Are you looking for the easiest way to get crypto portfolio data? With Moralis’ Token API and our crypto portfolio tracker endpoint, you can seamlessly get any wallet’s token balances – along with metadata, prices, and much more – with a single API call. For a little sneak peek of how this works, check out the example below:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch('https://deep-index.moralis.io/api/v2.2/wallets/0xcB1C1FdE09f811B294172696404e88E658659905/tokens?chain=eth', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
All you have to do is install the required dependencies, replace YOUR_API_KEY
with your Moralis API key, and configure the address parameter to fit your query. Then, once you run the code above, you’ll get a response that looks like this:
{ //... "result": [ { "token_address": "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", "symbol": "USDC", "name": "USD Coin", "logo": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48.png", "thumbnail": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_thumb.png", "decimals": 6, "balance": "4553447", "possible_spam": false, "verified_contract": true, "balance_formatted": "4.553447", "usd_price": 1.001818879776249, "usd_price_24hr_percent_change": 0.1818879776249283, "usd_price_24hr_usd_change": 0.0018221880998897314, "usd_value": 4.561729172660522, "usd_value_24hr_usd_change": 0.008297236936878599, "native_token": false, "portfolio_percentage": 100 }, //... ] }
This response not only contains the token balances of the wallet in question but also features metadata, prices, price changes over time, and much more. As such, when using this endpoint, you get everything you need to build a crypto portfolio view!
To learn more about this, check out the official token balances with prices documentation page or join us in this article!
Also, if you wish to start using our APIs yourself, don’t forget to sign up with Moralis. You can create an account free of charge, and you’ll gain immediate access to all our industry-leading development tools!
Leading Web3 APIs?
Overview
Whether you’re building a portfolio tracker, cryptocurrency wallet, crypto tax tool, or other Web3 platforms, you typically need easy access to portfolio data. This allows you to seamlessly display your users’ token balances – along with prices, metadata, and more. Yet, sourcing the data you need can be a complex task. And given the many options for querying portfolio data, how do you find the best alternative?
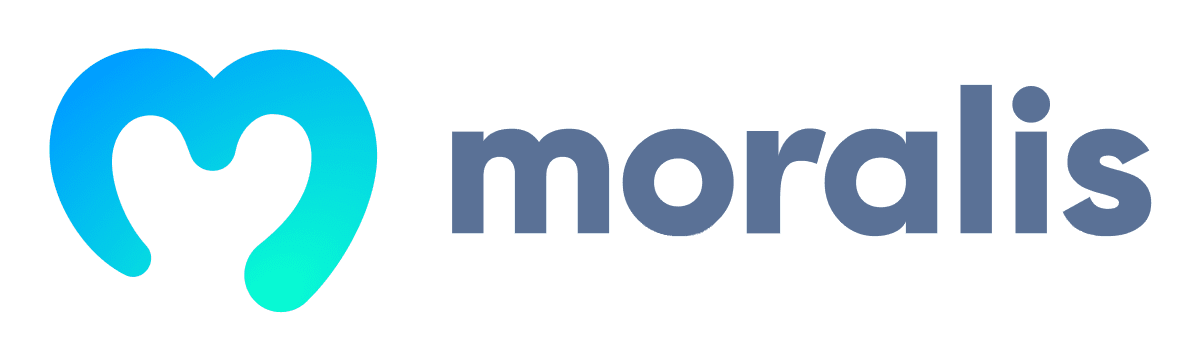
Throughout this article, we’ll introduce you to the easiest way to query portfolio data – Moralis’ Token API. With the Token API and our crypto portfolio tracker endpoint, you can seamlessly query a wallet’s token balances – including metadata, prices, and more – with single lines of code. Consequently, it doesn’t matter if you already have an existing dapp or want to build a new one from scratch; this guide is tailored for you. Let’s go!
Introducing Moralis’ Token API – Easiest Way to Get Portfolio Data
Moralis’ Token API is the industry’s most comprehensive interface for ERC-20 token data. The Token API supports every single token across ten+ EVM-compatible chains. This includes stablecoins like USDC, meme coins like Shiba Inu, and everything in between!
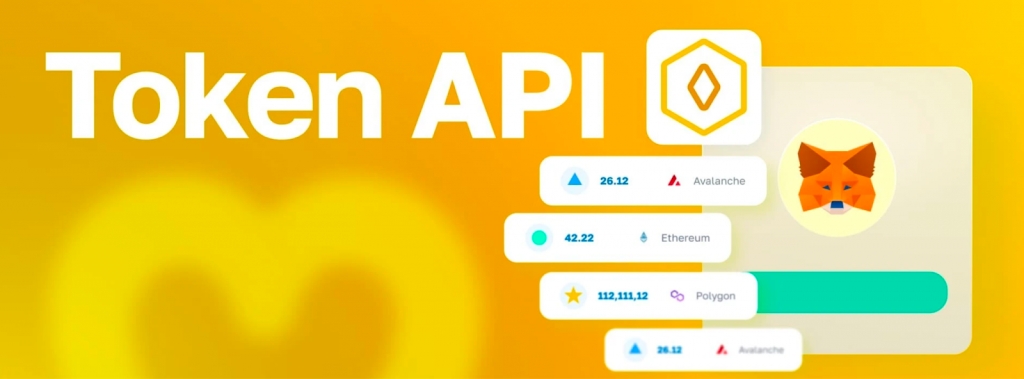
With only single lines of code, you can effortlessly use the Token API to query token balances, metadata, transactions, and much more. Consequently, when working with this top-tier Web3 API, you can build everything from cryptocurrency wallets to portfolio trackers without breaking a sweat.
So, why should you leverage Moralis and the Token API when building Web3 projects?
- Comprehensive: Moralis enriches all of its API responses with transaction decodings, market data, address labels, metadata, and more from multiple sources. Doing so allows us to deliver the industry’s most comprehensive Web3 APIs.
- Simple and Cross-Chain Compatibility: Request and response structures are universal across all APIs and endpoints, providing you and your team with a seamless developer experience. What’s more, our APIs are cross-chain compatible, supporting the biggest blockchains, including Ethereum, Polygon, Solana, and many others.
- Trusted: Hundreds of thousands of Web3 developers and large enterprise customers, including MetaMask, Delta, and Polygon, already use and trust Moralis’ Web3 APIs.

Also, if you wish to check out all our industry-leading development tools, please check out our Web3 API page!
With an overview of Moralis and the Token API, let’s now explore the ultimate crypto portfolio tracker endpoint!
Ultimate Crypto Portfolio Tracker Endpoint
As you now know, Moralis offers the industry’s most comprehensive Web3 APIs. We enrich all our API responses with metadata, address labels, transaction decodings, and much more. Consequently, with the Token Balance endpoint, you get everything you need to build a crypto portfolio view in one single API response.
Let’s explore what this looks like in practice!
With Moralis’ Token Balance endpoint, you can seamlessly query the token balances – along with metadata, prices, and much more – of any wallet with just one single call:
GET https://deep-index.moralis.io/api/v2.2/wallets/0xd8da6bf26964af9d7eed9e03e53415d37aa96045/tokens?chain=eth
In return for calling the endpoint above, you’ll get a response that looks like this:
{ //... "result": [ { "token_address": "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", "symbol": "USDC", "name": "USD Coin", "logo": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48.png", "thumbnail": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_thumb.png", "decimals": 6, "balance": "4553447", "possible_spam": false, "verified_contract": true, "balance_formatted": "4.553447", "usd_price": 1.001818879776249, "usd_price_24hr_percent_change": 0.1818879776249283, "usd_price_24hr_usd_change": 0.0018221880998897314, "usd_value": 4.561729172660522, "usd_value_24hr_usd_change": 0.008297236936878599, "native_token": false, "portfolio_percentage": 100 }, //... ] }
The response above is fully enriched with token metadata, prices, price changes over time, and much more for each token. From here, you can simply integrate this data into your projects and build your own crypto portfolio view in a heartbeat!
But why does the crypto portfolio tracker endpoint matter? And how does it differ from the competition?
Why the Crypto Portfolio Tracker Endpoint Matters
The crypto portfolio tracker endpoint clearly highlights the benefits of Moralis’ comprehensive APIs and demonstrates the richness of our API responses. With this single endpoint, you’re able to fetch the balances, along with metadata, prices, and more, from any address. As such, when using Moralis, you don’t have to bother with multiple endpoints and providers!
Let’s summarize the main benefits of the crypto portfolio tracker endpoint below:
- Streamlined Developer Experience: Fetch all the portfolio data you need in a single API call. This results in fewer providers, less programming logic, and an overall more streamlined developer experience.
- Improved User Experience: With access to real-time price data, price changes over time, and much more, you can give your users a comprehensive view of their portfolio and optimize the user experience.
- Save Time: Eliminate the need for multiple API requests where you need to fetch balances, metadata, and prices separately. This allows you to minimize the development time and effort needed to build a portfolio view. In return, you get more time to focus on other parts of the development process so you can bring more value to your users.
How Does the Endpoint Compare to the Competition?
With Moralis’ Token Balance endpoint, you get a wallet’s balances – along with metadata, prices, and more for each token – with one single endpoint. To highlight the value of this, let’s compare Moralis to our two main competitors: Alchemy and QuickNode.
We recently conducted a test using the Web3 APIs from Moralis, Alchemy, and QuickNode to create a portfolio view of Vitalik Buterin’s ERC-20 tokens. In doing so, we compared the number of calls required to complete this task for each provider. Check out the results of our test below:
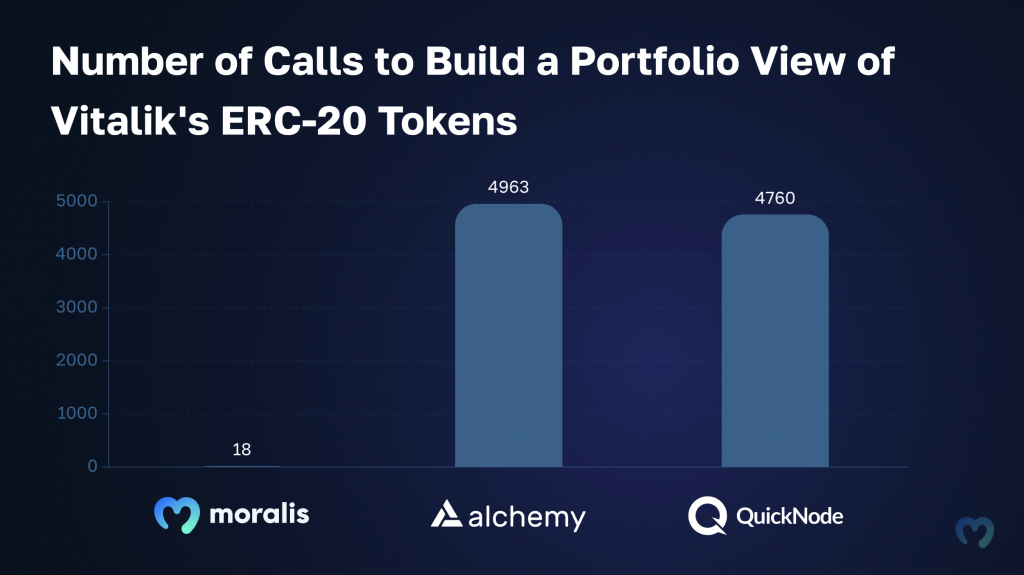
As the chart above demonstrates, you only need 18 calls to fetch all the data when working with Moralis. In comparison, the same task demands thousands and thousands of calls when using Alchemy and QuickNode. So, why are we seeing such drastically different results between Moralis and the other providers?
When using Alchemy and QuickNode, you must first use one endpoint to fetch the wallet’s balance. From there, you need to query another endpoint to get the metadata of each token. Lastly, you must use a third-party API provider like CoinGecko or CoinMarketCap to fetch price data. In comparison, with Moralis, you get all this information from one single endpoint!
If you want a more comprehensive breakdown of our test, check out our guide comparing the industry’s leading Web3 API providers!
3-Step Tutorial: How to Call Moralis’ Crypto Portfolio Tracker Endpoint
With an overview of Moralis’ Token API and our crypto portfolio tracker endpoint, we’ll now show you how to call the Token Balance endpoint in three steps:
- Step 1: Get a Moralis API Key
- Step 2: Write a Script Calling the Token Balance Endpoint
- Step 3: Run the Code
However, before we can get going with the first step, we need to deal with a couple of prerequisites!
Prerequisites
If you haven’t already, you need to install and set up the following before continuing:
- Node.js v14+
- Npm/Yarn
Step 1: Get a Moralis API Key
Create a Moralis account by clicking on the button at the top right:
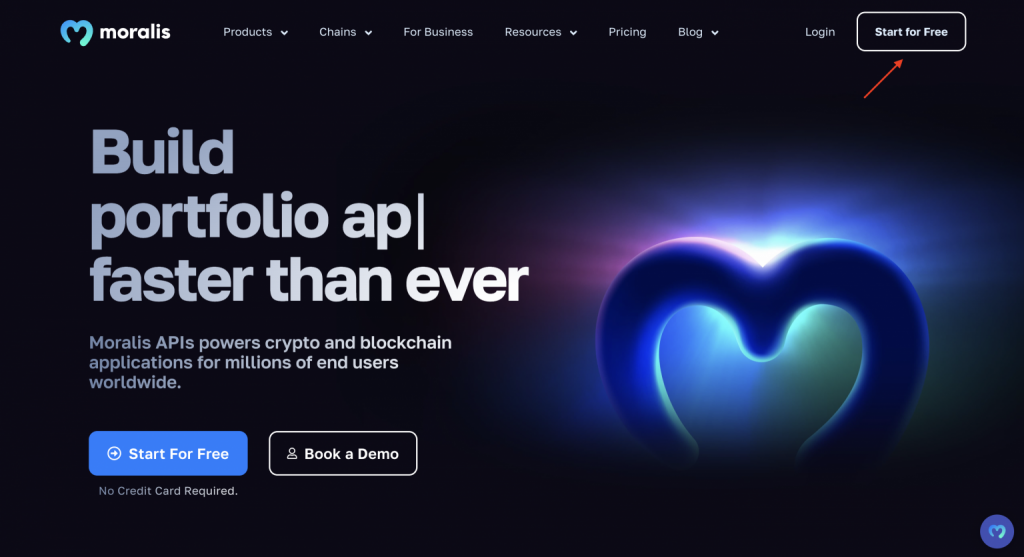
Next, go to the ”Settings” tab, scroll down to the ”API Keys” section, and copy your key:
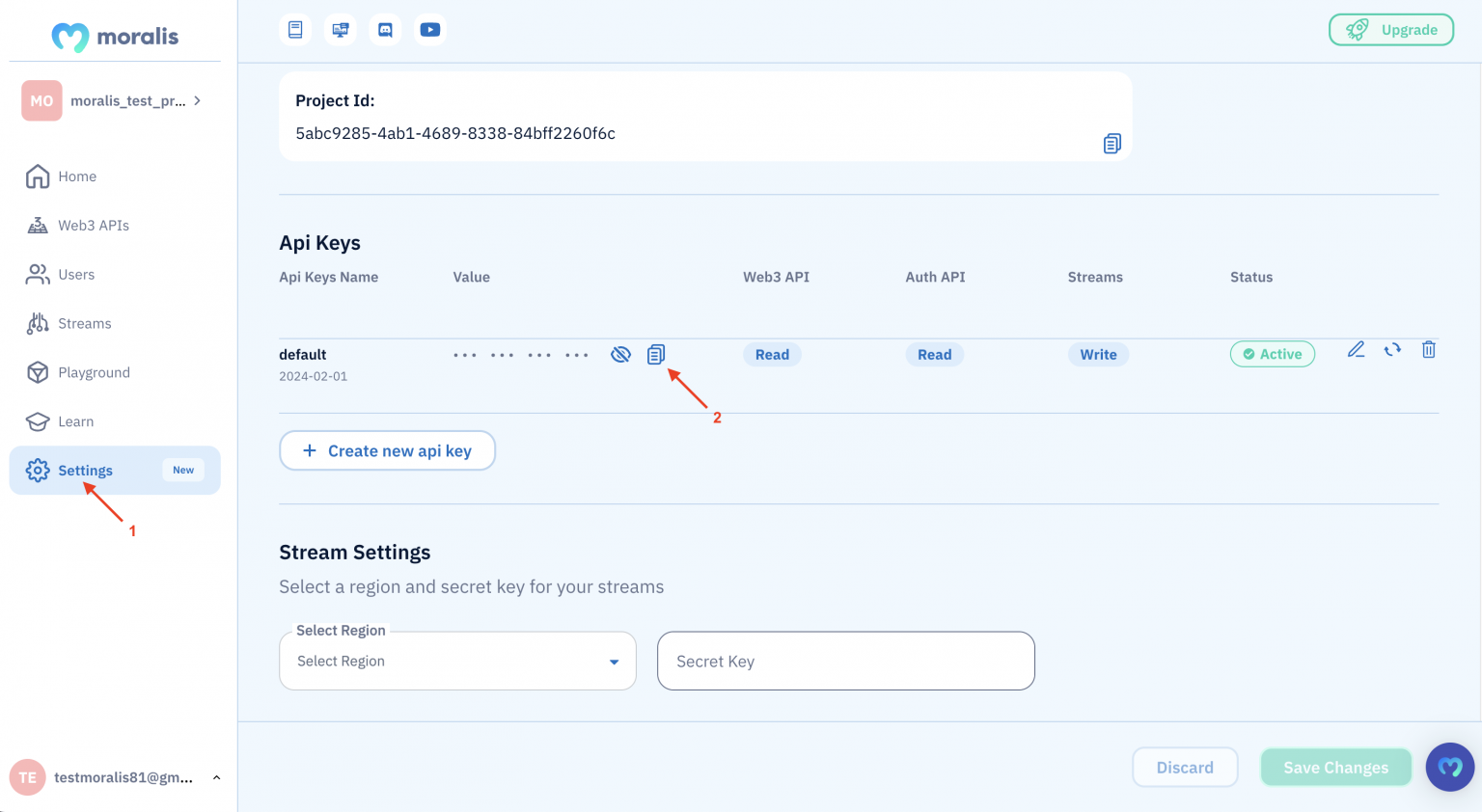
Keep it for now, as you’ll need it in the next section.
Step 2: Write a Script Calling the Token Balance Endpoint
Set up a new folder in your preferred IDE and run the following terminal command to initialize a new project:
npm init
Next, install the required dependencies by running the following command in the terminal:
npm install node-fetch --save npm install moralis @moralisweb3/common-evm-utils
From here, open your ”package.json” file and add ”type”: ”module”
to the list:
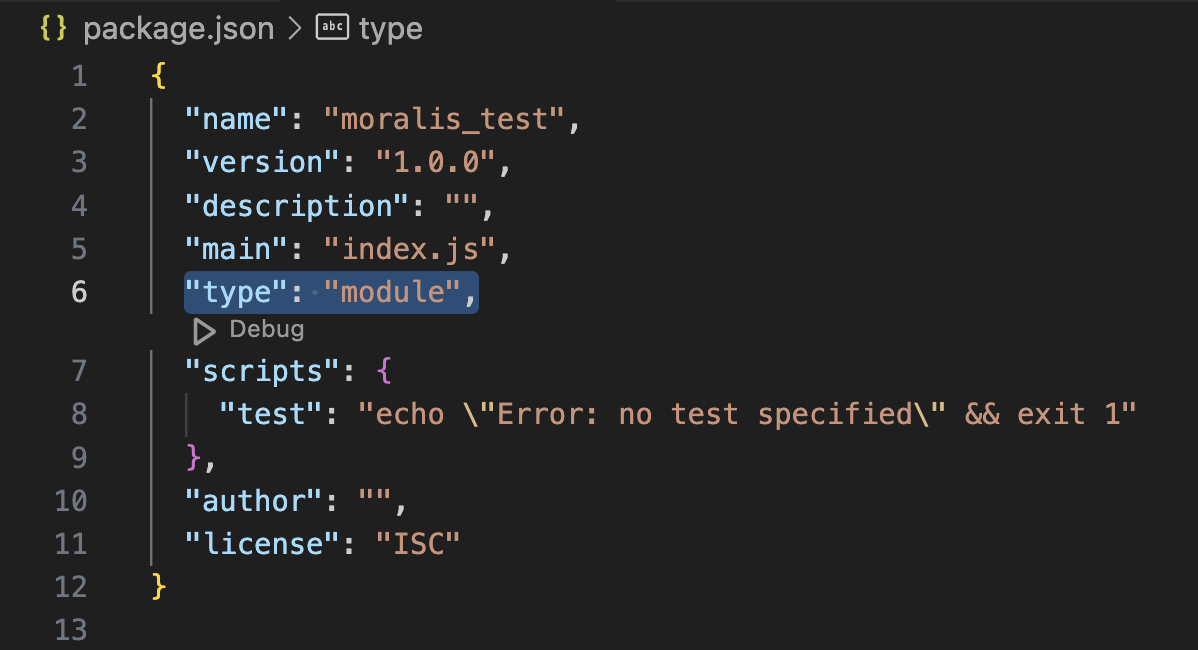
You can then create a new ”index.js” file and add the following code:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch('https://deep-index.moralis.io/api/v2.2/wallets/0xcB1C1FdE09f811B294172696404e88E658659905/tokens?chain=eth', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
Next, add your Moralis API key by replacing YOUR_API_KEY
and configure the address and chain parameters to fit your query:
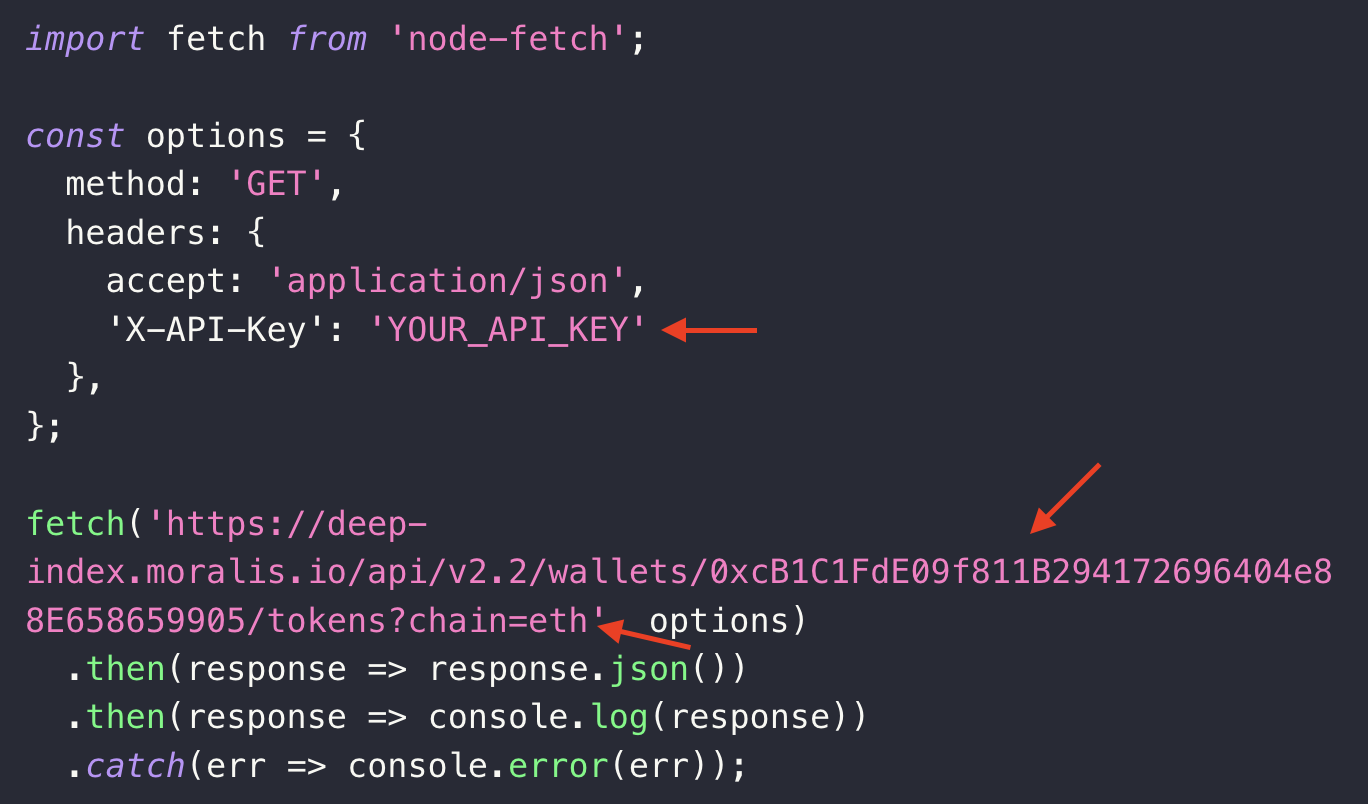
That’s it: you’re now ready to run the code!
Step 3: Run the Code
Open a new terminal, cd into the root folder of your project, and run this command:
node index.js
Once you run the code, you’ll get a response that looks similar to this:
{ //... "result": [ { "token_address": "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", "symbol": "USDC", "name": "USD Coin", "logo": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48.png", "thumbnail": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_thumb.png", "decimals": 6, "balance": "4553447", "possible_spam": false, "verified_contract": true, "balance_formatted": "4.553447", "usd_price": 1.001818879776249, "usd_price_24hr_percent_change": 0.1818879776249283, "usd_price_24hr_usd_change": 0.0018221880998897314, "usd_value": 4.561729172660522, "usd_value_24hr_usd_change": 0.008297236936878599, "native_token": false, "portfolio_percentage": 100 }, //... ] }
That’s it; it doesn’t have to be more challenging to get the token balances, along with prices, metadata, and more, from any wallet when using Moralis’ Token API!
Beyond the Crypto Portfolio Tracker Endpoint – Get Transfers, Metadata, and More with the Token API
In addition to the crypto portfolio tracker endpoint, there’s a lot of other data you can query using the Token API. As such, let’s explore three additional endpoints you’ll likely find helpful in your development endeavors:
getWalletTokenTransfers()
– Get the transfer history of any wallet:
const response = await Moralis.EvmApi.token.getWalletTokenTransfers({ "chain": "0x1", "address": "0x1f9090aaE28b8a3dCeaDf281B0F12828e676c326" });
getTokenPrices()
– Query the price of any token:
const response = await Moralis.EvmApi.token.getTokenPrice({ "chain": "0x1", "address": "0x7d1afa7b718fb893db30a3abc0cfc608aacfebb0" });
getTokenMetadata()
– Fetch the metadata of any token:
const response = await Moralis.EvmApi.token.getTokenMetadata({ "chain": "0x1", "addresses": [ "0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48" ] });
To learn more about the various endpoints of Moralis’ Token API, check out the official Token API documentation page!
What Can You Build with the Token API? – Use Cases for the Crypto Portfolio Tracker Endpoint
There are many instances when you, as a developer, need to integrate crypto portfolio data into your projects. As such, let’s explore three prominent use cases for Moralis’ Token API and the crypto portfolio tracker endpoint!
- Crypto Tax Tools: Crypto tax tools are specialized software designed to help individuals and businesses calculate and report cryptocurrency-related taxes. Given the challenges associated with the taxation of cryptocurrencies – including their volatility, the complexity of tracking gains and losses over multiple transactions, and the evolving regulatory landscape – these platforms play an essential role in the space.
A great example of a crypto tax tool is Koinly. Koinly imports all user transactions, finds the prices at the time of the trades and calculates taxes based on this data. So, when building a platform like this, you need access to token balances, metadata, and prices.
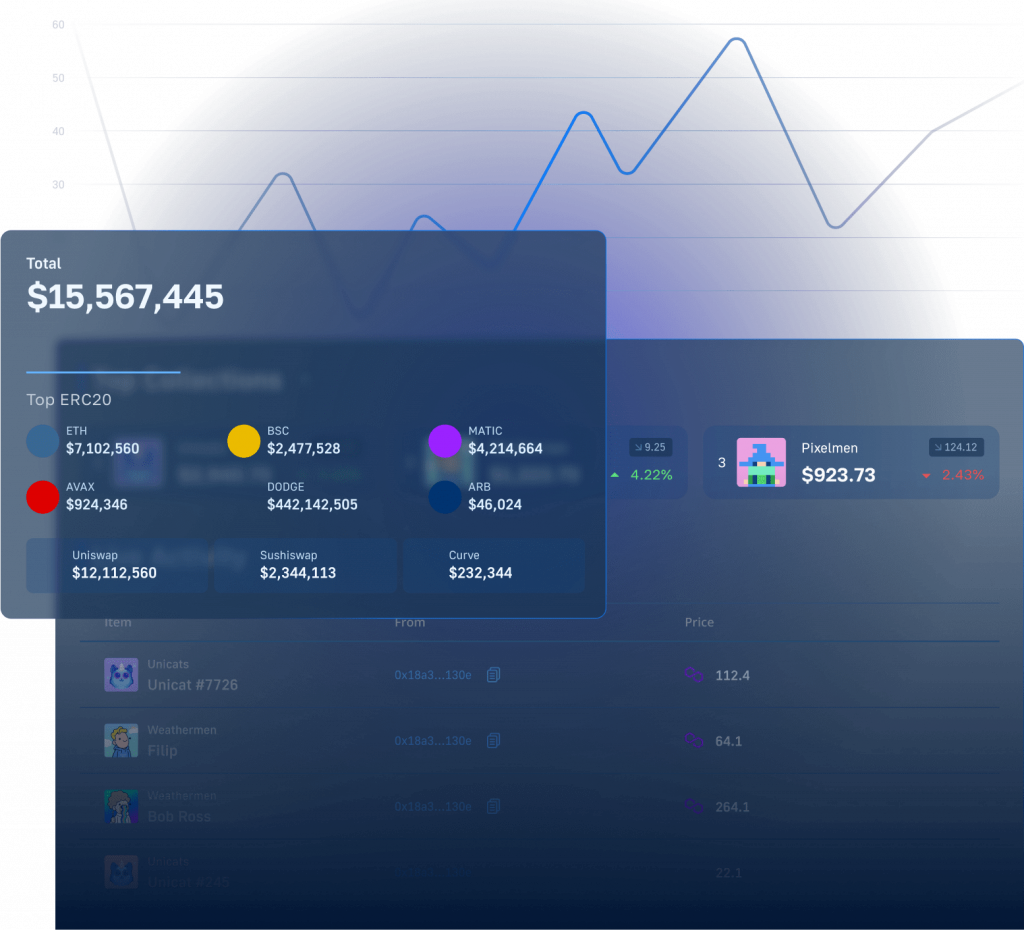
- Portfolio Trackers: Portfolio trackers are apps that allow users to monitor the performance of their digital assets. Furthermore, they give users an organized way to monitor and manage their fungible tokens and NFTs in one place.
An example of a prominent portfolio tracker is Delta – an industry-leading platform for tracking digital assets, including cryptocurrencies, NFTs, and stocks. Delta uses Moralis’ Why Did It Move API to inform users not only when the value of tokens changes but also why the price increases or decreases.
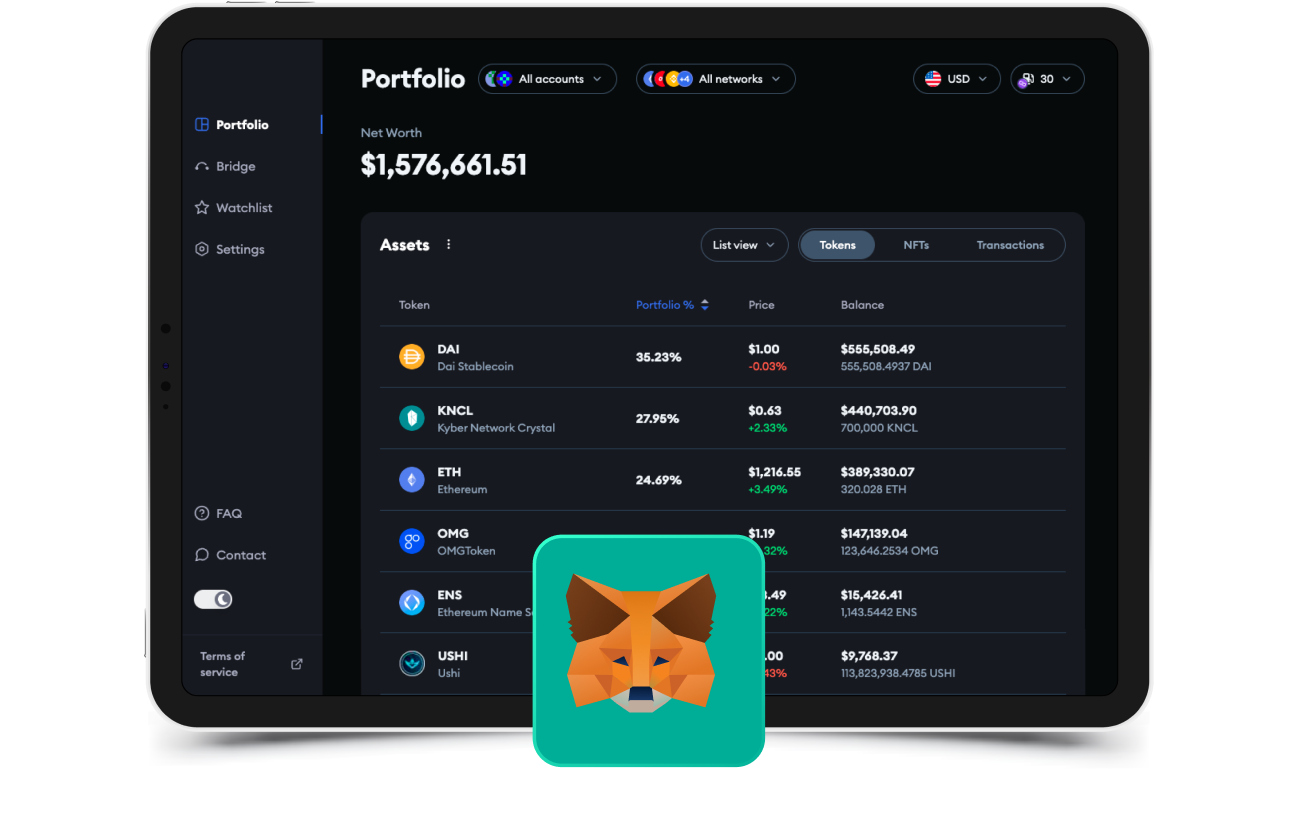
- Cryptocurrency Wallets: Cryptocurrency wallets are platforms that allow users to store their digital assets. The best wallets also provide tools for buying, selling, and swapping cryptocurrencies. As such, they give users the ability to fully manage their fungible and non-fungible tokens.
A great example of a cryptocurrency wallet provider leveraging Moralis’ Web3 APIs is MetaMask. MetaMask is the industry’s leading cryptocurrency wallet, with millions and millions of active users across the globe.
Summary: Crypto Portfolio Tracker Endpoint – Get Portfolio Data with a Single API Call
With Moralis’ Token API and our crypto portfolio tracker endpoint, you can seamlessly fetch token balances, along with metadata and prices, with just one single call. Just check out the Token Balance endpoint in action down below:
import fetch from 'node-fetch'; const options = { method: 'GET', headers: { accept: 'application/json', 'X-API-Key': 'YOUR_API_KEY' }, }; fetch('https://deep-index.moralis.io/api/v2.2/wallets/0xcB1C1FdE09f811B294172696404e88E658659905/tokens?chain=eth', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
Simply install the required dependencies, replace YOUR_API_KEY
, configure the address parameter to fit your query, and run the code. In return, you’ll get a response that looks something like this:
{ //... "result": [ { "token_address": "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", "symbol": "USDC", "name": "USD Coin", "logo": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48.png", "thumbnail": "https://cdn.moralis.io/eth/0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_thumb.png", "decimals": 6, "balance": "4553447", "possible_spam": false, "verified_contract": true, "balance_formatted": "4.553447", "usd_price": 1.001818879776249, "usd_price_24hr_percent_change": 0.1818879776249283, "usd_price_24hr_usd_change": 0.0018221880998897314, "usd_value": 4.561729172660522, "usd_value_24hr_usd_change": 0.008297236936878599, "native_token": false, "portfolio_percentage": 100 }, //... ] }
The fully enriched response above contains the token balances, along with metadata, prices, price changes over time, and much more for each token. As such, with this single endpoint, you can seamlessly build a crypto portfolio tracker view without breaking a sweat!
If you liked this crypto portfolio tracker endpoint article, consider checking out more content here on the blog. For instance, read our most recent guide on how to query the Ethereum blockchain or learn how to build on OP Mainnet.
Also, don’t forget to sign up with Moralis. You can create an account for free, and you’ll gain instant access to all our industry-leading Web3 APIs!