Do you want to get and integrate the net worth of any wallet into your decentralized applications (dapps)? With Moralis’ Wallet Net Worth endpoint, it’s now easier than ever. All you need is a single API call to get the total net worth of any ERC20 crypto wallet across most blockchains!
If you’re eager to dive straight into the code, check out the example below, where we write a short script for calling the endpoint to get ERC20 wallet net worth data:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch('https://deep-index.moralis.io/api/v2.2/wallets/0xd8da6bf26964af9d7eed9e03e53415d37aa96045/net-worth?chains%5B0%5D=eth&chains%5B1%5D=polygon&exclude_spam=true&exclude_unverified_contracts=true', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
In return for running the code above, you’ll get the total net worth of the specified ERC20 wallet. You’ll also get the individual values for each chain included as parameters of your query. Here’s an example of what the response might look like:
{ "total_networth_usd": "4286806.08", "chains": [ { "chain": "eth", "native_balance": "1085515469813080189177", "native_balance_formatted": "1085.515469813080189177", "native_balance_usd": "3550067.16", "token_balance_usd": "735008.04", "networth_usd": "4285075.20" }, { "chain": "polygon", "native_balance": "426857449018746625825", "native_balance_formatted": "426.857449018746625825", "native_balance_usd": "445.31", "token_balance_usd": "1285.57", "networth_usd": "1730.88" } ] }
If you’d like to learn more about this premier endpoint, check out the official Wallet Net Worth documentation page, watch the video below, or join us in this guide!
Also, if you wish to follow along in this tutorial, don’t forget to sign up with Moralis. You can create an account free of charge, and you’ll gain instant access to our top-tier Web3 API suite!
Leading Web3 APIs?
Overview
Whether you’re building or looking to scale a cryptocurrency wallet, portfolio tracker, or any other platform integrating wallet components in some capacity, you need a seamless way to get the net worth of any address. But what is the easiest way to get the net worth of an ERC20 crypto wallet via API?
In this tutorial, we’ll show you how to fetch the net worth of a crypto address using Moralis’ industry-leading Wallet API. With this top-tier API and the Wallet Net Worth endpoint, you can seamlessly get the data you need with only a few lines of code. So, if you want to build or scale a cryptocurrency wallet, portfolio tracker, or any other Web3 platform, join us throughout this guide. Now, let’s dive straight in!
Exploring Crypto Wallet Net Worth
The net worth of a cryptocurrency wallet refers to the total value of all assets stored in the portfolio, including native, ERC20, and non-fungible tokens (NFTs). This is an important metric that cryptocurrency wallets, tax tools, portfolio trackers, and other projects integrate into their platforms!
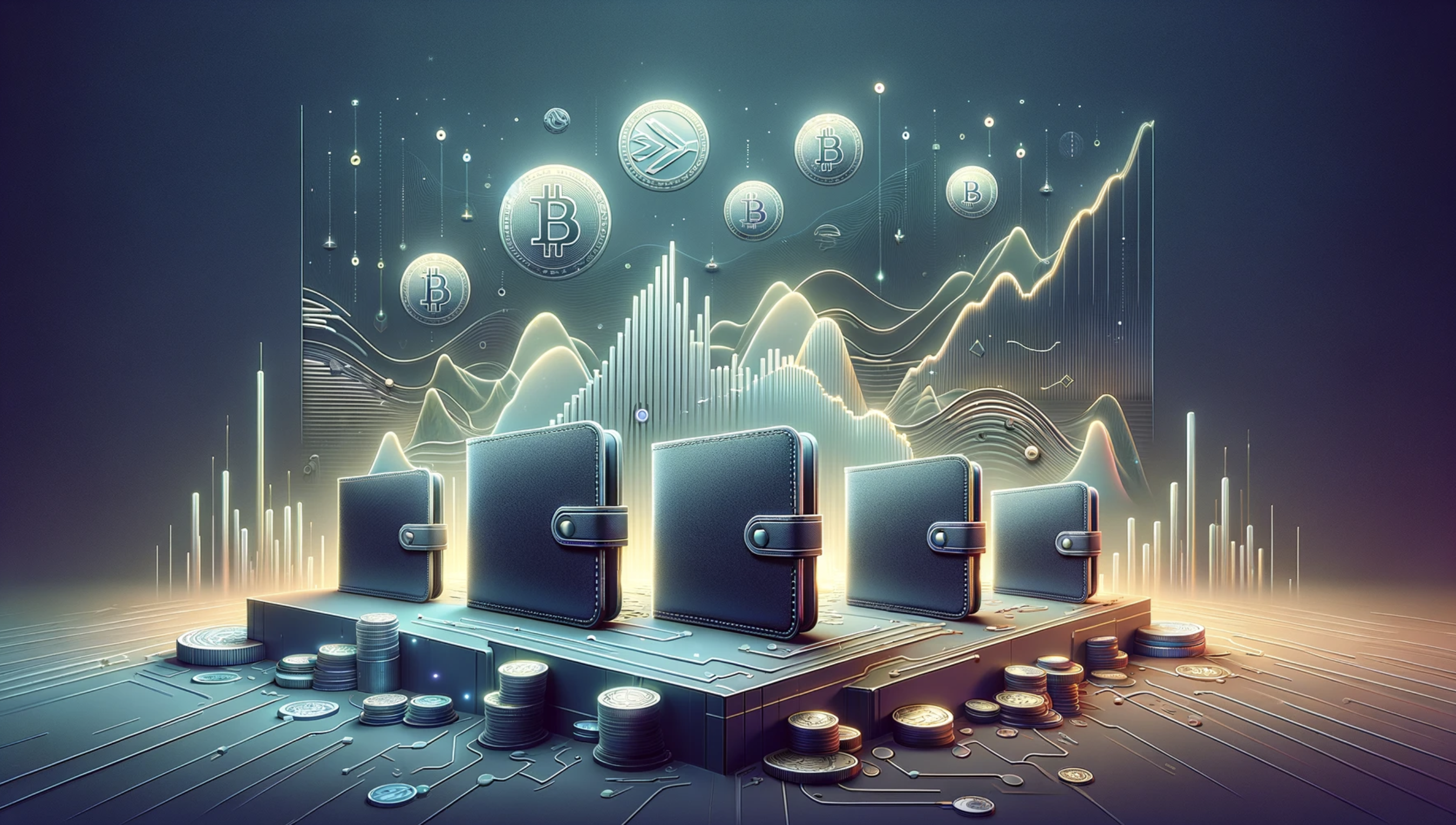
Calculating the net worth of a wallet is a tedious and time-consuming task. It typically involves manually collecting data across multiple blockchain networks, resulting in separate calls for ERC20 token balances, native balances, prices, and not to mention NFTs. The result of this is a complex workflow that demands a lot of development time and can lead to thousands of thousands of API calls.
But there has to be a better way, right?
To streamline this process, we introduced the Wallet Net Worth endpoint to Moralis’ Wallet API. With this endpoint, you can now seamlessly and accurately get ERC20 wallet net worth data and automatically calculate the net worth with just one single API call!
So, how does this work?
Get ERC20 Wallet Net Worth with a Single API Call
With Moralis’ Wallet Net Worth endpoint, you can now effortlessly and accurately get the wallet net worth of any address with a few lines of code. To showcase the accessibility of working with our APIs, check out the simple script below for calling the endpoint in question:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch('https://deep-index.moralis.io/api/v2.2/wallets/0xd8da6bf26964af9d7eed9e03e53415d37aa96045/net-worth?chains%5B0%5D=eth&chains%5B1%5D=polygon&exclude_spam=true&exclude_unverified_contracts=true', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
All you have to do before running the script above yourself is to install the required dependencies, replace YOUR_API_KEY
, configure the address parameter, specify the chains you wish to include, and run the script.
In the query above, we set both exclude_spam
and exclude_unverified_contracts
to true
. That way, we can remove spam tokens and unverified contracts from the response.
In return for running the script above, you’ll get a response that looks something like this:
{ "total_networth_usd": "4286806.08", "chains": [ { "chain": "eth", "native_balance": "1085515469813080189177", "native_balance_formatted": "1085.515469813080189177", "native_balance_usd": "3550067.16", "token_balance_usd": "735008.04", "networth_usd": "4285075.20" }, { "chain": "polygon", "native_balance": "426857449018746625825", "native_balance_formatted": "426.857449018746625825", "native_balance_usd": "445.31", "token_balance_usd": "1285.57", "networth_usd": "1730.88" } ] }
As you can see, this simple yet sophisticated response includes both the total net worth of the ERC20 wallet and separate metrics for each chain specified in the query. From here, you can now seamlessly integrate this data into any of your projects!
Why Do You Need the ERC20 Wallet Net Worth Endpoint?
Since you can leverage the Wallet Net Worth endpoint to avoid the tedious and time-consuming task of manually calculating the net worth of any wallet, it provides a lot of benefits to Web3 developers. Below, we’ll briefly explore three prominent examples:
- Effortless Net Worth Calculations: You can now effortlessly fetch the net worth of any wallet, removing the need for manual data aggregation.
- Accurate Price Data: Moralis provides up-to-date, real-time price data, ensuring precise portfolio assessments.
- Reduced Complexities: With the Wallet Net Worth endpoint, you don’t have to manually fetch and calculate the total value of a wallet. As such, this endpoint facilitates a more accessible developer experience, resulting in fewer API calls and a much simpler workflow.
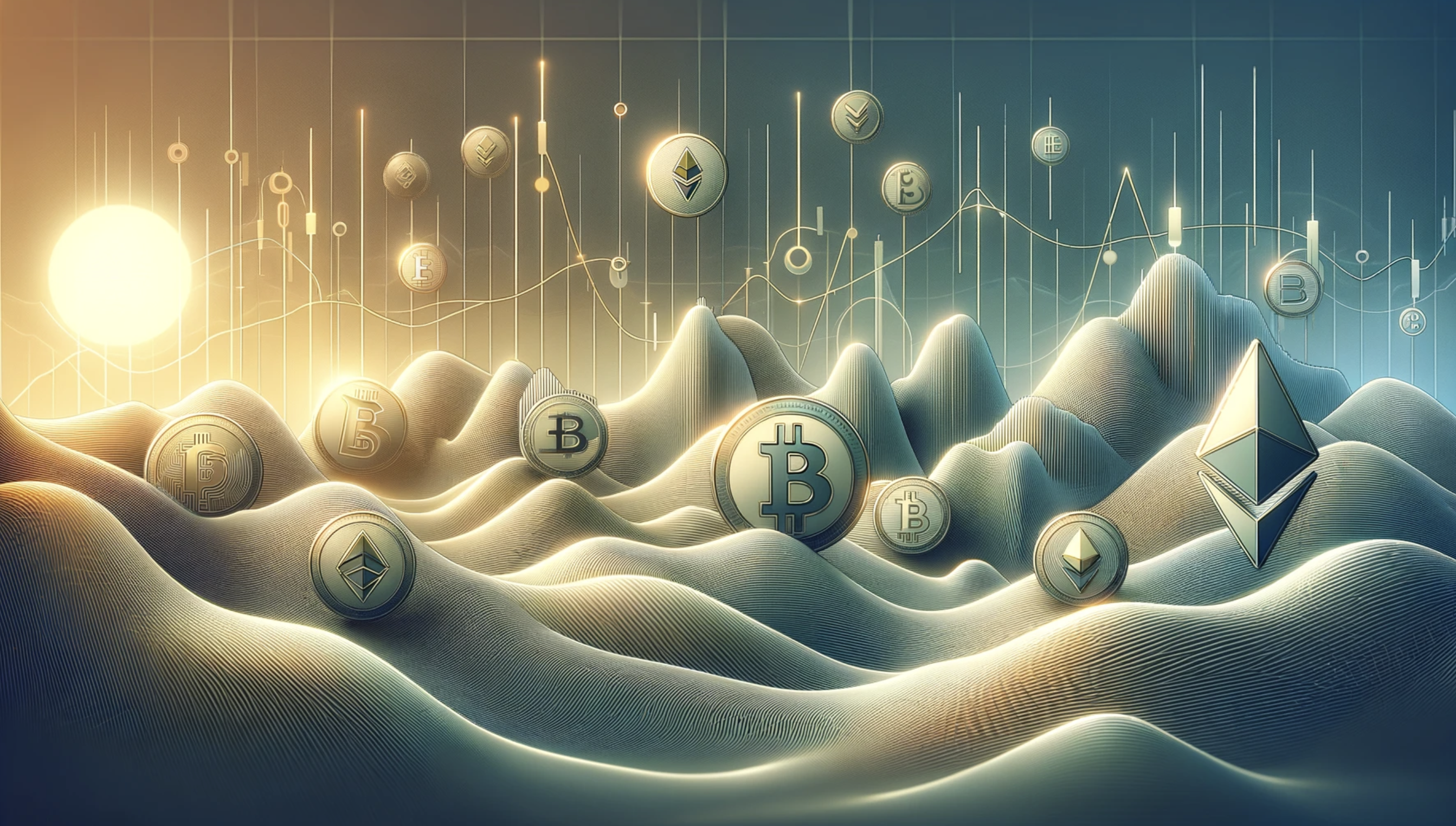
By launching this endpoint, we’re empowering developers worldwide to do more faster and with fewer API calls. As such, the Wallet Net Worth endpoint frees up a lot of time, enabling you and your team to focus on what’s really important: building a compelling user experience and increasing customer value.
In-Depth Tutorial: How to Get the Net Worth of Any ERC20 Wallet in 3 Steps
With an overview of our premier endpoint, we’ll now show you how to get a wallet’s ERC20 net worth in three simple steps:
- Step 1: Fetch Your Moralis API Key
- Step 2: Write a Script Calling the Wallet Net Worth Endpoint
- Step 3: Run the Code
However, before we can proceed, you need to take care of a few essential prerequisites!
Prerequisites
In today’s tutorial, we’ll be using JavaScript and Node.js. As such, if you haven’t already, make sure you have the following ready:
- Node.js v14+
- Npm/Yarn
Step 1: Fetch Your Moralis API Key
The first thing you’ll need is a Moralis account. As such, start by signing up with Moralis by clicking on the sign-up button at the top right of Moralis’ homepage:
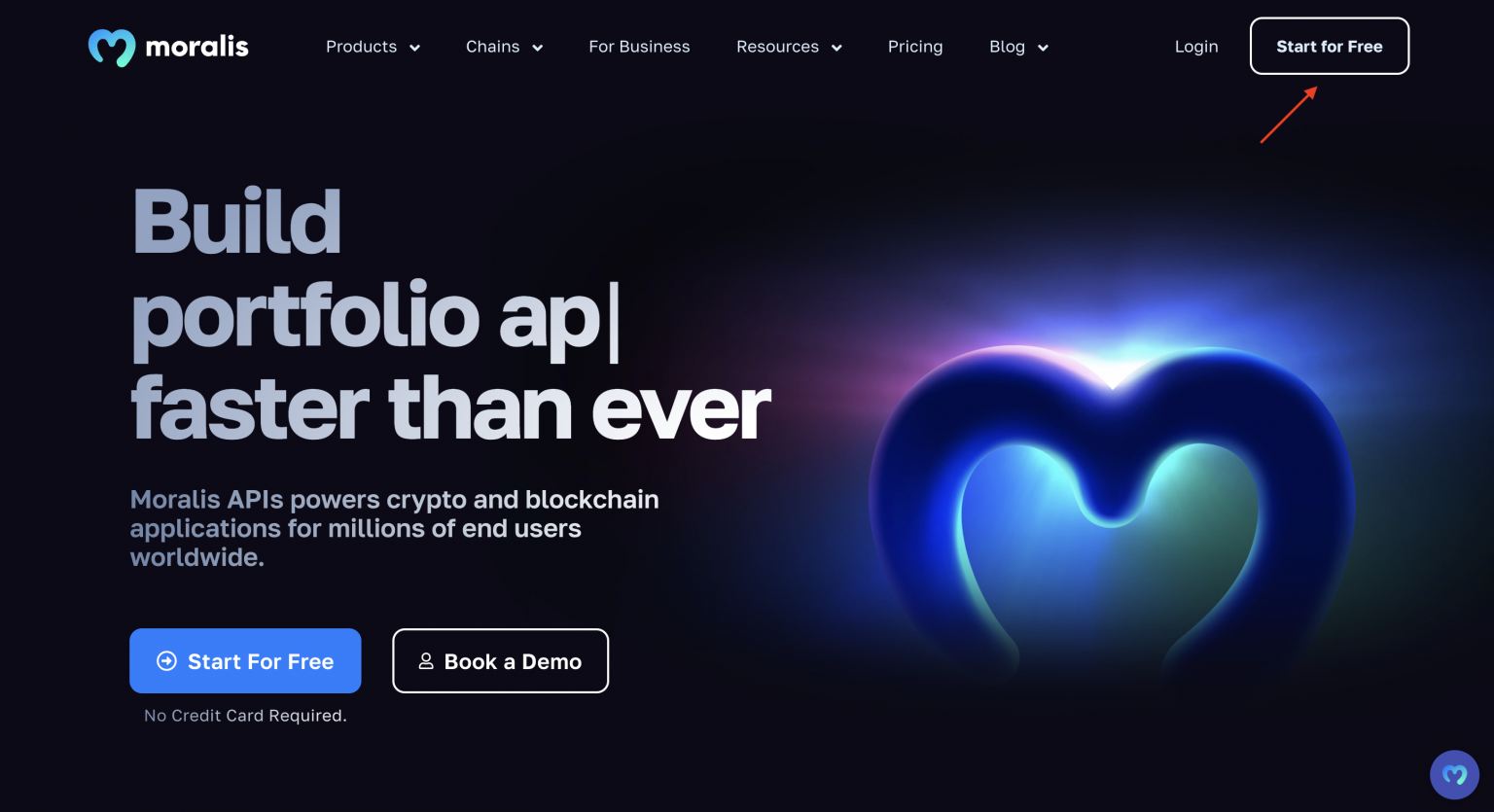
Next, go to the ”Settings” tab, find the ”API Keys” section, and copy your key:
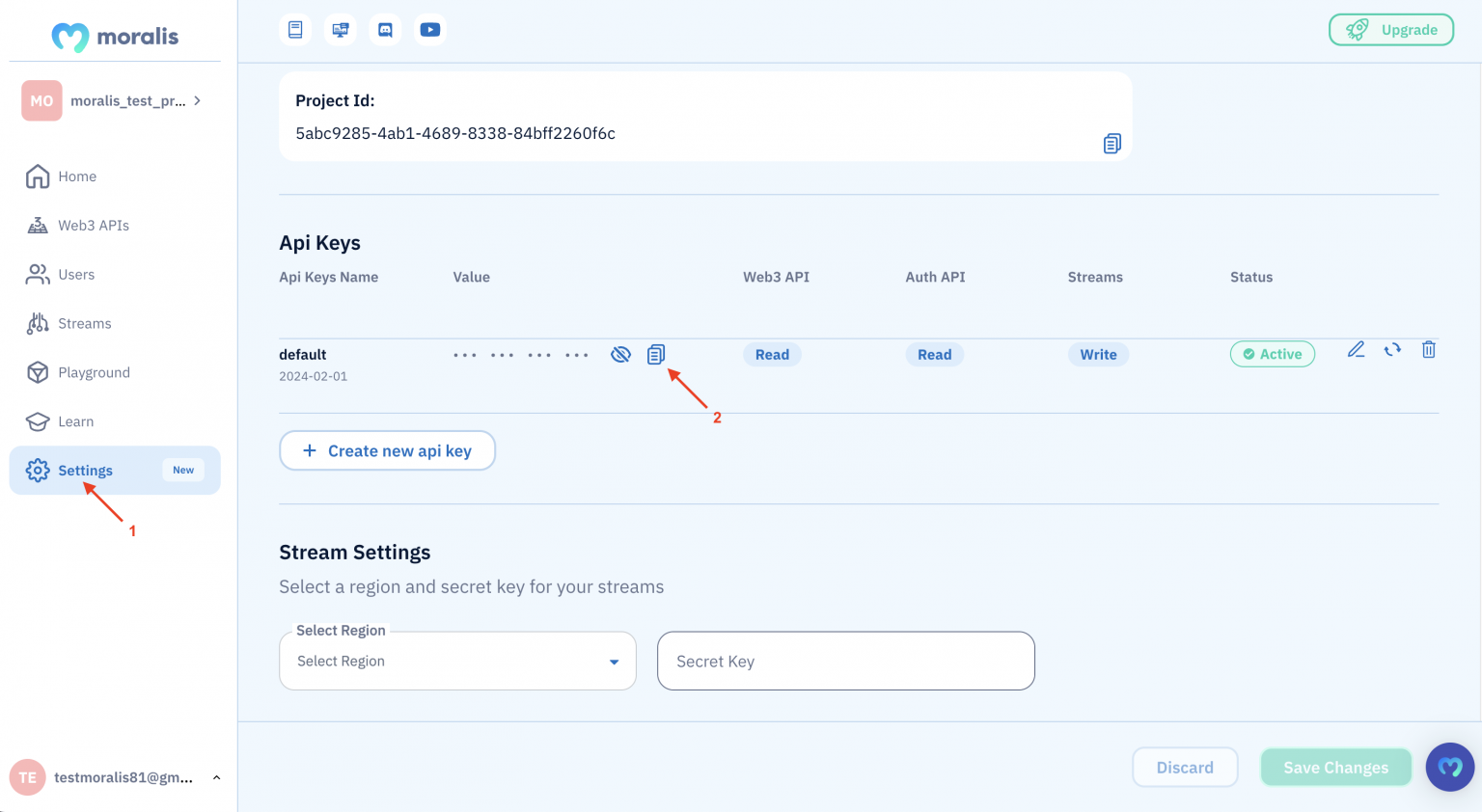
Please save it for now, as you’ll need it in the next section.
Step 2: Write a Script Calling the Wallet Net Worth Endpoint
Open your preferred IDE and set up a folder. Next, launch a new terminal and run the following command to initialize a project:
npm init
Run the following commands in the terminal to install the required dependencies:
npm install node-fetch --save npm install moralis @moralisweb3/common-evm-utils
You can then open your ”package.json” file and add ”type”: ”module”
to the list:
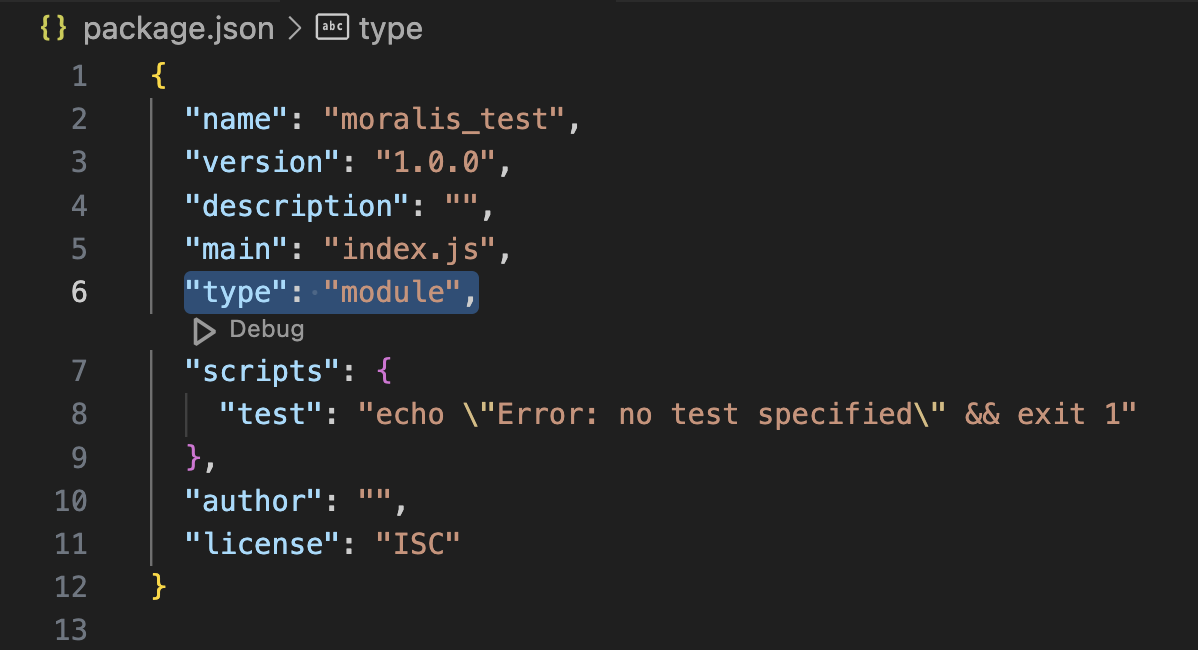
Next, create an ”index.js” file and add this code:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch('https://deep-index.moralis.io/api/v2.2/wallets/0xd8da6bf26964af9d7eed9e03e53415d37aa96045/net-worth?chains%5B0%5D=eth&chains%5B1%5D=polygon&exclude_spam=true&exclude_unverified_contracts=true', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
From here, you will need to configure the code slightly. Start by adding your Moralis API key by replacing YOUR_API_KEY
:
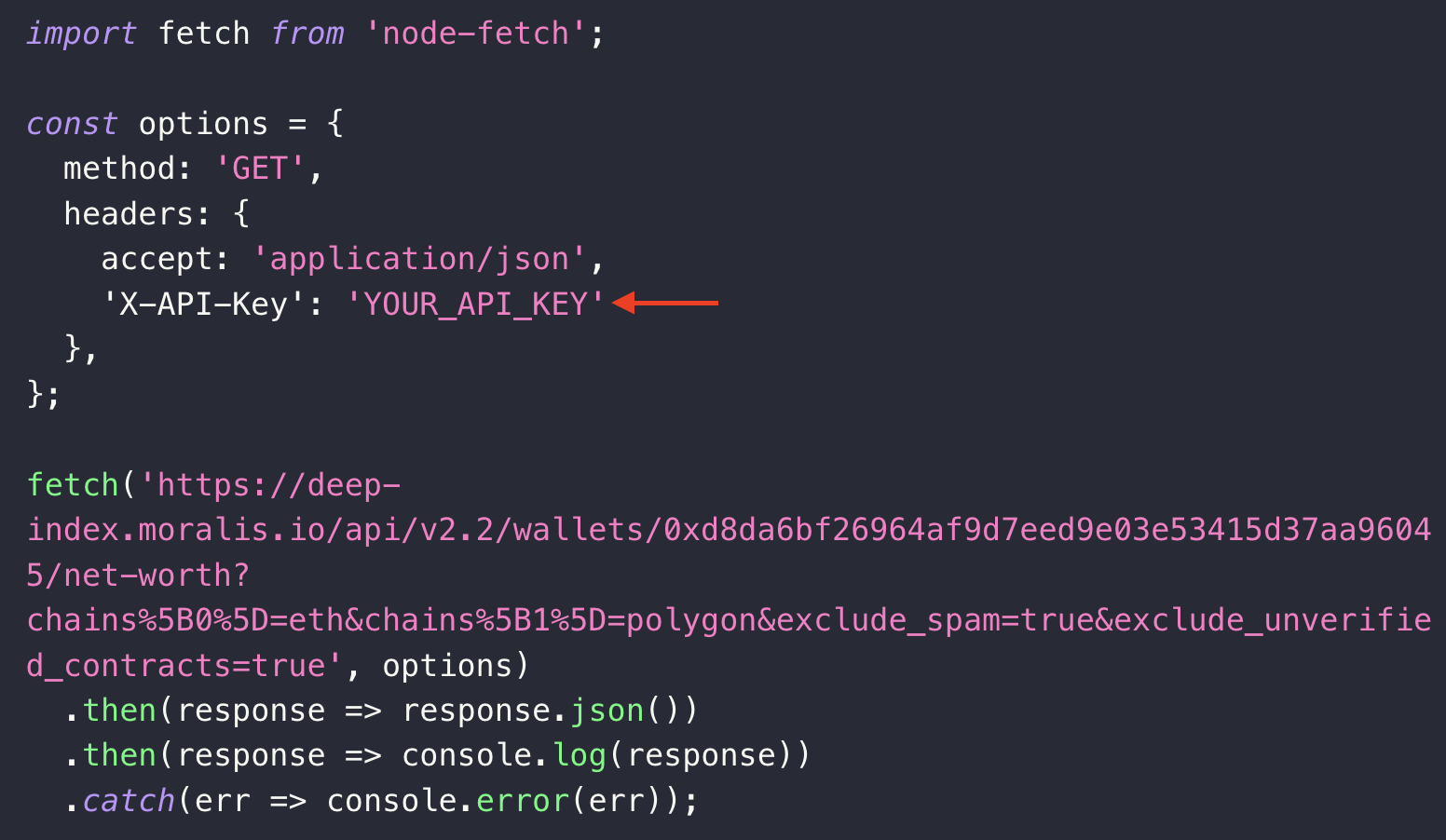
Then, you can also configure the parameters of your query. For instance, you can swap the address if you wish to query another wallet, and you can add additional chains if you want to include tokens from other networks:
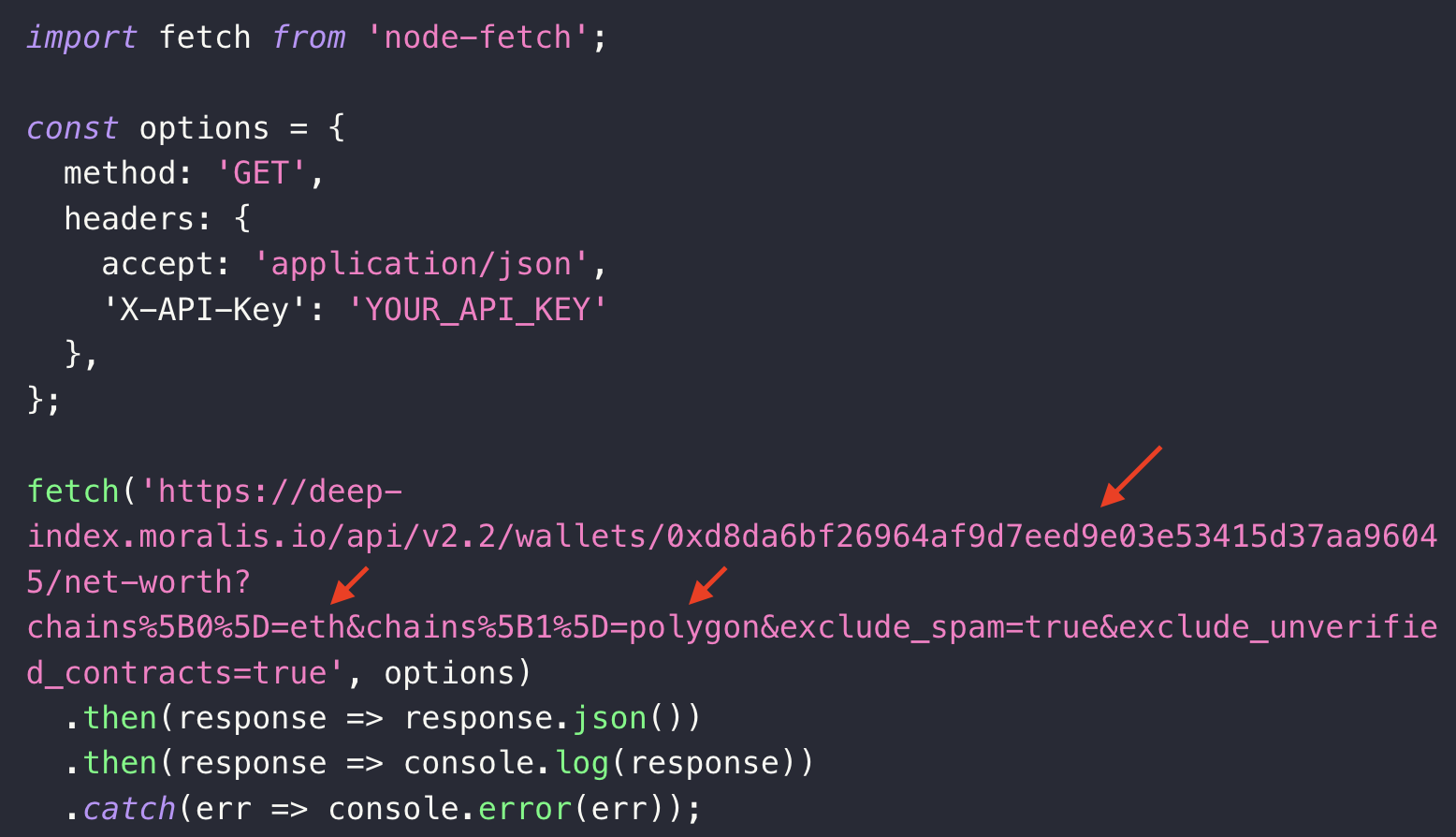
Also, note that we include exclude_spam
and exclude_unverified_contracts
as parameters and set them to true
. That way, our results will exclude spam tokens and non-verified contracts.
That’s it; you’re now ready to run the code!
Step 3: Run the Code
Open a new terminal and run this command in the root folder of the project to execute the code:
node index.js
In return, you’ll get a response including the total net worth of the wallet, along with the separate values for each chain included in the query. Here’s an example of what it might look like:
{ "total_networth_usd": "4286806.08", "chains": [ { "chain": "eth", "native_balance": "1085515469813080189177", "native_balance_formatted": "1085.515469813080189177", "native_balance_usd": "3550067.16", "token_balance_usd": "735008.04", "networth_usd": "4285075.20" }, { "chain": "polygon", "native_balance": "426857449018746625825", "native_balance_formatted": "426.857449018746625825", "native_balance_usd": "445.31", "token_balance_usd": "1285.57", "networth_usd": "1730.88" } ] }
With this data, you can now seamlessly get ERC20 wallet net worth data and integrate it into your projects!
Wallet Net Worth Use Cases: Why Do You Need to Get the Net Worth of an ERC20 Wallet?
The Wallet Net Worth endpoint has a lot of utility and will be useful for anyone building dapps that feature some sort of wallet component. However, to give you some examples, let’s explore three prominent use cases below!
- Cryptocurrency Wallets: Cryptocurrency wallets are software that allows users to store their digital assets, including NFTs and fungible tokens. The most prominent wallets also provide functionality for buying, selling, and trading crypto. As such, they give users the tools to manage their assets fully. Some notable examples of cryptocurrency wallets include MetaMask, Coinbase Wallet, and Rainbow.
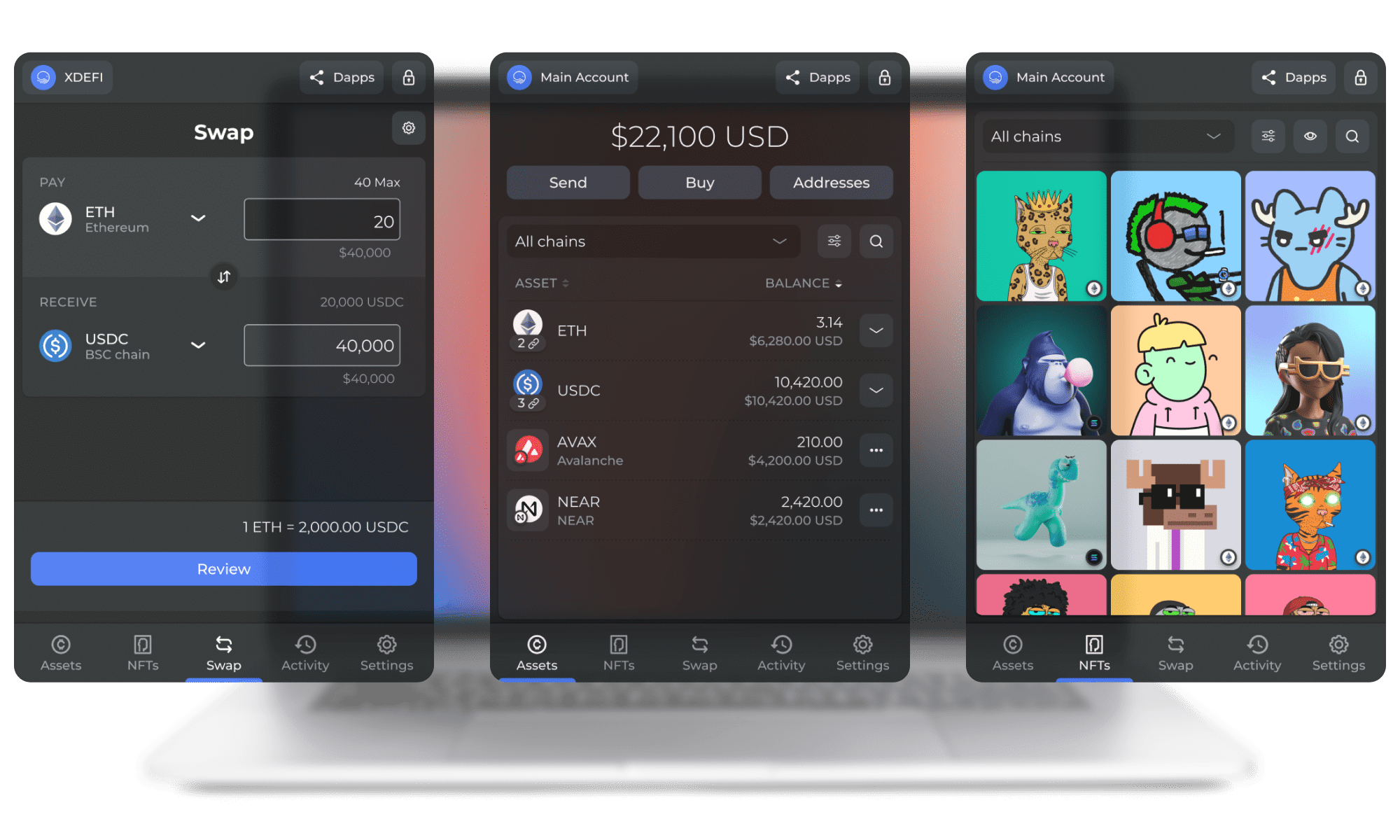
- Crypto Tax Platforms: Crypto tax platforms are applications designed to help both individuals and businesses calculate taxes on their cryptocurrency transactions. With the complex nature of crypto transactions and evolving regulations, these tools have become quite valuable for traders and investors. A great example of a crypto tax platform is Koinly.
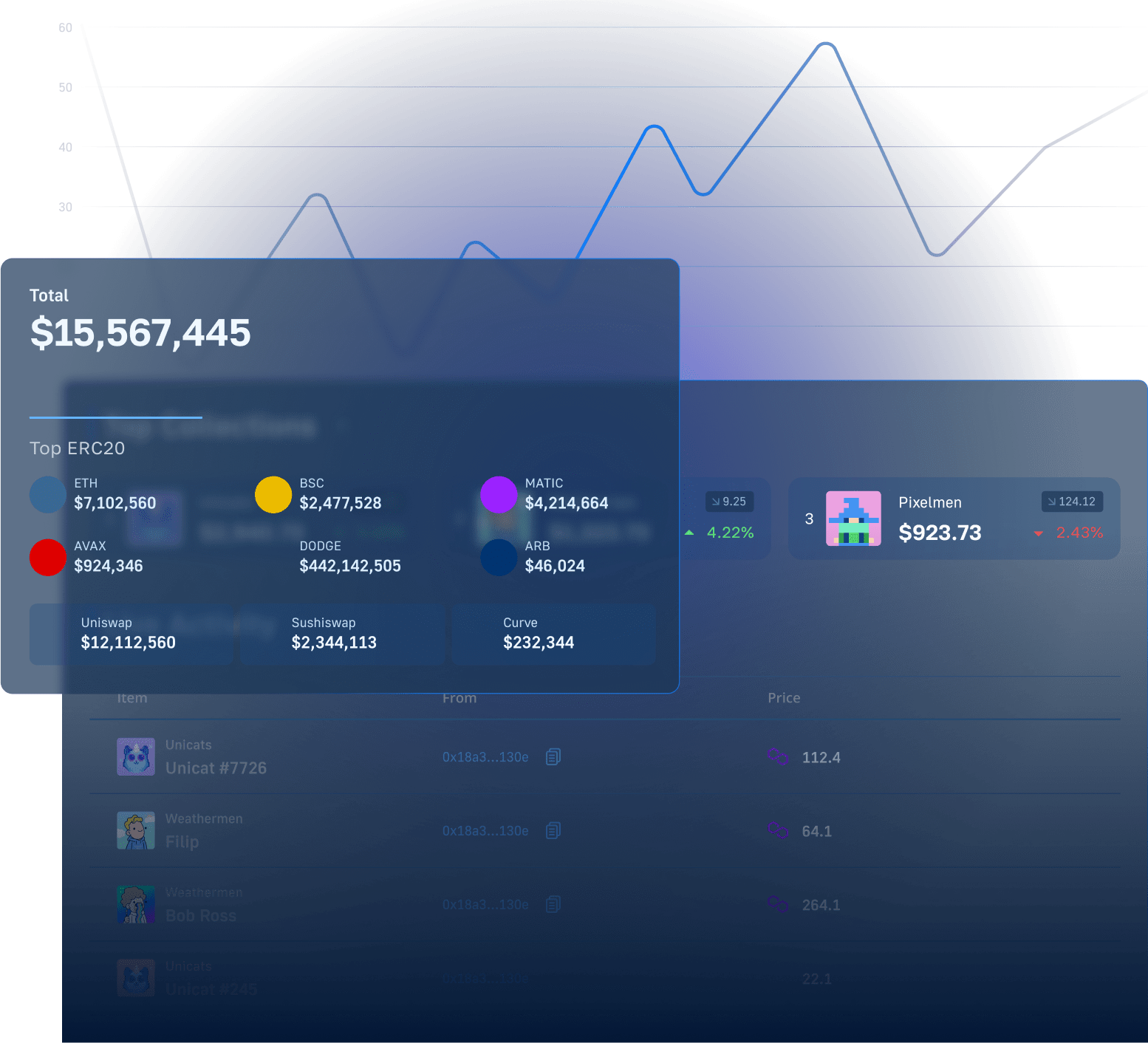
- Data and Analytics Tools: Data and analytics tools are platforms giving users in-depth insight into the performance of cryptocurrencies. These tools can provide a wide range of features, including market analytics, portfolio tracking, blockchain analytics, and more. A prominent example of an industry-leading data and analytics tool is Moralis Money!
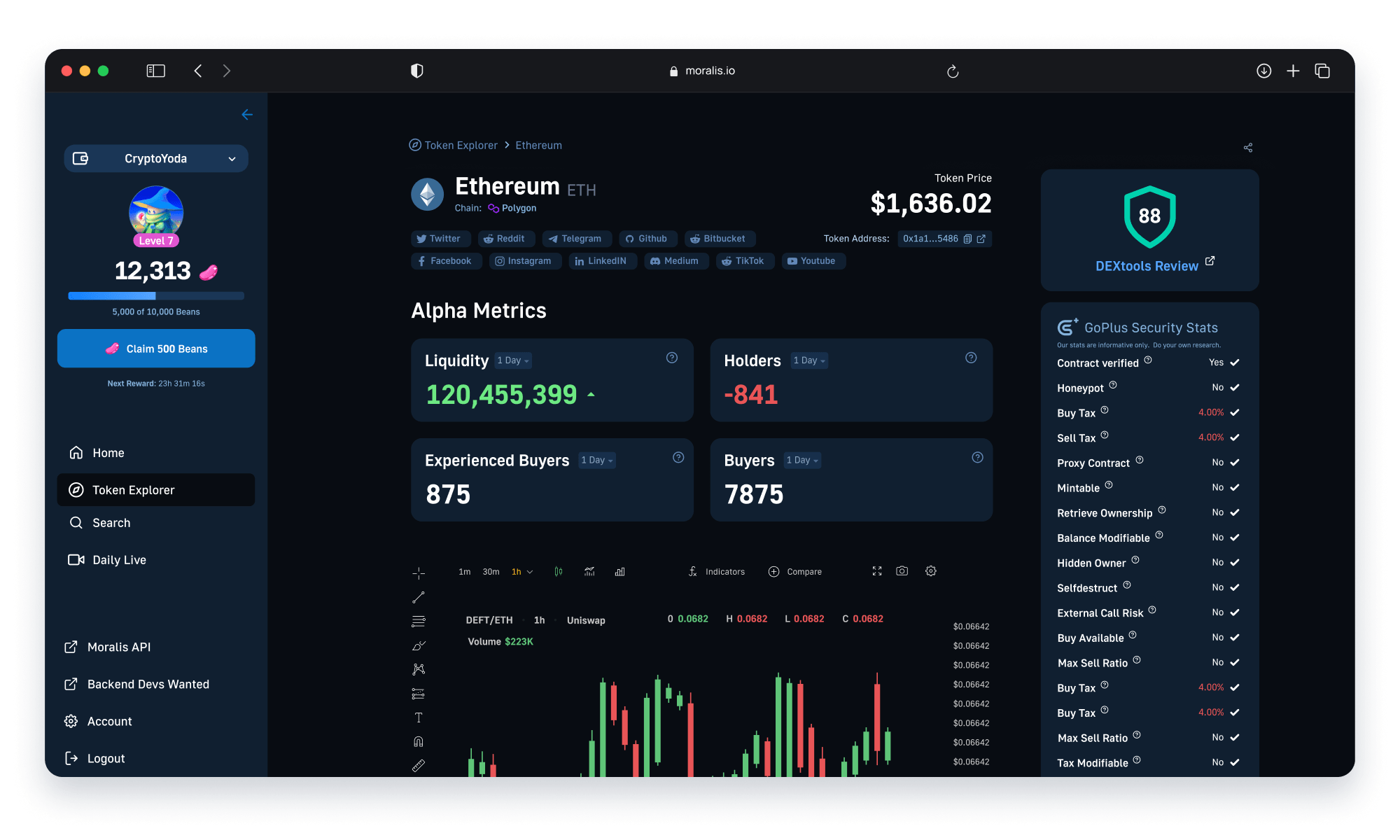
The use cases above are only a few prominent examples, and you’ll likely find the Wallet Net Worth endpoint useful in most of your development endeavors!
Beyond Getting the Wallet Net Worth – Exploring the Wallet API Further
Moralis’ Wallet API is the industry’s premier tool for anyone looking to build cryptocurrency wallets or integrate wallet functionality into dapps. This interface supports more than 300 million addresses across the most prominent blockchains, including Ethereum, Polygon, Solana, and many others!
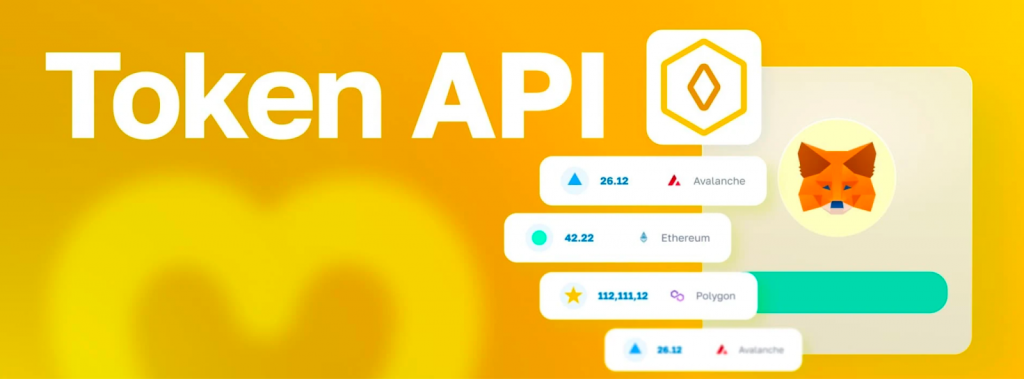
With the Wallet API, you can effortlessly query blockchain data. This includes wallet balances, profile data, transactions, and even the net worth of a wallet! Plus, you can do so with only single lines of code. As such, when using the Wallet API, you can build everything from crypto wallets to tax tools without breaking a sweat.
To highlight the capabilities of this premier API, let’s explore some additional endpoints below:
Token Balances with Prices:
The Token Balances with Prices endpoint lets you fetch a wallet’s ERC20 token balances along with metadata, prices, etc. Moreover, you can do so with only a single API call. Here’s an example of what it might look like:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch('https://deep-index.moralis.io/api/v2.2/wallets/0xcB1C1FdE09f811B294172696404e88E658659905/tokens?chain=eth', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
In return for running the code above, you’ll get a response that looks something like this:
{ //... "result": [ { "token_address": "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", "symbol": "USDC", "name": "USD Coin", "logo": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48.png", "thumbnail": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_thumb.png", "decimals": 6, "balance": "4553447", "possible_spam": false, "verified_contract": true, "balance_formatted": "4.553447", "usd_price": 1.001818879776249, "usd_price_24hr_percent_change": 0.1818879776249283, "usd_price_24hr_usd_change": 0.0018221880998897314, "usd_value": 4.561729172660522, "usd_value_24hr_usd_change": 0.008297236936878599, "native_token": false, "portfolio_percentage": 100 }, //... ] }
To learn more about how this works, check out our most recent guide exploring the ultimate crypto portfolio tracker endpoint!
getWalletNFTs()
: With thegetWalletNFTs()
endpoint, you can seamlessly get the NFT balance of any wallet. You’ll find an example of what it might look like here:
import Moralis from 'moralis'; try { await Moralis.start({ apiKey: "YOUR_API_KEY" }); const response = await Moralis.EvmApi.nft.getWalletNFTs({ "chain": "0x1", "format": "decimal", "mediaItems": false, "address": "0xff3879b8a363aed92a6eaba8f61f1a96a9ec3c1e" }); console.log(response.raw); } catch (e) { console.error(e); }
In return, you’ll get an array of all NFTs the wallet in question holds. It will look something like this:
{ //... { "amount": "1", "token_id": "5021", "token_address": "0xfff54e6fe44fd47c8814c4b1d62c924c54364ad3", "contract_type": "ERC721", "owner_of": "0xff3879b8a363aed92a6eaba8f61f1a96a9ec3c1e", "last_metadata_sync": null, "last_token_uri_sync": "2024-02-27T13:25:49.783Z", "metadata": null, "block_number": "14647390", "block_number_minted": "14647390", "name": "Youtopia", "symbol": "Youtopia", "token_hash": "d4719eaf84eabcf443065b0a463f5886", "token_uri": "http://api.youtopia-official.xyz/ipfs/5021", "minter_address": "0x13f11fd2c7c7be94674651386370d02b7aac9653", "verified_collection": false, "possible_spam": true, "collection_logo": "https://i.seadn.io/gae/e3uNxyaqT0FfnhcF9SuMqCZd3pdF36wgcnpRJ0VDjLOP71g_LwrFRgLweNNCMvsMqR5ZZ4dh5Wble12PBzvncmpLbtmdVdjr5zMy8w?w=500&auto=format", "collection_banner_image": "https://i.seadn.io/gae/n9j18OhplkvqP5SOtuYDwpUVkJSwF6WkIV6vZMWjcm0D5qCpbd12cAaVlfZS8-3gjxjYsnjL_tIlVIsjXz28KejPB3D19Jc_MZ9Z?w=500&auto=format" }, //… ] }
getWalletTransactions()
: You can use thegetWalletTranscactions()
endpoint to query the native transaction history of any wallet. Check out the example below to see how it works:
import Moralis from 'moralis'; try { await Moralis.start({ apiKey: "YOUR_API_KEY" }); const response = await Moralis.EvmApi.transaction.getWalletTransactions({ "chain": "0x1", "address": "0x1f9090aaE28b8a3dCeaDf281B0F12828e676c326" }); console.log(response.raw); } catch (e) { console.error(e); }
In return for calling the endpoint above, you’ll get a list of the wallet’s native transactions. Here’s an example of what it might look like:
{ //... "result": [ { "hash": "0xc2dae4e9323e4b4daae845d56bb2b0b1963b65b4cebd232a30c600147bd0d394", "nonce": "516871", "transaction_index": "343", "from_address": "0x1f9090aae28b8a3dceadf281b0f12828e676c326", "from_address_label": "rsync-builder", "to_address": "0x388c818ca8b9251b393131c08a736a67ccb19297", "to_address_label": "Lido: Execution Layer Rewards Vault", "value": "126626152737137840", "gas": "22111", "gas_price": "46932278594", "input": "0x", "receipt_cumulative_gas_used": "29863868", "receipt_gas_used": "22111", "receipt_contract_address": null, "receipt_root": null, "receipt_status": "1", "block_timestamp": "2024-02-27T13:29:59.000Z", "block_number": "19319196", "block_hash": "0xaf38cea22f06e3d31e24dadc8cc27daa65f09c68713ce8343489536dde6ea22d", "transfer_index": [ 19319196, 343 ] }, //... ] }
If you’d like to explore all the endpoints of this API, please check out the official Wallet API documentation page!
Summary: How to Get the Net Worth of Any ERC20 Wallet via API
From a conventional perspective, it has always been a tedious and time-consuming task to get the net worth of any ERC20 crypto wallet via API. Furthermore, it has typically required you to manually collect data across multiple blockchains, resulting in separate API calls for ERC20 token balances, native balances, prices, etc. The outcome of this is a complex workflow that takes up a lot of development resources and time. However, you can now circumvent this entire process using Moralis’ Wallet API and the Wallet Net Worth endpoint!
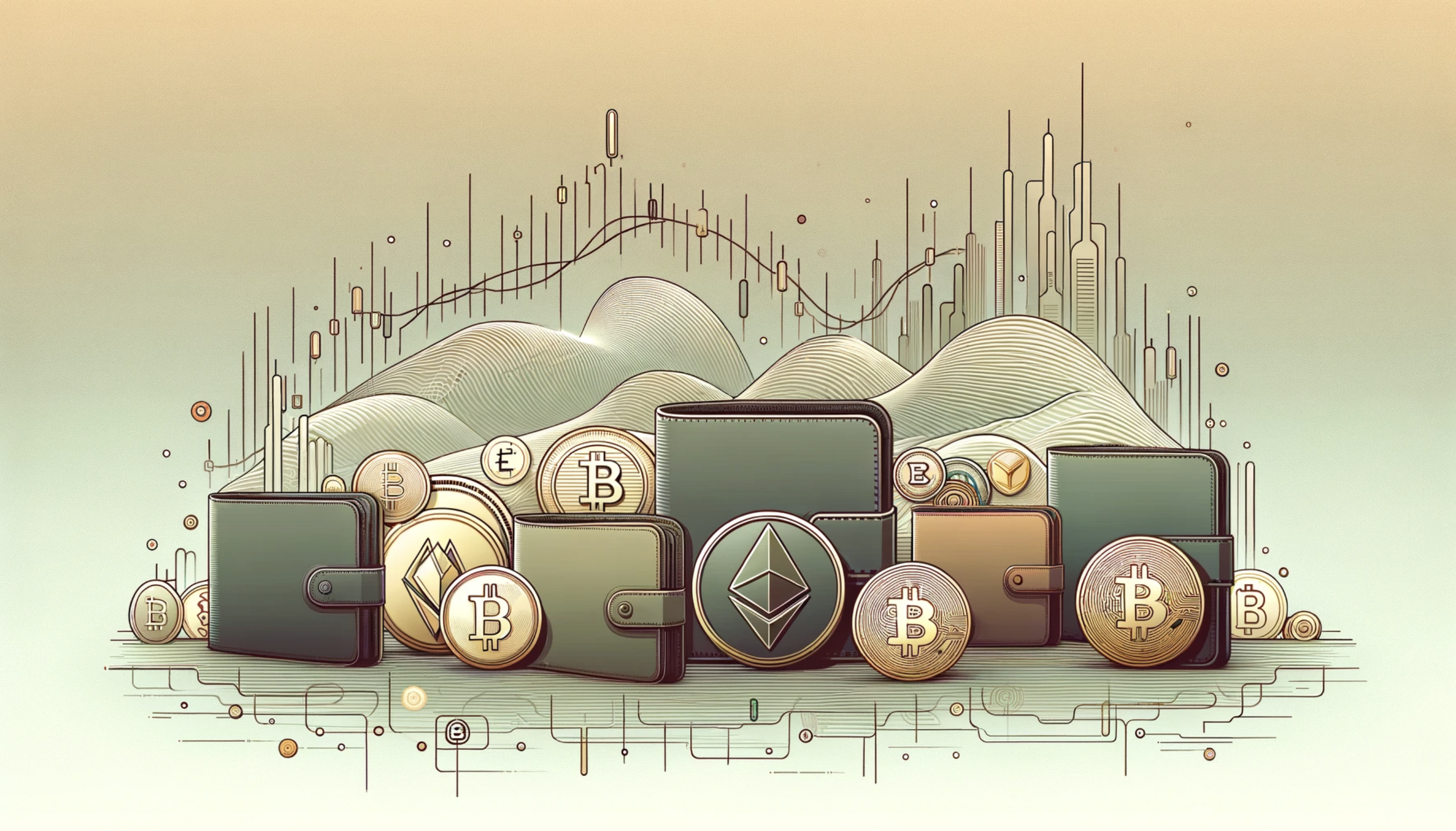
With Moralis’ Wallet Net Worth endpoint, you only need a single API call to get ERC20 wallet net worth data across the biggest blockchains. As such, when working with Moralis, it has never been easier to build cryptocurrency wallets, portfolio trackers, or any other platforms that integrate the net worth of an ERC20 wallet!
Did you like this tutorial showing how to get the net worth of an ERC20 wallet using Moralis’ API? If so, consider checking out more content here on the blog. For instance, read one of our most recent articles that shows you how to query the Ethereum blockchain! Also, if you wish to use the Wallet API, don’t forget to sign up with Moralis. You can create an account for free, and you’ll gain instantaneous access to our premier development tools!