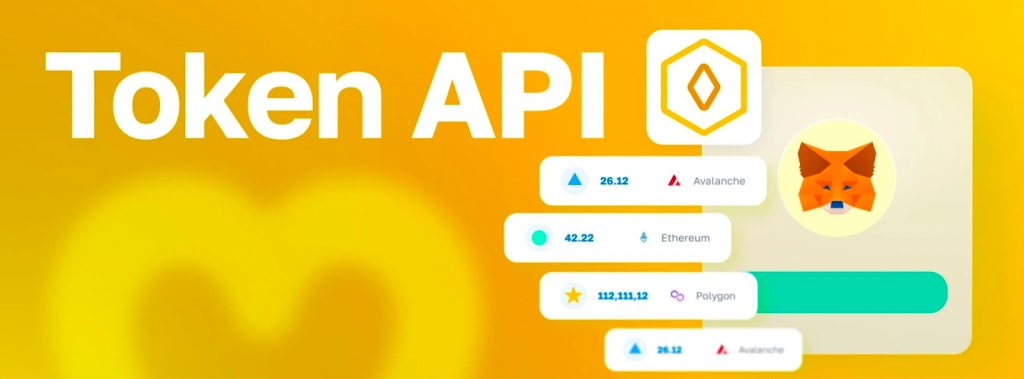
With the Token API, you can seamlessly integrate balances, prices, transfers, and much more into your dapps without breaking a sweat. What’s more, with our ERC20 Owners endpoint, you can get sorted holders of a token with a single line of code.
The ERC20 Owners endpoint simplifies the process of fetching accurate ERC20 owner data into a simple operation you can complete in a heartbeat. With this innovative feature, you can now fetch the top holders and total supply of any token, along with ownership percentages, the USD value of individual holdings, and more, with ease.
So, how does it work?
Well, to answer the above question, let’s dive into a short script calling our ERC20 Owners endpoint:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch( "https://deep-index.moralis.io/api/v2.2/erc20/0x7d1afa7b718fb893db30a3abc0cfc608aacfebb0/owners?chain=eth&order=DESC", options ) .then((response) => response.json()) .then((response) => console.log(response)) .catch((err) => console.error(err));
All you have to do is replace YOUR_API_KEY
with your Moralis API key, configure the parameters to fit your query and run the script. In return, you’ll get a response comprising an array of owners, including their total balance, the value of their holdings, and much more. Here’s an example of what the response can look like:
{ "cursor": "eyJhbGciOiJIUz//...", "page": 1, "page_size": 100, "result": [ { "balance": "3704449652939473320142469218", "balance_formatted": "3704449652.939473320142469218", "is_contract": false, "owner_address": "0x5e3ef299fddf15eaa0432e6e66473ace8c13d908", "owner_address_label": "Polygon (Matic): PoS Staking Contract", "usd_value": "3489442144.205111503601074219", "percentage_relative_to_total_supply": 37.044496529394735 }, //... ] }
The Benefits of Moralis’ ERC20 Owners Endpoint
Now that you have familiarized yourself with the ERC20 Owners endpoint, let’s dive a bit deeper into the benefits of this feature:
- Fewer API Calls: In the past, you needed to manually track ownership across the blockchain, which was a time-consuming and resource-intensive process. By consolidating ERC20 owner data into a ready-to-use endpoint, you now need far fewer API calls to get the data you need.
- Token Holder Insight: With the ERC20 Owners endpoint, you can seamlessly fetch the top holders of any token, significantly boosting transparency and the understanding of token distribution.
- Simplified Token Analytics: With the ERC20 Owners endpoint, you can now effortlessly complete complex analytics tasks. This includes identifying unique holders, ownership percentages, early adopters, newcomers, etc.
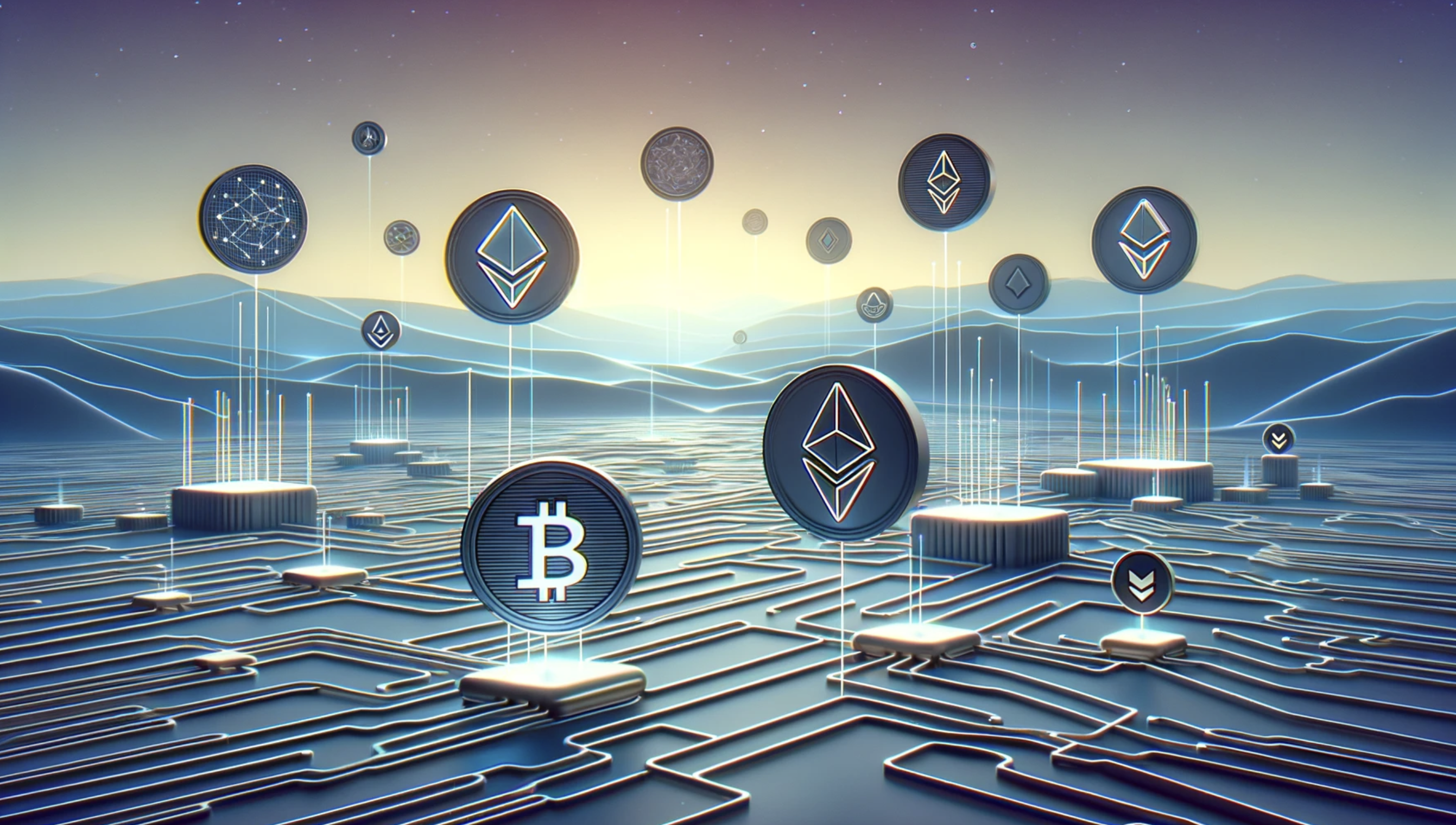
3-Step Tutorial: How to Get All Owners of an ERC20 Token with Moralis
We’ll now walk you through a simple tutorial on how to get sorted holders of a token using Moralis’ Token API. And thanks to the accessibility of this industry-leading tool, you’ll be able to get the data you need in three straightforward steps:
- Get a Moralis API Key
- Write a Script Calling the ERC20 Owners Endpoint
- Run the Code
However, before we can get going, you must deal with some prerequisites!
Prerequisites
This will be a JavaScript and Node.js tutorial. As such, if you haven’t already, make sure you set up the following before continuing:
- Node.js v.14+
- Npm/Yarn
Step 1: Get a Moralis API Key
To get a Moralis API key, you need to create an account by clicking on the ”Start for Free” button at the top right on the Moralis homepage:
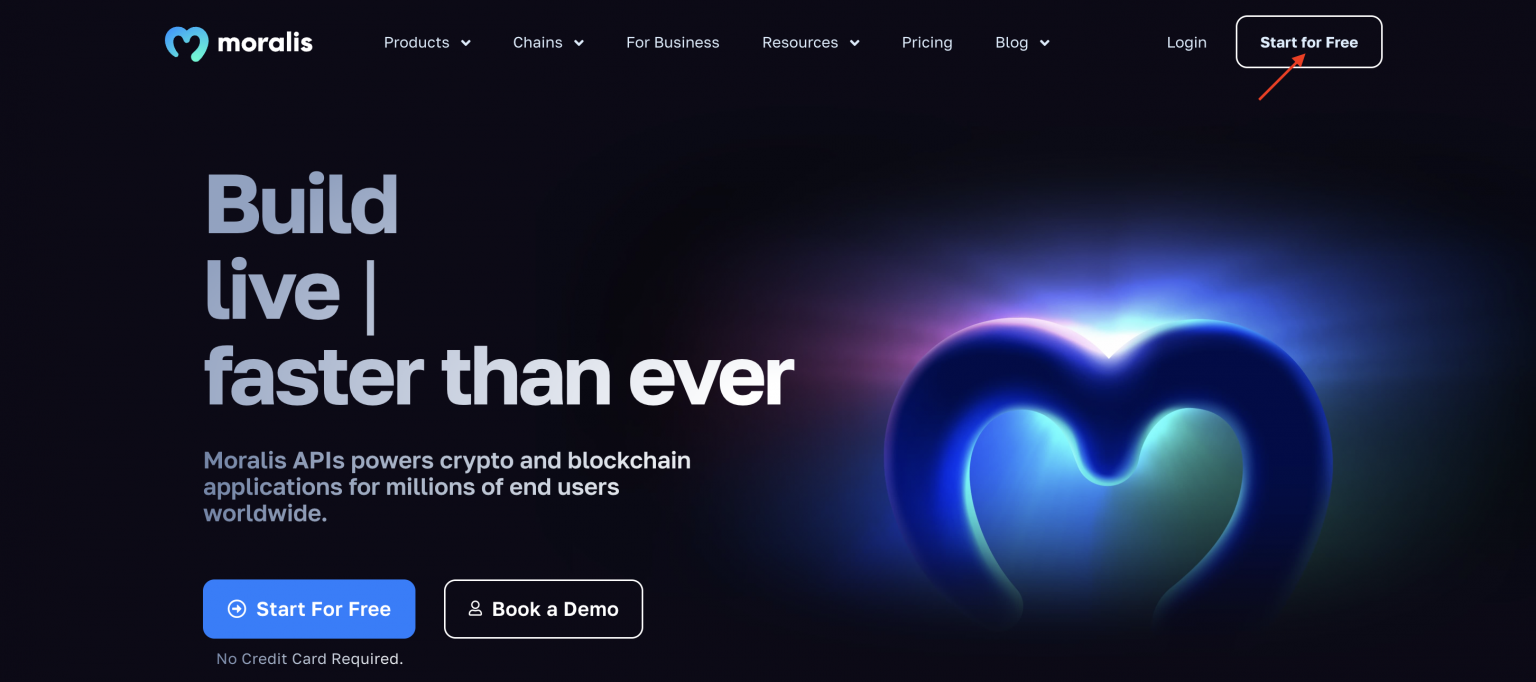
Once you’re done setting up your account, you need to log in, navigate to the ”Settings” tab, scroll down, and copy your key:
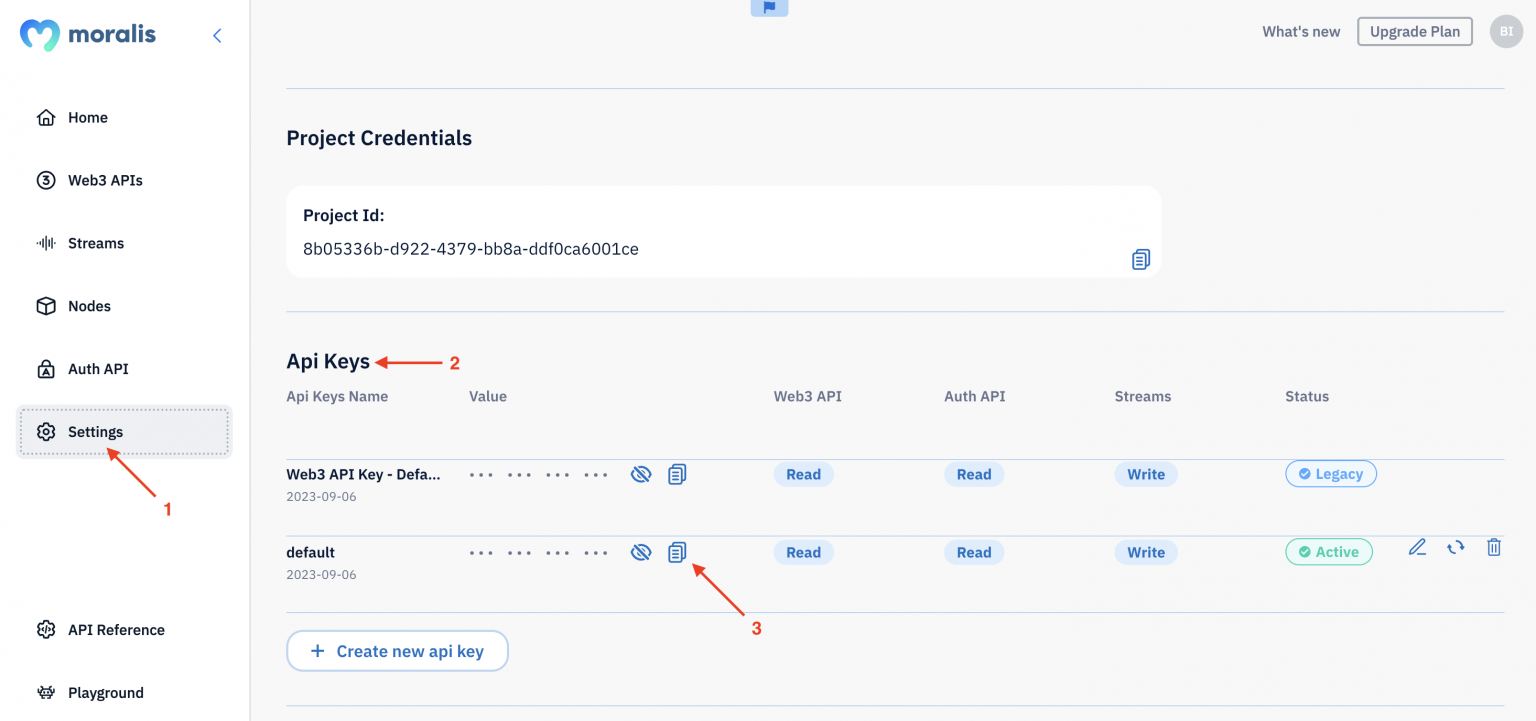
Keep the key for now. You’re going to need it in the following step to initialize Moralis.
Step 2: Write a Script Calling the ERC20 Owners
Endpoint
Start by opening your preferred IDE and set up a folder. Next, open a new terminal and initialize a project with the command below:
npm init
You then need to install the required dependencies with the following terminal inputs:
npm install node-fetch --save npm install moralis @moralisweb3/common-evm-utils
Next, open your ”package.json” file and add ”type”: ”module”
to the list:
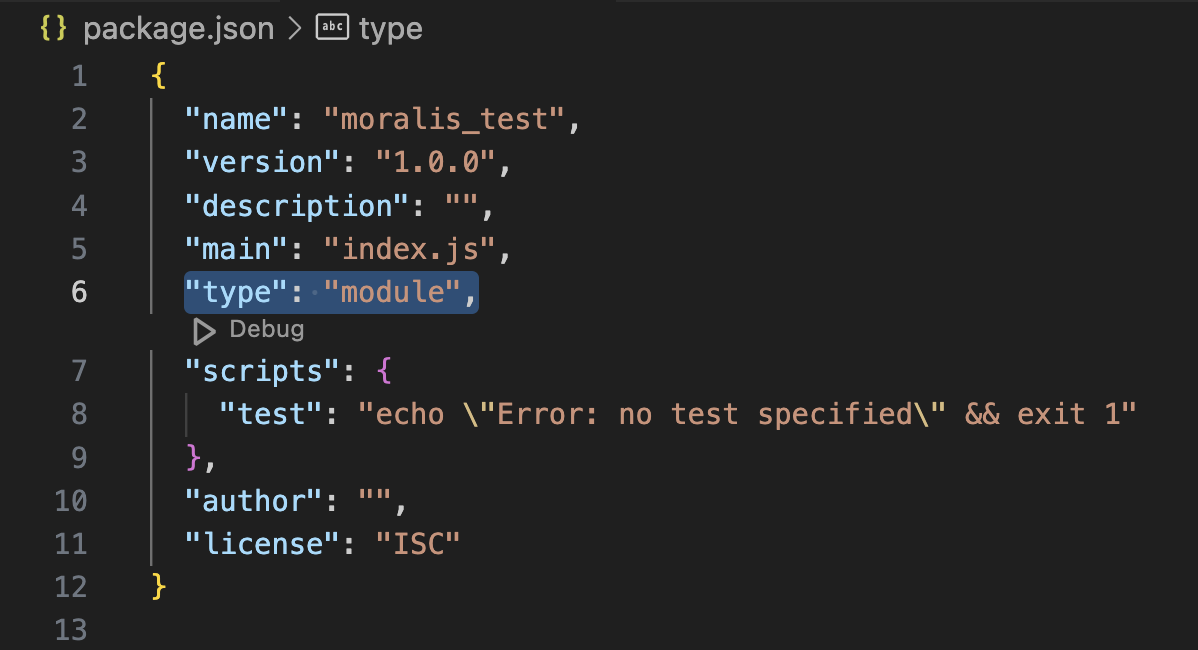
You can then create a new ”index.js” file and add the following contents:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch( "https://deep-index.moralis.io/api/v2.2/erc20/0x7d1afa7b718fb893db30a3abc0cfc608aacfebb0/owners?chain=eth&order=DESC", options ) .then((response) => response.json()) .then((response) => console.log(response)) .catch((err) => console.error(err));
From here, you need to configure the code slightly. First, replace YOUR_API_KEY
with the Moralis key you copied during the first step. Next, you also need to configure query parameters to fit your query.
That’s it for the code. All that remains from here is executing the script!
Step 3: Run the Code
You can execute the code by opening a new terminal and running the command below in the root folder of the project:
node index.js
In return, you’ll get a response containing all owners of the ERC20 token specified in the address parameter. Here’s an example of what it might look like:
{ "cursor": "eyJhbGciOiJIUz//...", "page": 1, "page_size": 100, "result": [ { "balance": "3704449652939473320142469218", "balance_formatted": "3704449652.939473320142469218", "is_contract": false, "owner_address": "0x5e3ef299fddf15eaa0432e6e66473ace8c13d908", "owner_address_label": "Polygon (Matic): PoS Staking Contract", "usd_value": "3489442144.205111503601074219", "percentage_relative_to_total_supply": 37.044496529394735 }, //... ] }
And that’s it; it doesn’t have to be more challenging than that to get sorted holders of a token when working with Moralis!
Use Cases for Our API and the ERC20 Owners Endpoint
Our ERC20 Owners endpoint is indispensable for most developers building dapps related to fungible tokens. However, to give you a few examples, let’s explore three prominent use cases for this feature:
- Token Analytics: Token analytics platforms are websites or applications that give users valuable insight into the performance of the market and individual tokens. A great example of a project leveraging our APIs is Moralis Money – the industry’s leading token analytics platform.
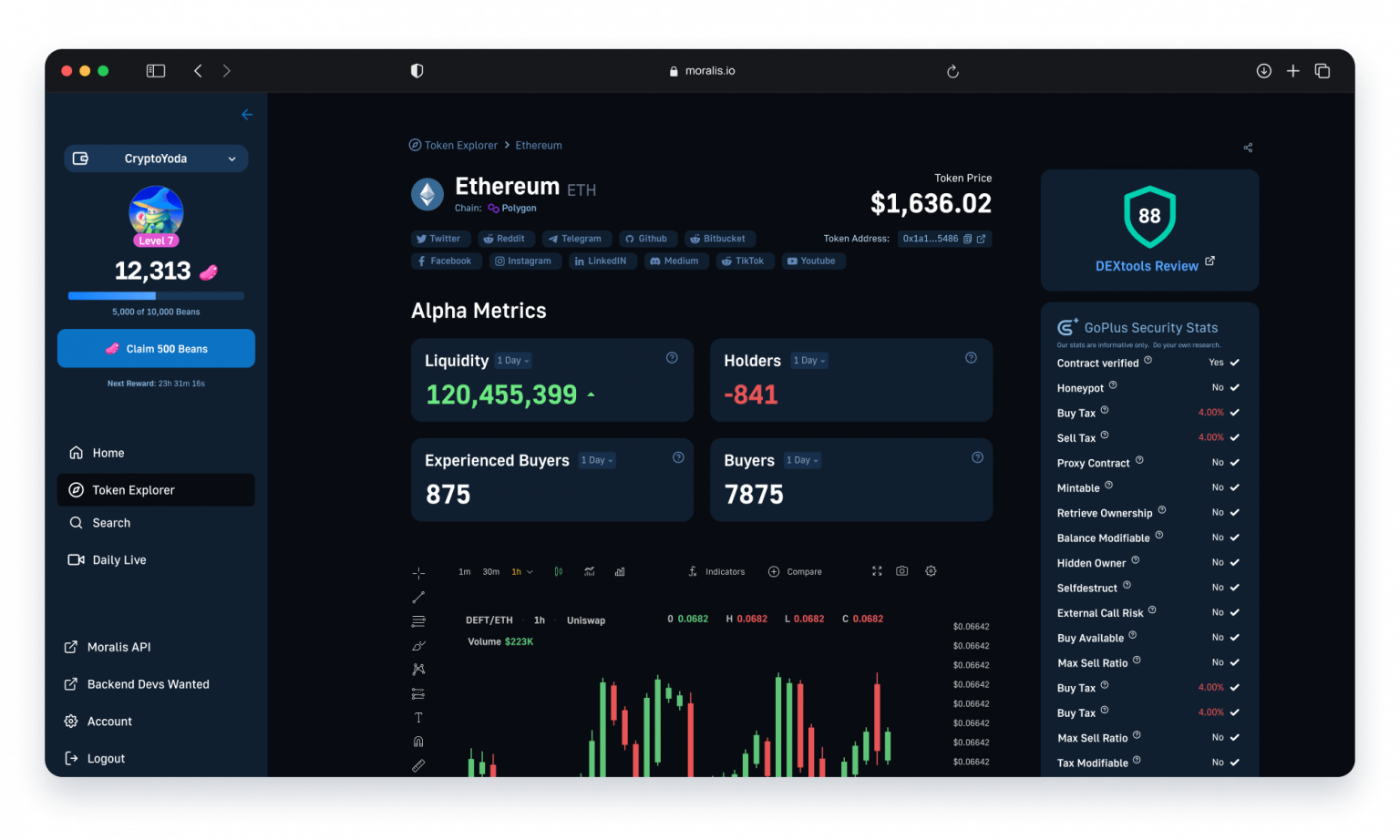
- Portfolio Trackers: Portfolio trackers are platforms that give users a complete overview of all their digital holdings. This typically includes both ERC20s and NFTs, as well as stocks and other digital assets. Delta is an excellent example of a portfolio tracker platform leveraging our APIs to keep users updated about recent on-chain events.
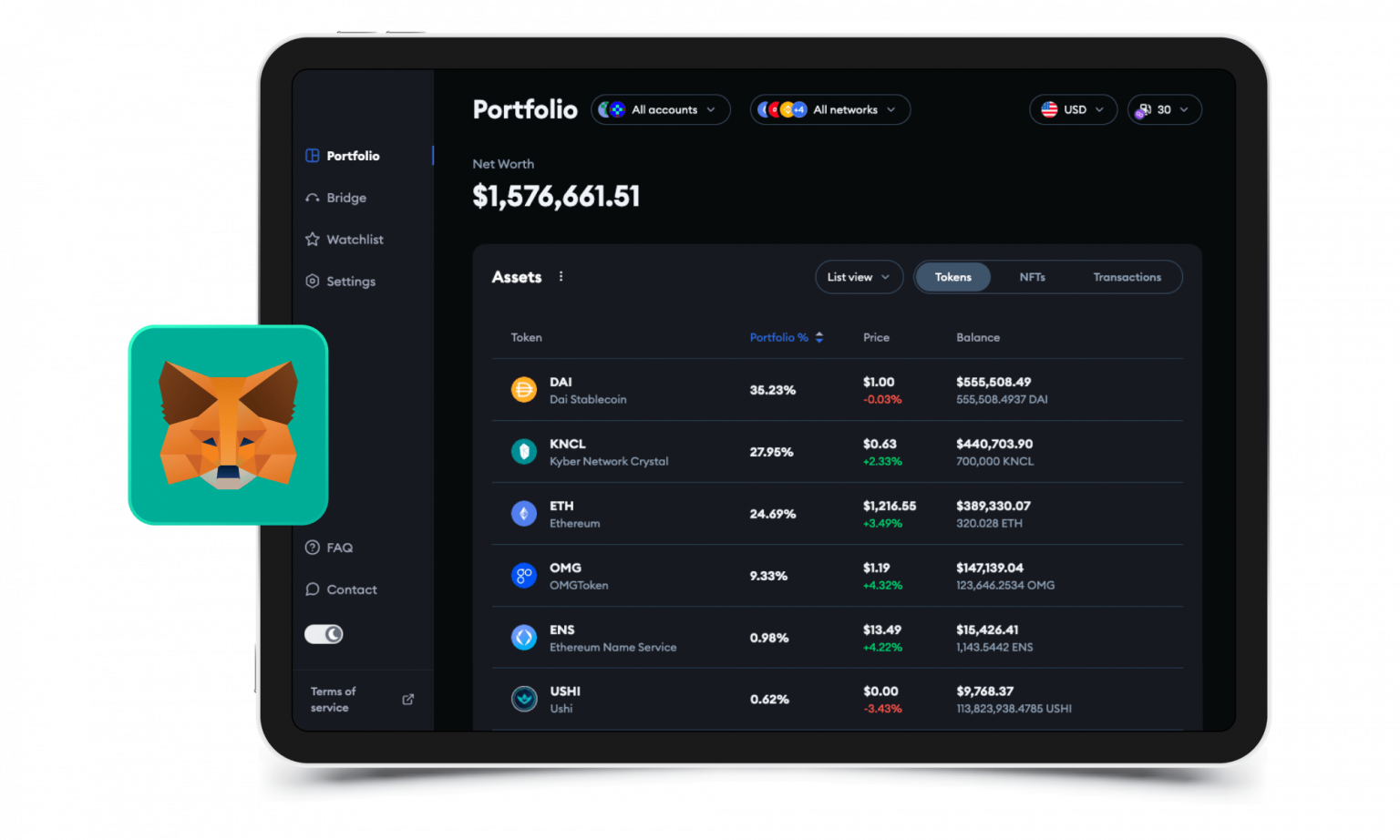
- Cryptocurrency Wallets: Cryptocurrency wallets are websites and applications for storing digital assets. The best wallets also provide features for buying, selling, and trading tokens. A prominent example of a provider using Moralis is MetaMask – the industry’s #1 cryptocurrency wallet.
Beyond the Token API – Exploring Moralis Further
The Token API only scratches the surface of what’s possible with Moralis. As such, let’s now explore some additional APIs you’ll likely find helpful when building your dapps. However, we won’t be able to cover them all in this guide, which is why we’ll focus on the following three interfaces:
- Wallet API
- NFT API
- Blockchain API
If you’d like to explore all our APIs, please visit the official Web3 API page, which includes a list of all available interfaces!
Wallet API
The Wallet API is the industry’s #1 API for wallet data, boasting an impressive array of features, unparalleled scalability, and exceptional flexibility. It’s the perfect tool if you’re looking to build your own crypto wallet or integrate wallet functionalities into your dapp!
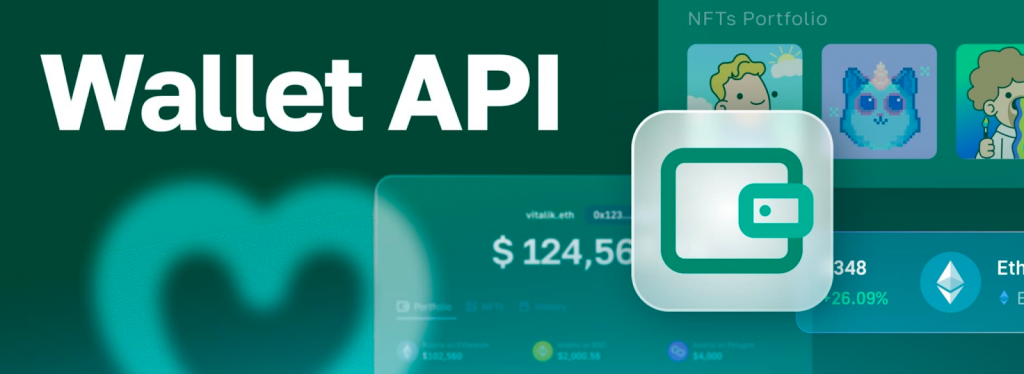
With the Wallet API, you can seamlessly fetch a wallet’s ERC20 balances, net worth, transaction history, and much more with a single line of code. To show you how it works, we’ll now look at an example of how easy it is to fetch the ERC20 balances – with prices and metadata – of a wallet using our getWalletTokenBalancesPrice()
endpoint:
import Moralis from 'moralis'; try { await Moralis.start({ apiKey: "YOUR_API_KEY" }); const response = await Moralis.EvmApi.wallets.getWalletTokenBalancesPrice({ "chain": "0x1", "address": "0xcB1C1FdE09f811B294172696404e88E658659905" }); console.log(response.raw()); } catch (e) { console.error(e); }
Calling the endpoint above returns an array of all tokens the wallet holds. What’s more, the response is enriched with prices and metadata, allowing you to, for instance, build a portfolio view with a single endpoint:
{ "cursor": null, "page": 0, "page_size": 100, "result": [ { "token_address": "0xae7ab96520de3a18e5e111b5eaab095312d7fe84", "symbol": "stETH", "name": "Liquid staked Ether 2.0", "logo": "https://logo.moralis.io/0x1_0xae7ab96520de3a18e5e111b5eaab095312d7fe84_cd0f5053ccb543e08f65554bf642d751", "thumbnail": "https://logo.moralis.io/0x1_0xae7ab96520de3a18e5e111b5eaab095312d7fe84_cd0f5053ccb543e08f65554bf642d751", "decimals": 18, "balance": "90399378678107406", "possible_spam": false, "verified_contract": true, "total_supply": "9542023372380838741270859", "total_supply_formatted": "9542023.372380838741270859", "percentage_relative_to_total_supply": 9.47381652195e-7, "balance_formatted": "0.090399378678107406", "usd_price": 3611.562488280326, "usd_price_24hr_percent_change": 4.98306233529165, "usd_price_24hr_usd_change": 15.496644212196657, "usd_value": 326.483004997701, "usd_value_24hr_usd_change": 16.268851653168795, "native_token": false, "portfolio_percentage": 55.2363 }, //... ] }
To explore other capabilities of this tool, check out our official Wallet API page!
NFT API
Moralis’ NFT API is Web3’s premier interface for NFT data. This leading tool supports over three million NFT collections across all the biggest chains, including everything from established projects like Nakamigos to tokens that dropped just seconds ago!
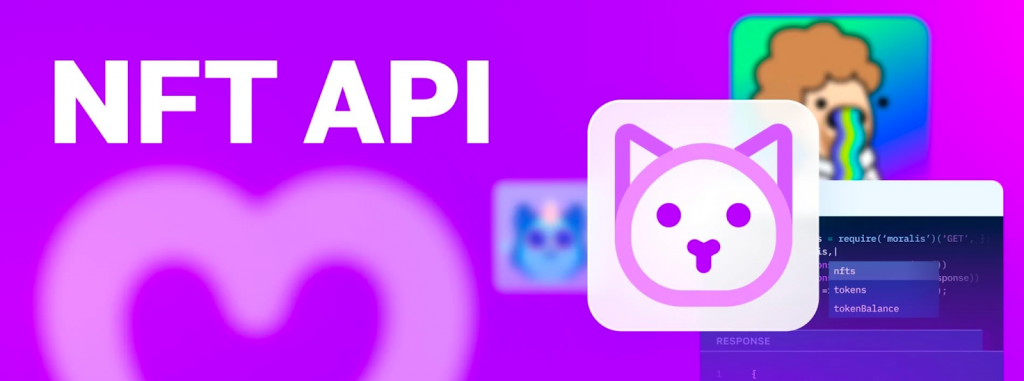
With the NFT API, you can seamlessly fetch metadata, balances, optimized image previews, and much more with single endpoints. As such, you can build everything from marketplaces to Web3 games without breaking a sweat when using the NFT API.
To highlight the accessibility of the NFT API, we’ll now show you how to fetch the balance of any wallet with our getWalletNFTs()
endpoint:
import Moralis from 'moralis'; try { await Moralis.start({ apiKey: "YOUR_API_KEY" }); const response = await Moralis.EvmApi.nft.getWalletNFTs({ "chain": "0x1", "address": "0xff3879b8a363aed92a6eaba8f61f1a96a9ec3c1e" }); console.log(response.raw); } catch (e) { console.error(e); }
In return for calling the endpoint above, you’ll get an array of all NFTs held by the wallet in question. Here’s an example of what the response might look like:
{ "status": "SYNCED", "page": 1, "page_size": 100, "cursor": "eyJhbGciOiJIUzI1Ni//...", "result": [ { "amount": "1", "token_id": "5021", "token_address": "0xfff54e6fe44fd47c8814c4b1d62c924c54364ad3", "contract_type": "ERC721", "owner_of": "0xff3879b8a363aed92a6eaba8f61f1a96a9ec3c1e", "last_metadata_sync": "2024-04-09T11:27:46.910Z", "last_token_uri_sync": "2024-04-09T11:27:46.896Z", "metadata": null, "block_number": "14647390", "block_number_minted": "14647390", "name": "Youtopia", "symbol": "Youtopia", "token_hash": "d4719eaf84eabcf443065b0a463f5886", "token_uri": "http://api.youtopia-official.xyz/ipfs/5021", "minter_address": "0x13f11fd2c7c7be94674651386370d02b7aac9653", "verified_collection": false, "possible_spam": true, "collection_logo": "", "collection_banner_image": "" }, //... ] }
Please check out our NFT API page to learn more about this interface!
Blockchain API
The Blockchain API lets you unlock the power of raw blockchain data with pace and precision. It is scalable, precise, and cross-chain, giving you what you need to build everything from block explorers to analytics tools!
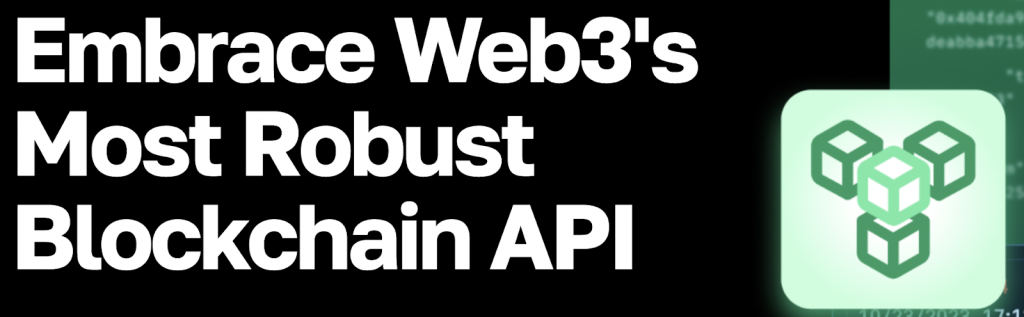
With the Blockchain API, you can seamlessly fetch block data, transactions, smart contract logs, and more with single lines of code. To show you how it works, let’s look at our getBlock()
endpoint, allowing you to fetch the contents of any given block:
import Moralis from 'moralis'; try { await Moralis.start({ apiKey: "YOUR_API_KEY" }); const response = await Moralis.EvmApi.block.getBlock({ "chain": "0x1", "blockNumberOrHash": "18541416" }); console.log(response.raw); } catch (e) { console.error(e); }
In return for calling the endpoint above, you’ll get the block’s hash, miner address, gas limit, and more. Here’s an example of what the response will look like:
{ "timestamp": "2023-11-10T12:02:11.000Z", "number": "18541416", "hash": "0xe9de65582d5a210f3f5703e0d7dc1daf9fbb5a0670937f5d92283a41ea1bcb2b", "parent_hash": "0xb63af7df0e2639cc25c323f22359b784408a117b534dea09cfec14263ea8d4fb", "nonce": "0x0000000000000000", "sha3_uncles": "0x1dcc4de8dec75d7aab85b567b6ccd41ad312451b948a7413f0a142fd40d49347", "logs_bloom": "0x00200000410//...", "transactions_root": "0x0b6895fbd7fdf9b8e0239e5be21a195c89ffae093c1c2bcdea9ad498d720003b", "state_root": "0x787cc0fff225d2b6d8acef1cea33522293d134297c1f4b22819a90f157db183d", "receipts_root": "0x6d7caa30f12937a232dcedda5f8bc2c973ba917a67062cedeb4ccb52c729058c", "miner": "0x95222290DD7278Aa3Ddd389Cc1E1d165CC4BAfe5", "difficulty": "0", "total_difficulty": "58750003716598352816469", "size": "7792", "extra_data": "0x6265617665726275696c642e6f7267", "gas_limit": "30000000", "gas_used": "1372772", "transaction_count": "11", "base_fee_per_gas": "35229184857", "transactions": [ //... ] }
Check out our Blockchain API page to learn more about this premier interface!
Summary: How to Get All Owners of an ERC20 Token
From a conventional perspective, fetching the owners of an ERC20 token has been an annoying task. It typically requires manually tracking ownership and transactions across the blockchain, which is both tedious and time-consuming. Fortunately, it’s now possible to circumvent this entirely with Moralis’ ERC20 Owners endpoint!
With our ERC20 Owners endpoint, you can now get sorted holders of a token with a single API call. In addition to the owners, you also get ownership percentages, the USD value of individual holdings, and much more within the same response. And with this premier feature, we’re reducing the barriers to complex data analytics, making token ownership data more accessible to everyone!
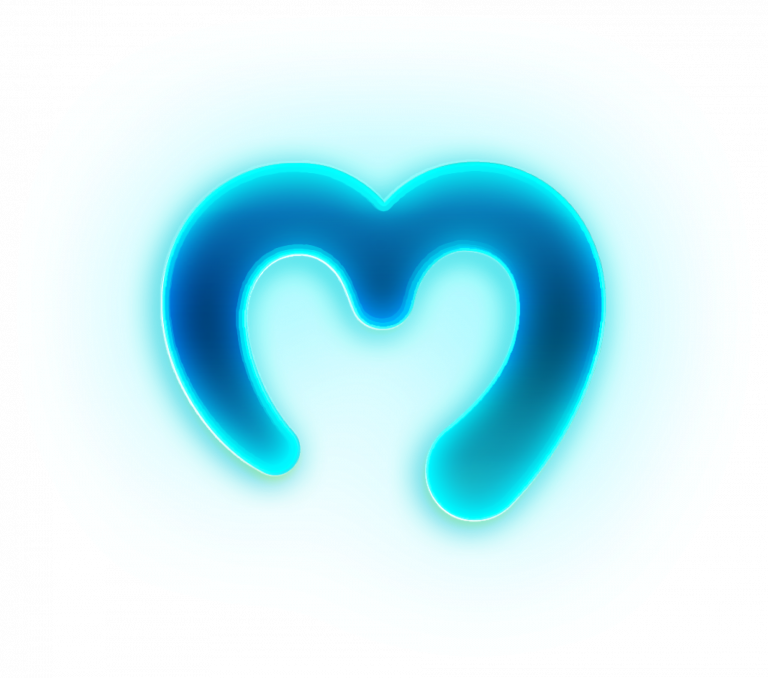
If you liked this tutorial on how to get all owners of an ERC20 token, consider reading more content here at Moralis. For instance, check out our most recent article on the best crypto logo API or learn how to get the net worth of any ERC20 wallet.
Also, don’t forget that you can sign up with Moralis free of charge. So, take this opportunity to supercharge your Web3 projects with streamlined data integration from Moralis!